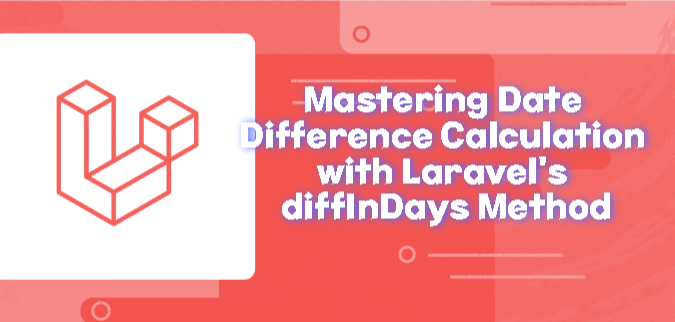
When working with dates in Laravel applications, often we need to calculate the difference between two dates. For example, we might want to know how many days have passed since a particular event or how far apart two dates are. Laravel provides a convenient method called diffInDays
to precisely handle such scenarios.
Understanding diffInDays Method
The diffInDays method is part of Laravel’s built-in support for the Carbon library, which offers a fluent API for working with dates and times. Carbon instances provide various methods to perform date calculations easily, and diffInDays is one of them.
This method calculates the difference in days between two Carbon instances. It returns the number of days between the two dates, with positive values indicating that the second date is after the first date and negative values indicating the opposite.
Examples of how to use diffInDays in your Laravel applications:
Example 1: Calculate Days Since an Event
Suppose you have a blog post model with a published_at attribute, and you want to display how many days have passed since the post was published.
use App\Models\Post;
use Illuminate\Support\Carbon;
$post = Post::find($postId);
$daysSincePublished = $post->published_at->diffInDays(Carbon::now());
echo "Days since publication: $daysSincePublished";
Example 2: Check if a Deadline Has Passed
Imagine you have a task model with a deadline attribute, and you need to determine if the deadline has passed.
use App\Models\Task;
use Illuminate\Support\Carbon;
$task = Task::find($taskId);
$isDeadlinePassed = $task->deadline->diffInDays(Carbon::now()) < 0;
if ($isDeadlinePassed) {
echo "Deadline has passed!";
} else {
echo "You still have time!";
}
Example 3: Calculate Age
You can even calculate someone’s age based on their birthdate.
use App\Models\User;
use Illuminate\Support\Carbon;
$user = User::find($userId);
$age = $user->birthdate->diffInYears(Carbon::now());
echo "Age: $age years old";