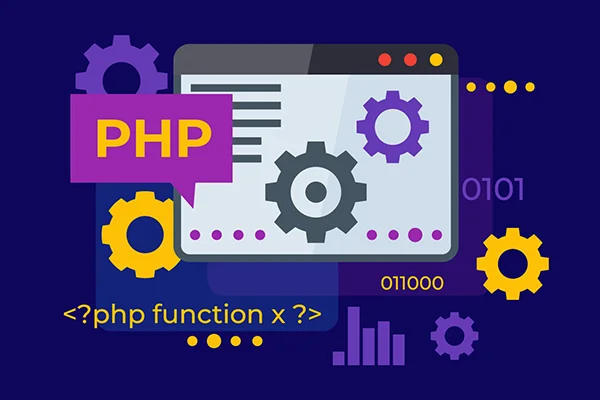
In PHP, a function represents a block of code designed to perform a specific task, aiding in the organization and reusability of code within a program. They can accept inputs, execute operations, and provide outputs.
Syntax:
function functionName($param1, $param2, ...) {
// Code to be executed
return $result; // Optional
}
function
: Keyword to define a function.functionName
: Name assigned to the function.$param1
,$param2
, etc.: Parameters that can be passed into the function (optional).return
: Keyword indicating the value to return from the function (optional).
Example:
function greet($name) {
echo "Hello, $name!";
}
// Calling the function
greet("John");
Parameters and Return Values:
- Parameters: Inputs provided to the function for its operation.
- Return Values: Outputs returned by the function after execution.
function add($num1, $num2) {
$sum = $num1 + $num2;
return $sum;
}
$result = add(5, 3); // $result will be 8
Variable Scope:
Variables declared within a function are locally scoped, accessible only within that function. Global variables, declared outside of functions, are accessible throughout the script, including within functions.
Built-in Functions:
PHP offers a vast library of built-in functions for various tasks such as string manipulation, array handling, date management, etc. These functions can be directly utilized in your code without requiring explicit definition.
By leveraging functions, developers can modularize their code, enhancing readability, reusability, and maintainability of PHP applications.