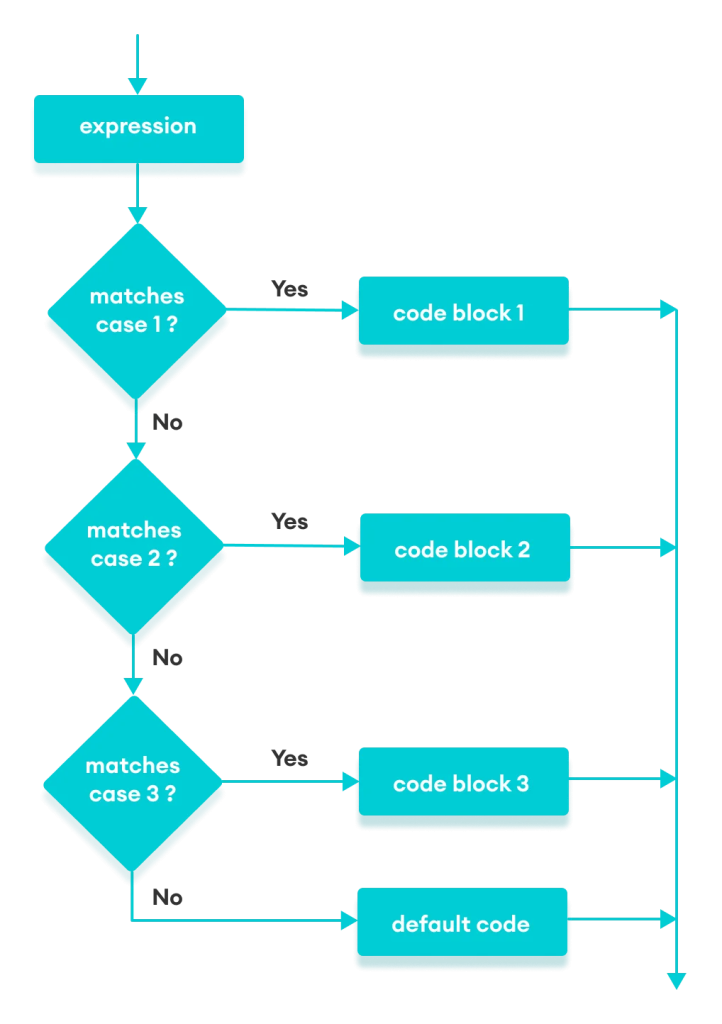
JavaScript is a versatile programming language that offers developers a multitude of tools and techniques to solve complex problems efficiently. One such feature is the switch statement, a control flow mechanism that allows for concise and readable code when handling multiple conditional cases. In this blog post, we will explore the JavaScript switch statement, its syntax, and provide practical examples to demonstrate its usage.
Syntax:
The switch statement in JavaScript follows a specific syntax:
switch (expression) { case value1: // Code to execute when the expression matches value1 break; case value2: // Code to execute when the expression matches value2 break; case value3: // Code to execute when the expression matches value3 break; // … default: // Code to execute when no case matches the expression }The switch statement begins with the keyword switch
, followed by an expression enclosed in parentheses. The expression is evaluated, and its value is compared against the values in each case
statement. When a match is found, the corresponding block of code executes until a break
statement is encountered. If no match is found, the code within the default
block executes (if present).
A simple example where we use a switch statement to handle different days of the week:
const day = new Date().getDay(); let dayName; switch (day) { case 0: dayName = “Sunday”; break; case 1: dayName = “Monday”; break; case 2: dayName = “Tuesday”; break; case 3: dayName = “Wednesday”; break; case 4: dayName = “Thursday”; break; case 5: dayName = “Friday”; break; case 6: dayName = “Saturday”; break; default: dayName = “Invalid day”; } console.log(`Today is ${dayName}.`);In this example, the day
variable holds the numeric value of the current day of the week. The switch statement matches this value against the cases, assigns the corresponding dayName
, and prints it using console.log()
. If the value does not match any of the cases, the default case sets dayName
to “Invalid day.”