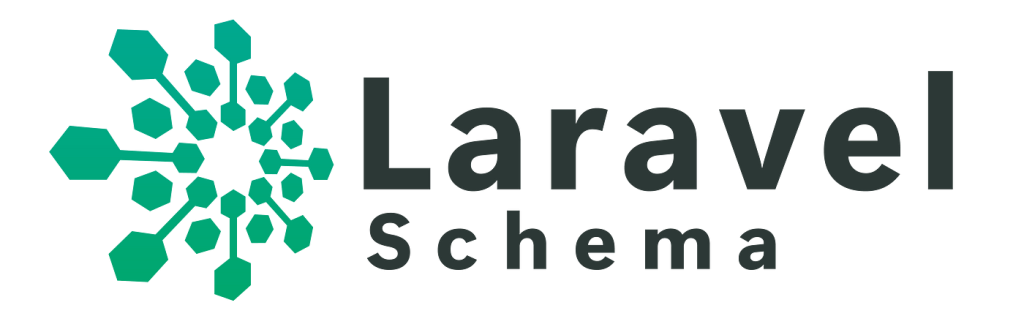
Managing database schema efficiently is crucial for any application, and Laravel’s Schema Builder provides a powerful set of methods and concepts to achieve this goal seamlessly within your Laravel application. These methods not only aid in creating and modifying tables but also in ensuring consistency across different environments and version-controlling your database schema changes. Let’s delve into each of these methods and concepts in detail:
- Creating Tables:
Schema::create('table', function($table) { })
: This method is used to define a new table. Within the closure, you can specify the table’s columns, indexes, and other options. This approach helps in structuring the database schema effectively.
- Specifying a Connection:
Schema::connection('foo')->create('table', function($table) { })
: This method allows creating a table using a specific database connection. It’s particularly useful when dealing with multiple databases within your Laravel application.
- Renaming Tables:
Schema::rename($from, $to)
: Renaming an existing table can be achieved using this method. It helps in maintaining a clean and organized database structure by updating table names as required.
- Dropping Tables:
Schema::drop('table')
: Permanently deletes a table along with its data. Use this method with caution as it’s irreversible.Schema::dropIfExists('table')
: This method safely deletes the table only if it exists, thus avoiding errors during execution.
- Checking for Table Existence:
Schema::hasTable('table')
: It checks if a table exists in the database and returns true or false accordingly. This is useful for conditional logic within migrations or other database operations.
- Checking for Column Existence:
Schema::hasColumn('table', 'column')
: Determines whether a column exists in a table and returns true or false. It helps in handling scenarios where you need to validate or alter existing columns.
- Updating Tables:
Schema::table('table', function($table) { })
: This method modifies an existing table’s structure. You can add, modify, or remove columns, indexes, and constraints within the closure.
- Renaming Columns:
$table->renameColumn('from', 'to')
: Renames an existing column within a table. It’s useful for refining the database schema without losing data or relationships.
- Dropping Columns:
$table->dropColumn(string|array)
: Removes one or more columns from a table. This can be handy when certain columns are no longer needed or need to be replaced.
- Setting Storage Engine:
$table->engine = 'InnoDB'
: This sets the storage engine for the table, particularly useful in MySQL databases. It allows you to specify the engine that best fits your application’s requirements.
11. Modifying Columns:
$table->string('name')->after('email')
: Modifies column attributes, such as data type and default values.
12. Indexes:
- Laravel provides methods like
primary
,unique
, andindex
to create different types of indexes, improving query performance.
13. Dropping Indexes:
- Methods like
dropPrimary
,dropUnique
, anddropIndex
remove indexes from a table.
14. Foreign Keys:
$table->foreign('user_id')->references('id')->on('users')
: Defines foreign key constraints, establishing relationships between tables.
15. Dropping Foreign Keys:
$table->dropForeign(array('user_id'))
: Removes foreign key constraints.
