In PHP, variable functions allow you to use variables to dynamically call functions. This powerful feature can make your code more flexible and dynamic. This tutorial covers all the essential aspects of PHP variable functions, including their definition, usage, and examples.
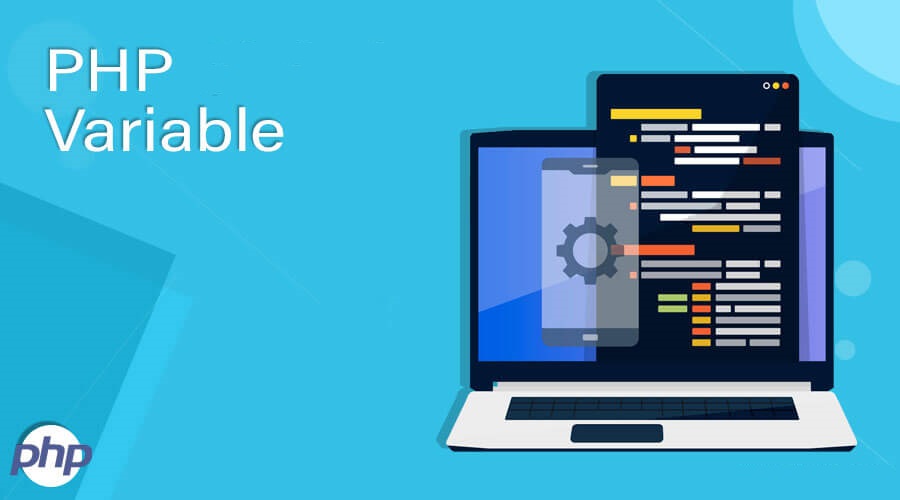
1. Introduction to PHP Variable Functions
A variable function in PHP refers to using a variable that contains the name of a function to call that function. This can be particularly useful when you want to decide which function to call dynamically at runtime.
2. Basic Usage of Variable Functions
Defining and Calling Variable Functions
To use a variable function, you simply assign the function name (as a string) to a variable and then use that variable to call the function.
<?php
function sayHello() {
echo "Hello, World!";
}
$func = 'sayHello';
$func(); // Calls sayHello()
?>
Output:-
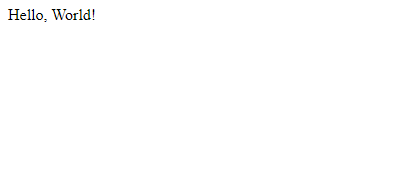
3. Passing Parameters to Variable Functions
Variable functions can also accept parameters. When calling the function, you pass the parameters as you would with a regular function.
<?php
function greet($name) {
echo "Hello, $name!";
}
$func = 'greet';
$func('Cotocus');
?>
Output:-
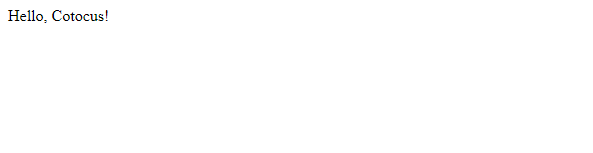
4. Using Variable Functions with Class Methods
Variable functions can also be used with class methods. Both static and instance methods can be called this way.
Static Methods
<?php
class Greeter {
public static function sayHello() {
echo "Hello from static method!";
}
}
$func = ['Greeter', 'sayHello'];
$func(); // Calls Greeter::sayHello()
?>
Output:-
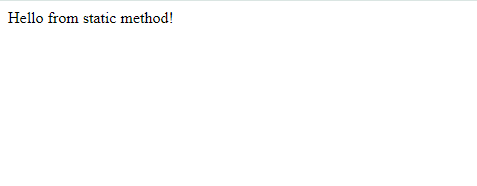
Instance Methods
<?php
class Greeter {
public function sayHello() {
echo "Hello from instance method!";
}
}
$greeter = new Greeter();
$func = [$greeter, 'sayHello'];
$func(); // Calls $greeter->sayHello()
?>
Output:-
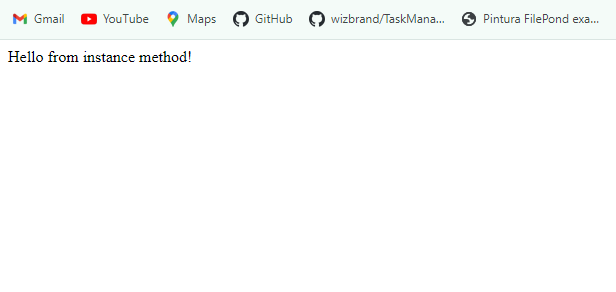
5. Advanced Usage of Variable Functions
Array of Functions
You can store multiple function names in an array and iterate over them to call each function dynamically.
<?php
function func1() {
echo "Function 1\n";
}
function func2() {
echo "Function 2\n";
}
$functions = ['func1', 'func2'];
foreach ($functions as $func) {
$func();
}
?>
Output:-
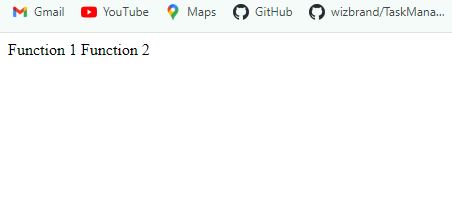
Dynamic Function Selection
You can use conditional statements to dynamically choose which function to call based on certain conditions.
<?php
function add($a, $b) {
return $a + $b;
}
function subtract($a, $b) {
return $a - $b;
}
$operation = 'add';
$result = $operation(5, 3);
echo $result;
?>
Output:
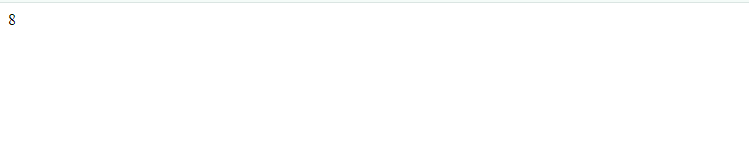
6. Practical Examples
Example 1: Calculator
Here’s a simple calculator that uses variable functions to perform different operations.
<?php
function add($a, $b) {
return $a + $b;
}
function subtract($a, $b) {
return $a - $b;
}
function multiply($a, $b) {
return $a * $b;
}
function divide($a, $b) {
if ($b != 0) {
return $a / $b;
} else {
return "Division by zero error!";
}
}
$operation = 'multiply';
$a = 10;
$b = 5;
if (function_exists($operation)) {
echo $operation($a, $b);
} else {
echo "Invalid operation";
}
?>
Output:-
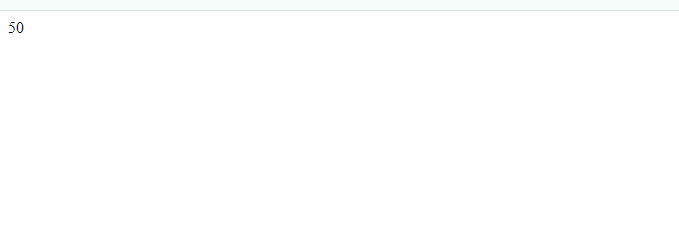
7. Best Practices
- Validate Function Existence: Always check if the function exists using
function_exists()
before calling it to avoid runtime errors. - Use Meaningful Names: Ensure that the function names stored in variables are meaningful and descriptive.
- Security Considerations: Be cautious when using user input to determine function names, as it can lead to security vulnerabilities. Always validate and sanitize user inputs.