Routing is an essential concept in web development, determining how an application responds to various user requests. In Laravel, routing plays a pivotal role, allowing you to define the URLs your application should respond to and specify the controller methods that handle these requests. This tutorial provides a comprehensive overview of the basics of routing in Laravel, covering key concepts, functions, and examples to help you effectively define and manage routes.
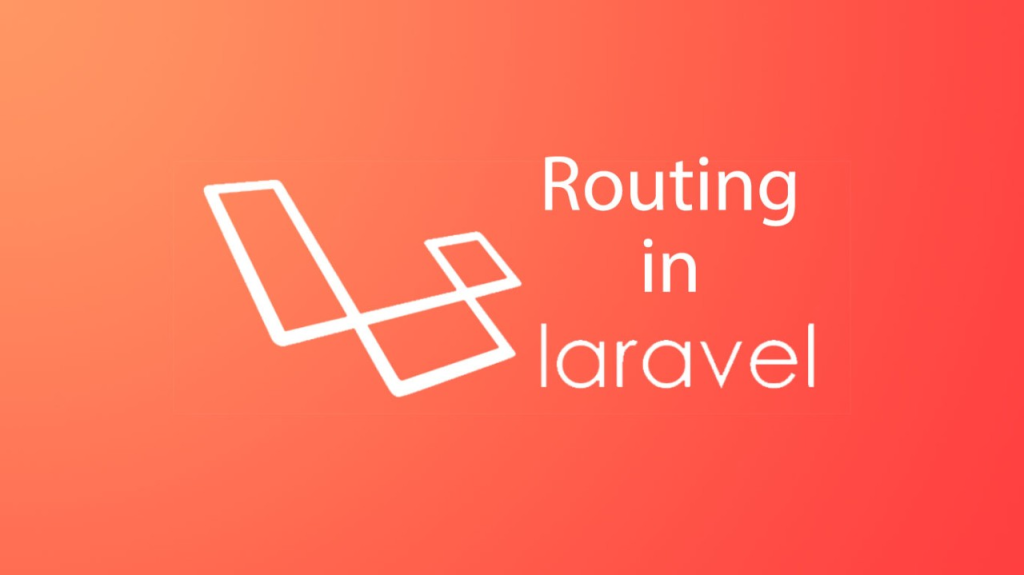
Introduction to Routing in Laravel
In Laravel, routing is the mechanism that maps HTTP requests to specific controllers or closures. Routes are defined in route files located in the routes
directory of your Laravel application.
Key Concepts in Laravel Routing
(a). Laravel supports various HTTP methods such as GET, POST, PUT, DELETE, PATCH, and OPTIONS.
- Route Definition:
(a). Routes in Laravel are defined in route files using a simple and expressive syntax.
(b). Common route files includeweb.php
,api.php
,console.php
, andchannels.php
. - Route Methods:
(b). Each method corresponds to a type of HTTP request that the route will respond to.
- Route Parameters:
(a). Routes can accept parameters to capture dynamic values from the URL.
(b). Parameters can be required or optional and can have constraints. - Named Routes:
(a). Named routes allow you to assign a name to a specific route, making it easier to generate URLs or redirects.
- Route Groups:
(a). Route groups allow you to apply common middleware, namespaces, or prefixes to a group of routes.
Basic Route Definition
Routes are typically defined in the routes/web.php
file for web routes and routes/api.php
for API routes.
Example of a Basic Route
// routes/web.php
use Illuminate\Support\Facades\Route;
Route::get('/', function () {
return view('welcome');
});
This route responds to a GET request to the root URL (/
) and returns the welcome
view.
Defining Routes with Controller Methods
Instead of defining a closure directly in the route, you can specify a controller method to handle the request.
Example of a Route with a Controller
// routes/web.php
use App\Http\Controllers\HomeController;
Route::get('/home', [HomeController::class, 'index']);
This route responds to a GET request to /home
and invokes the index
method of the HomeController
.
Route Parameters
Routes can capture dynamic values from the URL using parameters.
Example of a Route with Parameters
// routes/web.php
Route::get('/user/{id}', function ($id) {
return 'User '.$id;
});
This route responds to a GET request to /user/{id}
and captures the id
parameter from the URL.
Optional Parameters
Parameters can be made optional by appending a ?
to the parameter name.
Example of a Route with Optional Parameters
// routes/web.php
Route::get('/user/{name?}', function ($name = 'Guest') {
return 'Hello '.$name;
});
This route responds to a GET request to /user/{name?}
and defaults the name
parameter to ‘Guest’ if not provided.
Named Routes
Named routes provide a way to generate URLs or redirects for specific routes by name.
Example of a Named Route
// routes/web.php
Route::get('/user/profile', [UserProfileController::class, 'show'])->name('profile');
// Generating a URL
$url = route('profile');
// Generating a Redirect
return redirect()->route('profile');
Route Groups
Route groups allow you to apply common attributes such as middleware, namespaces, or prefixes to a group of routes.
Example of a Route Group
Code:
// routes/web.php
Route::middleware(['auth'])->group(function () {
Route::get('/dashboard', function () {
// Uses 'auth' middleware
});
Route::get('/account', function () {
// Uses 'auth' middleware
});
});
This route group applies the auth
middleware to all routes within the group.
Middleware in Routes
Middleware can be applied to individual routes or groups of routes to filter HTTP requests entering your application.
Example of Middleware in Routes
Code:
// routes/web.php
Route::get('/profile', function () {
// Profile logic
})->middleware('auth');
This route applies the auth
middleware to the /profile
route.
Route Resource
Laravel provides a convenient way to create CRUD (Create, Read, Update, Delete) routes using Route::resource
.
Example of Route Resource
Code:
// routes/web.php
use App\Http\Controllers\PostController;
Route::resource('posts', PostController::class);
This single line generates all the necessary routes for CRUD operations on the Post
resource.
Route Model Binding
Route model binding allows you to automatically inject the model instance corresponding to the route parameter.
Example of Route Model Binding
Code:
// routes/web.php
use App\Models\User;
Route::get('/user/{user}', function (User $user) {
return $user;
});
This route automatically injects the User
model instance corresponding to the {user}
parameter.