CRUD operations are fundamental to web applications. Here’s a step-by-step guide to setting up and performing CRUD operations in a Laravel application.
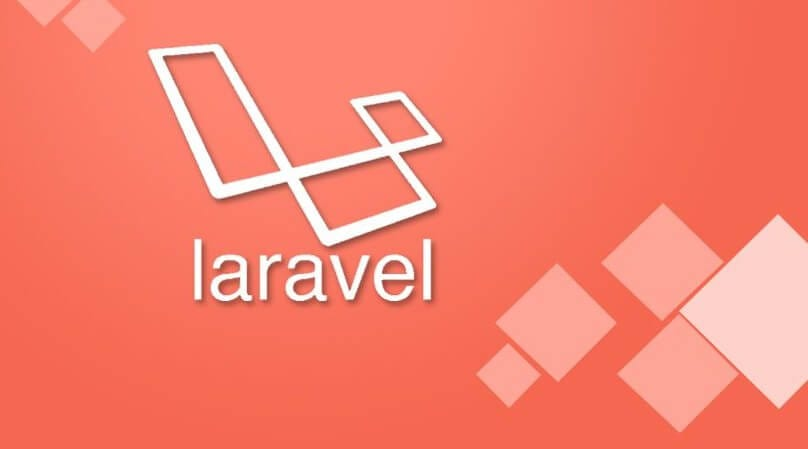
Step 1: Setting Up Laravel
- Install Laravel:
Use Composer to create a new Laravel project. Open your terminal and run:
composer create-project --prefer-dist laravel/laravel crud-example
- Configure the Database:
Update your.env
file with your database details:
DB_CONNECTION=mysql
DB_HOST=127.0.0.1
DB_PORT=3306
DB_DATABASE=your_database
DB_USERNAME=your_username
DB_PASSWORD=your_password
- Run Migrations:
Execute the migrations to create the necessary database tables:
php artisan migrate
Step 2: Creating a Model and Migration
- Generate a Model and Migration:
php artisan make:model Item -m
This command creates an Item
model and a migration file.
- Define the Database Schema:
Open the generated migration file indatabase/migrations/
and define your table schema:
public function up()
{
Schema::create('items', function (Blueprint $table) {
$table->id();
$table->string('name');
$table->text('description')->nullable();
$table->timestamps();
});
}
- Run the Migration:
php artisan migrate
Step 3: Setting Up Routes
Open routes/web.php
and add the routes for CRUD operations:
use App\Http\Controllers\ItemController;
Route::resource('items', ItemController::class);
Step 4: Creating a Controller
Generate a resource controller to handle CRUD operations:
php artisan make:controller ItemController --resource
Step 5: Implementing CRUD Methods in the Controller
Open app/Http/Controllers/ItemController.php
and implement the CRUD methods:
namespace App\Http\Controllers;
use App\Models\Item;
use Illuminate\Http\Request;
class ItemController extends Controller
{
public function index()
{
$items = Item::all();
return view('items.index', compact('items'));
}
public function create()
{
return view('items.create');
}
public function store(Request $request)
{
$request->validate([
'name' => 'required',
'description' => 'nullable',
]);
Item::create($request->all());
return redirect()->route('items.index')->with('success', 'Item created successfully.');
}
public function show(Item $item)
{
return view('items.show', compact('item'));
}
public function edit(Item $item)
{
return view('items.edit', compact('item'));
}
public function update(Request $request, Item $item)
{
$request->validate([
'name' => 'required',
'description' => 'nullable',
]);
$item->update($request->all());
return redirect()->route('items.index')->with('success', 'Item updated successfully.');
}
public function destroy(Item $item)
{
$item->delete();
return redirect()->route('items.index')->with('success', 'Item deleted successfully.');
}
}
Step 6: Creating Views
Create Blade template files for each method inside resources/views/items/
:
- Index (
index.blade.php
):
<!DOCTYPE html>
<html>
<head>
<title>Items List</title>
</head>
<body>
<h1>Items List</h1>
<a href="{{ route('items.create') }}">Create New Item</a>
<ul>
@foreach ($items as $item)
<li>
<a href="{{ route('items.show', $item->id) }}">{{ $item->name }}</a>
<a href="{{ route('items.edit', $item->id) }}">Edit</a>
<form action="{{ route('items.destroy', $item->id) }}" method="POST">
@csrf
@method('DELETE')
<button type="submit">Delete</button>
</form>
</li>
@endforeach
</ul>
</body>
</html>
- Create (
create.blade.php
):
<!DOCTYPE html>
<html>
<head>
<title>Create Item</title>
</head>
<body>
<h1>Create Item</h1>
<form action="{{ route('items.store') }}" method="POST">
@csrf
<label for="name">Name:</label>
<input type="text" name="name" id="name" required>
<label for="description">Description:</label>
<textarea name="description" id="description"></textarea>
<button type="submit">Save</button>
</form>
</body>
</html>
- Show (
show.blade.php
):
<!DOCTYPE html>
<html>
<head>
<title>Item Details</title>
</head>
<body>
<h1>{{ $item->name }}</h1>
<p>{{ $item->description }}</p>
<a href="{{ route('items.index') }}">Back to List</a>
</body>
</html>
- Edit (
edit.blade.php
):
<!DOCTYPE html>
<html>
<head>
<title>Edit Item</title>
</head>
<body>
<h1>Edit Item</h1>
<form action="{{ route('items.update', $item->id) }}" method="POST">
@csrf
@method('PUT')
<label for="name">Name:</label>
<input type="text" name="name" id="name" value="{{ $item->name }}" required>
<label for="description">Description:</label>
<textarea name="description" id="description">{{ $item->description }}</textarea>
<button type="submit">Update</button>
</form>
</body>
</html>
Final Steps
- Start the Development Server:
php artisan serve
- Access Your Application:
Open your browser and navigate tohttp://localhost:8000/items
to start using your CRUD application.
With these steps, you have set up a basic CRUD application using Laravel. Customize and enhance it further according to your requirements.