Introduction
PHP provides two fundamental constructs for outputting data: echo
and print
. Both are used to display information to the user, but there are subtle differences between them. This tutorial will explore these differences, how to use both constructs effectively, and some practical examples.
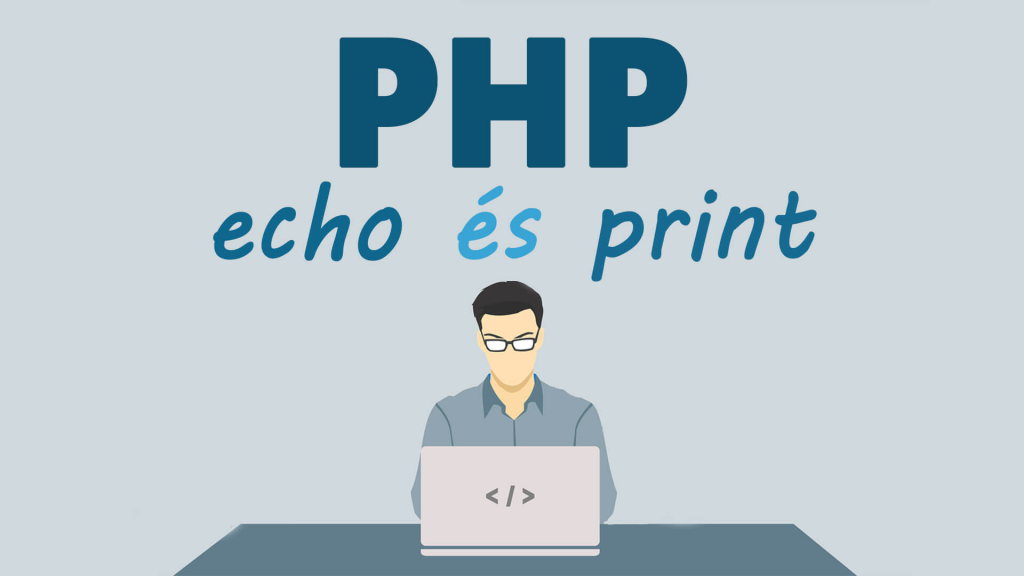
Echo Statement
Characteristics
- No Return Value:
echo
does not return any value. - Multiple Parameters:
echo
can take multiple parameters separated by commas. - Performance:
echo
is slightly faster thanprint
because it does not return a value.
Basic Usage
You can use echo
to display a string.
<?php
echo "Hello, World!"; // Outputs: Hello, World!
?>
Using Parentheses
Although echo
is not a function, you can use parentheses.
<?php
echo("Hello, World!"); // Outputs: Hello, World!
?>
Multiple Parameters
You can pass multiple strings to echo
, separated by commas.
<?php
echo "Hello", ", ", "World", "!"; // Outputs: Hello, World!
?>
Outputting Variables
You can use echo
to output the value of variables.
<?php
$greeting = "Hello";
$name = "World";
echo $greeting . ", " . $name . "!"; // Outputs: Hello, World!
?>
Outputting HTML
echo
is often used to output HTML code.
<?php
echo "<h1>Hello, World!</h1>"; // Outputs: <h1>Hello, World!</h1>
?>
Combining Strings and Variables
You can combine strings and variables directly within echo
.
<?php
$name = "World";
echo "Hello, $name!"; // Outputs: Hello, World!
?>
Print Statement
Characteristics
- Return Value:
print
always returns1
, making it useful in expressions. - Single Argument:
print
can only take one argument. - Performance:
print
is slightly slower thanecho
due to its return value.
Basic Usage
You can use print
to display a string.
<?php
print "Hello, World!"; // Outputs: Hello, World!
?>
Using Parentheses
Like echo
, print
can be used with parentheses.
<?php
print("Hello, World!"); // Outputs: Hello, World!
?>
Return Value
Since print
returns 1
, it can be used in expressions.
<?php
if (print "Hello, World!") {
print " This will also be printed."; // Outputs: Hello, World! This will also be printed.
}
?>
Outputting Variables
You can use print
to output variables.
<?php
$greeting = "Hello";
$name = "World";
print $greeting . ", " . $name . "!"; // Outputs: Hello, World!
?>
Outputting HTML
print
can also be used to output HTML code.
<?php
print "<h1>Hello, World!</h1>"; // Outputs: <h1>Hello, World!</h1>
?>
Differences Between Echo and Print
- Return Value:
echo
does not return a value, whileprint
returns1
. - Multiple Parameters:
echo
can take multiple parameters, whileprint
can only take one. - Performance:
echo
is slightly faster thanprint
.
Practical Examples
Example 1: Using Echo to Output HTML
<?php
echo "<div>";
echo "<h1>Welcome to my website</h1>";
echo "<p>This is a sample paragraph.</p>";
echo "</div>";
?>
Example 2: Using Print to Output Variables
<?php
$name = "John";
$age = 30;
print "Name: " . $name . "<br>";
print "Age: " . $age . "<br>";
?>
Example 3: Using Echo with Multiple Parameters
<?php
echo "This ", "string ", "was ", "made ", "with multiple parameters.";
?>
Example 4: Using Print in an Expression
“`php