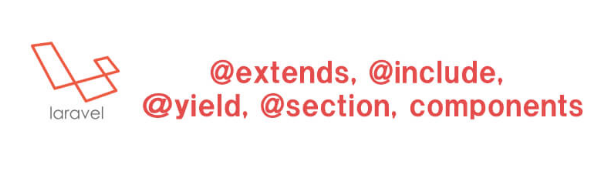
In Laravel, the concepts of layouts, yield, extend, and sections are used to create reusable templates and organize the structure of your application’s views. These features make it easier to manage the common elements shared across multiple pages while allowing flexibility to customize specific sections of each page.
Creating a Layout:
First, you’ll create a layout file that defines the common structure and components of your application. This file typically resides in the views/layouts
directory. Let’s call it app.blade.php
.
Here’s an example of a basic layout file:
<!DOCTYPE html>
<html>
<head>
<title>@yield('title')</title>
</head>
<body>
<header>
<!-- Common header content goes here -->
</header>
<main>
@yield('content')
</main>
<footer>
<!-- Common footer content goes here -->
</footer>
</body>
</html>
In this layout, you can see that we have defined sections for the title, content, header, and footer. The @yield
directives indicate the areas where content from other views can be injected.
Extending the Layout:
To utilize the layout, you need to create views that extend it. These views will inherit the structure and components defined in the layout while allowing you to customize specific sections. Let’s create a view called home.blade.php
as an example:
@extends('layouts.app')
@section('title', 'Home')
@section('content')
<h1>Welcome to our website!</h1>
<p>This is the content of the home page.</p>
@endsection
In this view, we use the @extends
directive to specify that we want to extend the app.blade.php
layout. We also use the @section
directive to define the content for the title
and content
sections.
Rendering the View:
To render the final view, you can use the view
helper function in your routes or controllers. Here’s an example of how you can render the home.blade.php
view:
Route::get('/', function () {
return view('home');
});
When the home.blade.php
view is rendered, Laravel will automatically pull in the content defined in the @section
directives and inject them into the corresponding @yield
directives in the layout. This allows the final output to include the common layout structure along with the customized content specific to the home page. By using layouts, yield, extend, and sections, you can create a consistent structure for your views while providing the flexibility to customize individual sections. This approach simplifies the management of shared elements and improves the maintainability of your Laravel application’s views.