In PHP, comments play a vital role in code documentation, readability improvement, and fostering collaboration among developers. PHP supports three primary types of comments: single-line comments, multi-line comments, and documentation comments, often known as “doc comments.”
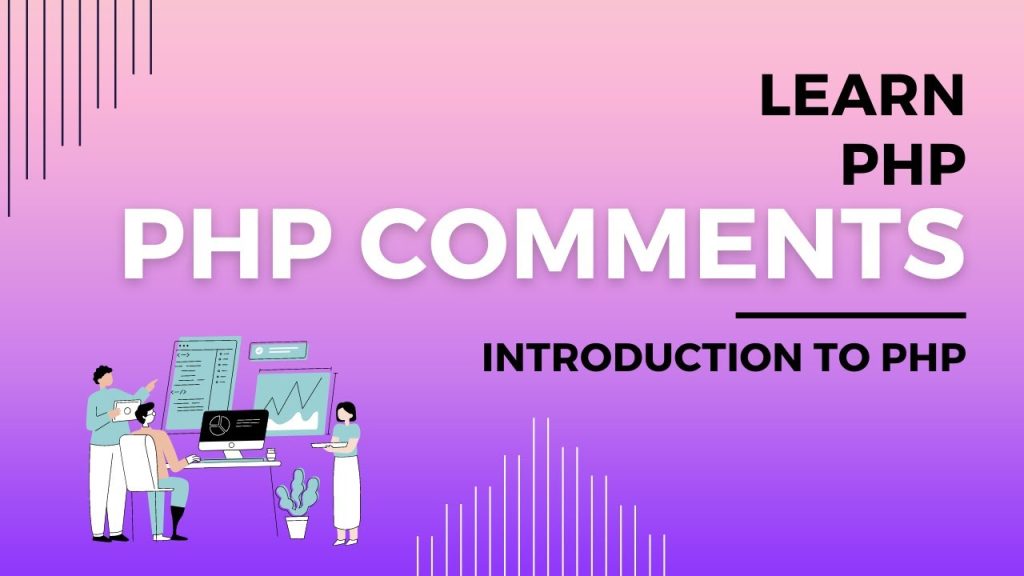
Single-Line Comments
Single-line comments are initiated with //
and extend to the end of the line. They are suitable for brief explanations or annotations within the code.
// This is a single-line comment
$name = "John"; // Assigning a value to the variable $name
Multi-Line Comments
Multi-line comments start with /*
and end with */
. They can span across multiple lines, making them useful for commenting out large sections of code or providing block-level descriptions.
/*
This is a multi-line comment.
It can span across multiple lines.
*/
$age = 30;
Documentation Comments (Doc Comments)
Documentation comments serve a special purpose in generating automated documentation. Typically placed above classes, functions, or methods, they follow specific formats like PHPDoc or Doxygen.
/**
* This function calculates the sum of two numbers.
*
* @param int $a The first number
* @param int $b The second number
* @return int The sum of $a and $b
*/
function add($a, $b) {
return $a + $b;
}
PHPDoc Tags
@param
: Describes function or method parameters.@return
: Describes the return value.@var
: Describes variable types.@throws
: Describes exceptions.@deprecated
: Indicates deprecated functions or methods.
Importance of Comments
Comments are crucial for:
- Code Documentation: They provide insights into code functionality and purpose.
- Code Readability: Well-commented code is easier to understand.
- Collaboration: They facilitate collaboration among developers by providing context within the codebase.
Best Practices
- Descriptive Comments: Explain why code exists, not just how it works.
- Updated Comments: Keep comments updated with code changes.
- Avoid Overcommenting: Comments should add value without adding noise.
- Meaningful Names: Use meaningful variable, function, and class names to minimize the need for comments.
Example
“`php
// Calculate the total price
$total = $price * $quantity;
/*
This code block retrieves user data from the database
and assigns it to the $user variable.
*/
$user = getUserData($userId);
/**
- Display a greeting message.
* - @param string $name The name of the user
*/
function greet($name) {
echo “Hello, $name!”;
}