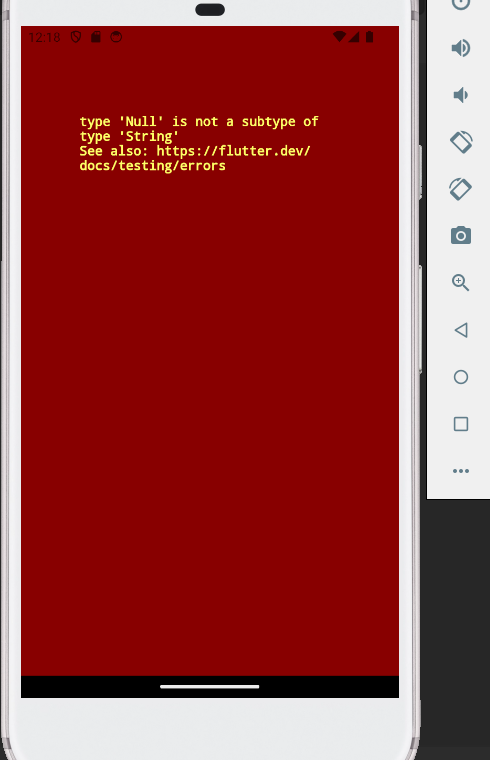
The “type ‘Null’ is not a subtype of type ‘String’” error typically occurs when trying to access a property of an object that is null. In Flutter, this often happens when using the null-aware operator (??
) or when accessing properties of widget parameters that could be null.
Causes of Null Values: Null values can arise from various sources in a Flutter application:
- Widget Parameters: When passing data to a widget using constructor parameters, the data might be null if not provided or initialized properly.
- Asynchronous Operations: Data fetched asynchronously from APIs or databases might not be available immediately, leading to null values until the operation completes.
- Optional Data: Some properties of objects may be optional and could be null under certain conditions.
Solutions:
- Null-aware Operators:
- Use the null-aware operator (
??
) to provide default values for nullable variables. - Example:
String name = nullableName ?? 'Unknown';
- Use the null-aware operator (
- Null Checking:
- Check if a variable is null before accessing its properties.
- Example:
if (data != null) {
// Access properties of data
} else {
// Handle the case where data is null
}
Default Values:
- Provide default values when accessing properties that might be null.
Example
String regNumber = widget.registrationData['regnumber'] ?? '';
Asynchronous Operations:
- Use
FutureBuilder
to handle asynchronous operations and display loading indicators or error messages while waiting for data. - Check for null data before accessing it in the
builder
function.
In my case:
@override
void initState() {
super.initState();
fetchCouncils(); // Fetch councils when the widget initializes
regNumberController.text = widget.registrationData['regnumber'] ?? '';
_dropdownValue = widget.registrationData['regcouncil'] ?? 'Select Council'; // Initialize as null
_selectedYear = widget.registrationData['regyear'] ?? null;
}
After changing
@override
void initState() {
super.initState();
fetchCouncils(); // Fetch councils when the widget initializes
if (widget.registrationData != null) {
regNumberController.text = widget.registrationData['regnumber'] ?? '';
_dropdownValue =
widget.registrationData['regcouncil'] ?? 'Select Council';
_selectedYear = widget.registrationData['regyear'] ??
yearsList.first; // Use a default value if regyear is null
} else {
// Handle the case where widget.registrationData is null
}
}