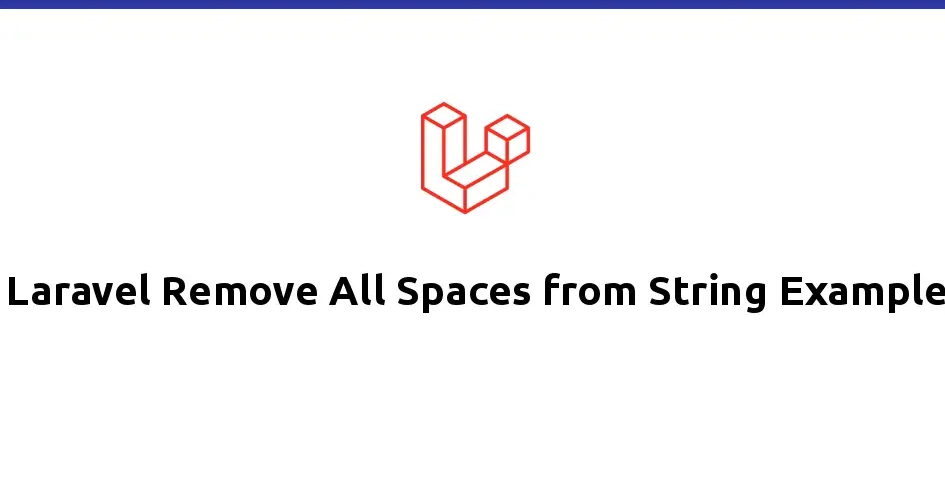
In Laravel, data manipulation is a fundamental part of web development. One common task is removing spaces from strings, which can be essential for various purposes such as sanitizing user input, formatting data, or generating URLs. In this guide, we’ll explore how to remove all spaces from a string in a Laravel project using the str_replace()
function. This method works seamlessly across different Laravel versions, including Laravel 6, Laravel 7, Laravel 8, Laravel 9, and Laravel 10.
The provided code snippet demonstrates how to remove spaces from a string using Laravel’s str_replace()
function. Let’s break it down step by step.
use App\Http\Controllers\Controller;
class DemoController extends Controller
{
public function index()
{
$string = "Hi, This is Test";
$string = str_replace(' ', '', $string);
dd($string);
}
}
Controller and Namespace: We begin by importing the necessary dependencies and specifying the namespace for the DemoController
.
index
Method: Inside the DemoController
, there is an index
method. In a real-world scenario, this method might be part of a route that responds to a specific HTTP request.
String Variable: We declare a string variable $string
with the value "Hi, This is Test"
. This is the string from which we want to remove spaces.
str_replace()
Function: The heart of this operation is the str_replace()
function. It takes three arguments:
dd()
Function: Finally, we use the dd()
function (short for “dump and die”) to display the modified string. After removing spaces, the result is stored back in the $string
variable.
Output
The expected output of this code is a string with all spaces removed. In this case, the output will be.
"Hi,ThisisTest"