Loops in PHP are control structures that allow you to execute a block of code repeatedly based on a condition. They enable you to automate tasks and iterate over arrays or other data structures.
there are several types of loops
- While Loop
- Do while Loop
- For Loop
- For Each Loop
While Loops
While the loop keeps repeating in action until an associated condition returns a false.
Code
<?php
$num= 1;
while ($num <7)
{
echo "ritik hansda". $num ."</br>";
$num++;
}
echo "loop finished";
?>
Output
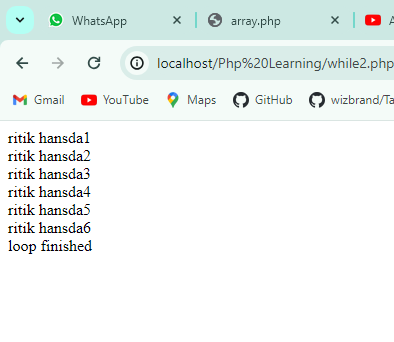
2. Do while Loop
Do-while loop is a control structure that executes a block of code once, and then repeatedly executes the block of code as long as the specified condition is true.
Code
<?php
$counter = 1;
do {
echo "The counter is: $counter <br>";
$counter++;
} while ($counter <= 5);
?>
Output
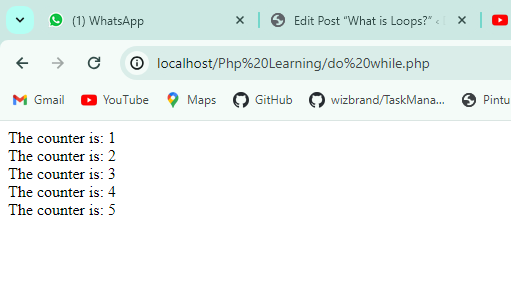
3. For Loop
The for loop in PHP enables the execution of a block of code repeatedly, with a defined initialization, condition, and increment (or decrement) statement.
Code
<?php
for ($i = 1; $i <= 10; $i++) {
echo "The current value of i is: $i <br>";
}
?>
Output
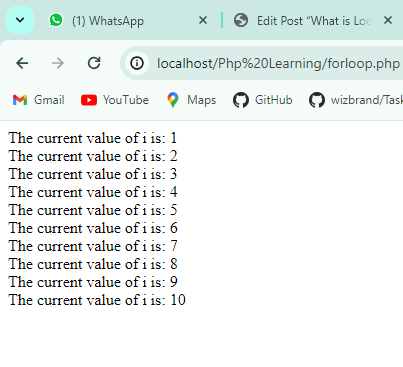
4. For Each Loop
The foreach loop is a control structure used to iterate over arrays and objects. It allows you to execute a block of code for each element in the array or each property in the object.
Code
<?php
$colors = array("red", "green", "blue");
foreach ($colors as $color) {
echo "Color: $color <br>";
}
?>
Output
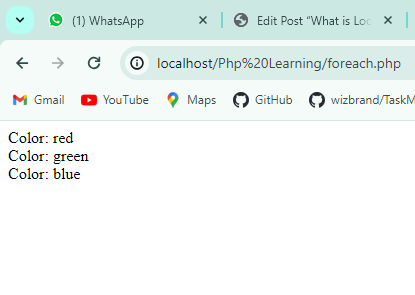