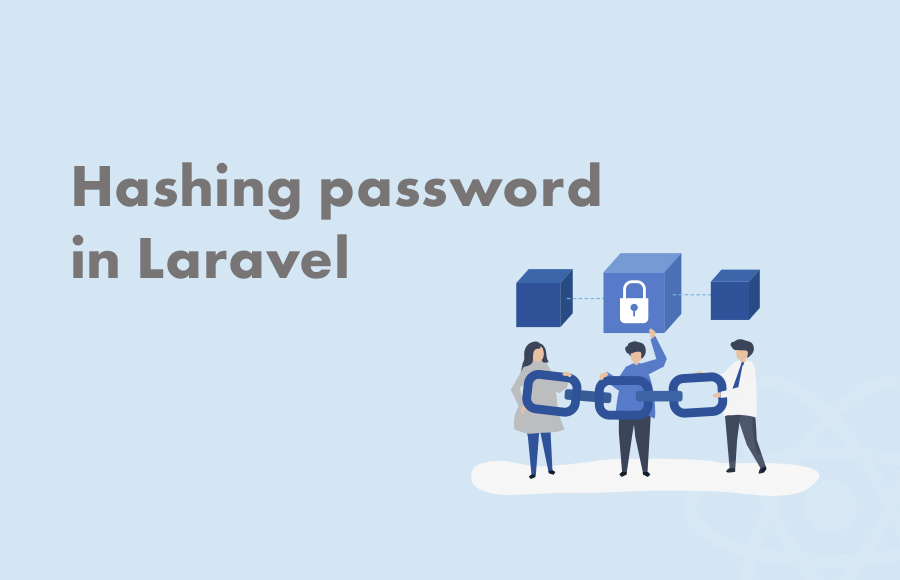
In any web application, ensuring the security of user passwords is paramount. Laravel, being a robust PHP framework, offers convenient methods for hashing passwords securely using the Bcrypt algorithm.
, let’s briefly understand the concept of password hashing. Hashing is a process of converting plain text passwords into a hashed representation, which is a one-way encryption method. This means that once a password is hashed, it cannot be reversed to retrieve the original password. Therefore, even if the hashed passwords are compromised, the original passwords remain secure.
Using Bcrypt in Laravel
Laravel provides a convenient Hash facade that allows you to hash passwords using the Bcrypt algorithm effortlessly. Here’s how you can use it in your Laravel application.
$password = Hash::make('yourpassword');
This single line of code generates a hashed representation of the provided password. You can use this method directly in your controllers, models, or wherever necessary within your application.
Practical Example
Let’s consider a scenario where you need to hash a password submitted by a user through a registration form. Here’s how you can implement it.
$password = Input::get('password'); // Retrieve password from form input
$hashedPassword = Hash::make($password); // Hash the password
In this example, $hashedPassword will contain the securely hashed representation of the user’s password. You would typically perform this operation when registering a new user or updating an existing user’s password.
Laravel simplifies the process of hashing passwords using the Bcrypt algorithm, ensuring that user authentication remains secure and robust. By incorporating password hashing into your application, you can safeguard user credentials effectively and mitigate the risk of security breaches.
By following the guidelines outlined in this guide, you can implement secure password hashing practices in your Laravel applications seamlessly, contributing to a safer user experience and enhanced security measures.
Now that you understand how to create hashed passwords in Laravel, you can confidently incorporate this essential security feature into your web applications. Stay proactive in safeguarding user data and prioritize security in every aspect of your development process.