16. Column Types:
- Laravel offers various column types for different data types, including integers, floats, strings, text, date and time, binary, boolean, and enums.
17. Modifiers:
- You can apply modifiers like
nullable
,default
,unsigned
, and others to columns.
18. Timestamps:
- Methods like
timestamps
andnullableTimestamps
automatically addcreated_at
andupdated_at
columns to track timestamps.
19. Soft Deletes:
softDeletes
adds adeleted_at
column for soft deletes.
20. Remember Token:
rememberToken
adds aremember_token
column for authentication.
21. Morphs:
morphs('parent')
creates columns for polymorphic relationships.
22. increments
and bigIncrements
:
$table->increments('id')
and$table->bigIncrements('id')
create auto-incrementing integer columns.
23. Numbers:
- Laravel provides integer and numeric column types with different storage and precision characteristics.
24. String and Text:
- Column types allow you to store character data of varying lengths.
25. Date and Time:
- Column types for working with date and time values.
26. Binary:
- The
binary
column type stores binary data.
27. Boolean:
boolean
represents true/false or 1/0 values.
28. Enums:
- The
enum
column type enforces predefined values.
29. nullable()
:
- Specifies that a column can hold NULL values.
30. default($value)
:
- Sets a default value for a column.
31. unsigned()
:
- Specifies that a column stores only positive integers.
32. $table->engine
:
- Sets the storage engine for the table.
33. ->after('column_name')
:
- Specifies the position of a newly created column within the table.
34. ->comment('comment')
:
- You can add comments or descriptions to columns using the
comment
method. This helps document your database schema for future reference.
35. ->charset('utf8mb4')
and ->collation('utf8mb4_unicode_ci')
:
- These methods allow you to set the character set and collation for a table, which can be essential for handling multilingual data.
36. dropPrimary()
, dropUnique()
, and dropIndex()
:
- These methods are used to remove primary keys, unique constraints, and indexes from a table, optimizing your database schema.
37. Foreign Keys:
- Laravel supports defining foreign key constraints using the
foreign
method, which helps establish relationships between tables and ensures data integrity.
38. onDelete()
and onUpdate()
:
- These methods allow you to specify actions to be taken when related records are deleted or updated. Options include ‘cascade,’ ‘restrict,’ ‘set null,’ and ‘no action.’
39. Migrations:
- Laravel’s Schema Builder is often used in migration files to version-control your database schema changes and make them reproducible across different environments.
40. Schema Dump:
- Laravel provides the
php artisan schema:dump
command to generate a schema dump file, which can be used to recreate the database schema without running migrations.
41. Reverse Engineering:
- You can generate Laravel migration files from an existing database schema using the
php artisan migrate:generate
command, which helps if you’re starting with an existing database.
42. Index Optimization:
- Laravel’s Schema Builder allows you to optimize your database schema by adding appropriate indexes to speed up query performance.
43. Foreign Key Naming Conventions:
- Laravel follows conventions for naming foreign keys, making it easier to understand the relationships between tables in your database.
44. Column Length Limits:
- Different column types in Laravel have length limits, which are important to consider when designing your database schema to avoid data truncation.
45. Database Agnostic:
- Laravel’s Schema Builder abstracts database-specific SQL, making your application more portable across different database management systems (DBMS).
46. Blueprint Macros:
- You can extend Laravel’s Schema Builder by creating custom macros to simplify repetitive tasks or define specific column types for your application.
47. ipAddress('column_name')
:
- The
ipAddress
method creates an IP address column, allowing you to store IPv4 or IPv6 addresses.
48. json('column_name')
and jsonb('column_name')
:
- These methods create JSON and JSONB (binary JSON) columns for storing structured JSON data in your database.
49. uuid('column_name')
:
- The
uuid
method creates a universally unique identifier (UUID) column, which is a 128-bit value used for identifying records across different systems.
50. year('column_name')
:
- The
year
method creates a column for storing year values, often used for representing years in date data.