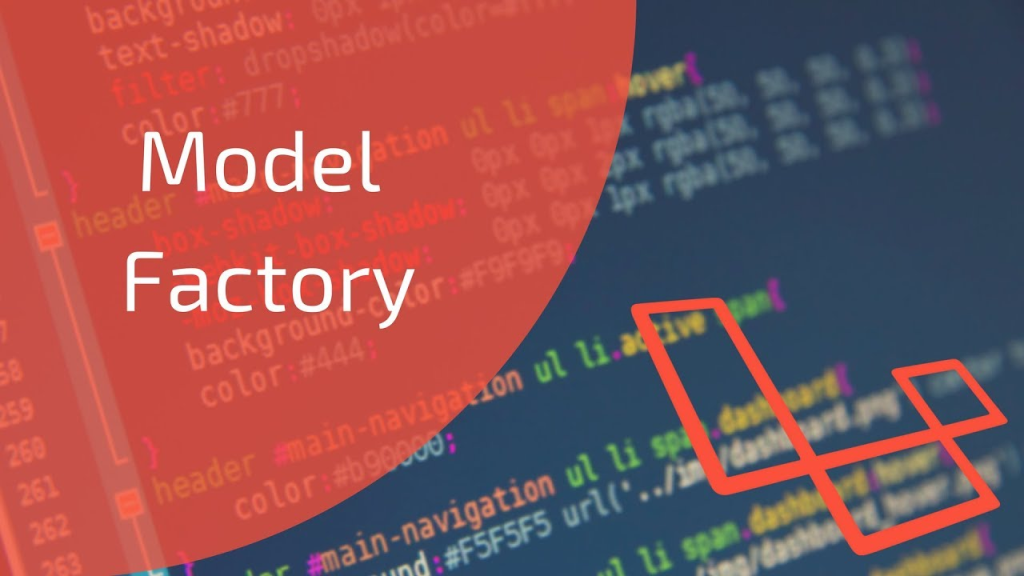
Laravel, one of the most popular PHP frameworks, continues to simplify web development with its elegant syntax and powerful features. Among the many tools Laravel provides, one standout feature is Laravel Factories.
Laravel Factories are a crucial component of Laravel’s testing suite. They are designed to help developers create realistic and meaningful test data for their applications. Instead of manually inserting data into databases or dealing with static test data, Laravel Factories enable developers to generate dynamic and diverse datasets for testing their application’s functionality.
Laravel Factories operate on the concept of model factories, allowing you to define the structure and attributes of your database records in a systematic way. By utilizing these factories, you can easily create multiple instances of your models with varying attributes, facilitating comprehensive testing scenarios.
How to use Laravel factories?
In Laravel, you can use the make:factory
Artisan command to create a new factory. This command generates a factory class file in the database/factories
directory, allowing you to define the structure and default attributes for your Eloquent models. Here’s the basic syntax for creating a factory:
php artisan make:factory FactoryName --model=ModelName
Replace FactoryName
with the desired name for your factory and ModelName
with the name of the Eloquent model associated with the factory. For example, if you have a User
model and you want to create a factory for it named UserFactory
, you would run:
php artisan make:factory UserFactory --model=User
After running this command, Laravel will generate a new factory class file in the database/factories
directory. You can then open this file and define the default attributes for your model.
Here’s an example of what the generated factory file (UserFactory.php
) might look like:
<?php
namespace Database\Factories;
use App\Models\User;
use Illuminate\Database\Eloquent\Factories\Factory;
class UserFactory extends Factory
{
/**
* The name of the factory's corresponding model.
*
* @var string
*/
protected $model = User::class;
/**
* Define the model's default state.
*
* @return array
*/
public function definition()
{
return [
'name' => $this->faker->name,
'email' => $this->faker->unique()->safeEmail,
'password' => bcrypt('password'), // Default password for simplicity
];
}
}
After creating the factory, you can use it in your tests to generate instances of the associated model with realistic and randomized data.