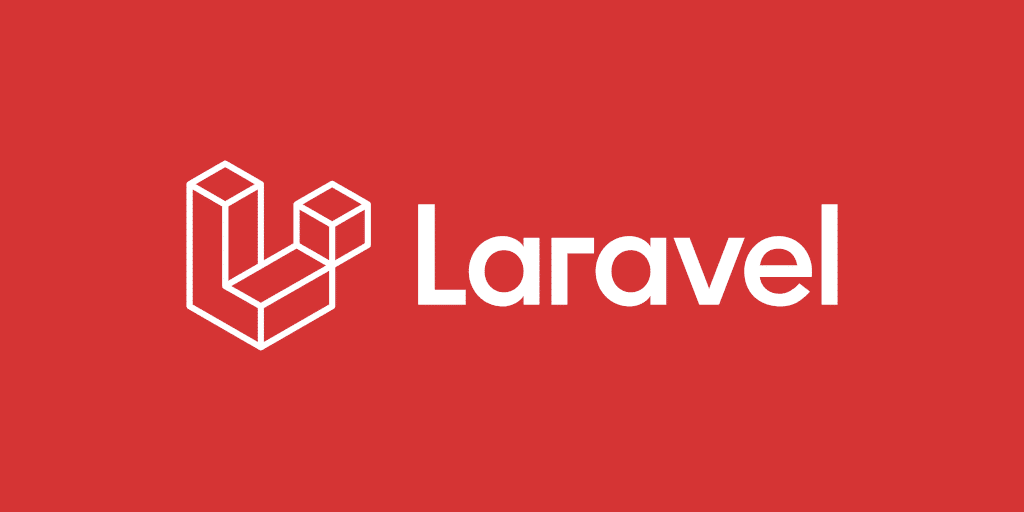
When starting a new project with Laravel, developers are often amazed by the robust set of features and tools that come right out of the box. Laravel, known for its elegance and developer-friendly approach, provides a rich set of default packages that streamline development and make building web applications a breeze.
Introduction to Laravel
Laravel, a PHP web application framework, has gained immense popularity in the web development community for its expressive syntax, powerful features, and modern development practices. With each version release, Laravel continues to evolve, introducing new functionalities while maintaining its user-friendly nature.
Default Packages in Laravel 10
Let’s take a closer look at some of the default packages and components that Laravel 10 provides:
Blade Templating Engine: Laravel’s Blade templating engine offers a simple yet powerful way to write views. With Blade, developers can create reusable templates, include partials, and easily manage layout files.
Here are some key features and concepts of the Blade templating engine:
- Template Inheritance: Blade allows you to define a master layout file that serves as the base template for your application. You can then create child templates that extend the master layout and override specific sections as needed. This promotes code reusability and helps maintain a consistent layout across your application.
- Control Structures: Blade provides familiar control structures such as if statements, loops, and switch statements, allowing you to conditionally render content or iterate over data within your templates. Blade’s control structures are concise and easy to read, making it straightforward to manipulate data and control the flow of your views.
- Template Includes: Blade enables you to include other Blade templates within your views using the
@include
directive. This allows you to modularize your templates and reuse common components across multiple views. You can also pass data to included templates, making them dynamic and flexible. - Escaping Output: Blade automatically escapes output by default to help prevent XSS (Cross-Site Scripting) attacks. This means that any user input rendered in your views will be automatically sanitized, reducing the risk of malicious code injection. However, you can also explicitly output unescaped content using the
{!! !!}
syntax when necessary. - Custom Directives: Blade allows you to define custom directives using the
@directive
syntax. This enables you to extend Blade’s functionality and create your own reusable template macros or shortcuts. Custom directives can streamline your template code and encapsulate complex logic into simple, expressive statements. - Conditional Statements: Blade provides convenient shortcuts for common conditional statements, such as the
@if
,@else
,@elseif
, and@unless
directives. These directives make it easy to conditionally render content based on the evaluation of variables or expressions, improving the readability and clarity of your templates.
Eloquent ORM (Object-Relational Mapping):
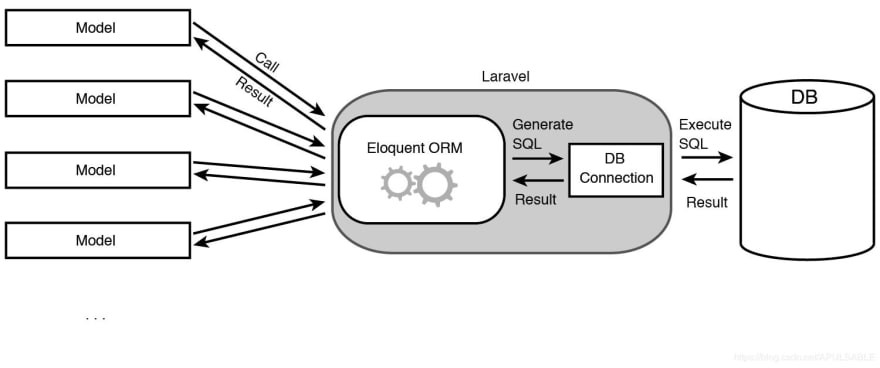
Eloquent is Laravel’s elegant ActiveRecord implementation, providing a convenient way to interact with the database. With Eloquent, developers can define models and perform database operations using intuitive methods and conventions.
- Model-View-Controller (MVC) Architecture: Eloquent follows the MVC pattern, where models represent data structures and business logic, views handle presentation logic, and controllers act as intermediaries between models and views. Eloquent models map database tables to PHP objects, providing a convenient way to work with database records.
- Database Table Relationships: Eloquent allows you to define relationships between database tables using intuitive methods such as
belongsTo
,hasMany
,hasOne
,belongsToMany
, andmorphTo
. These relationships enable you to establish connections between different models, making it easy to retrieve related data and navigate complex data structures. - CRUD Operations: Eloquent provides simple and expressive methods for performing CRUD (Create, Read, Update, Delete) operations on database records. For example, you can use the
create
method to insert a new record, thefind
method to retrieve a record by its primary key, and theupdate
anddelete
methods to modify or remove records. - Query Builder: Eloquent includes a query builder that allows you to construct database queries using method chaining and fluent syntax. You can use methods such as
where
,orderBy
,groupBy
,join
, andselect
to build complex queries without writing raw SQL code. Eloquent automatically translates these methods into SQL queries behind the scenes. - Model Events and Observers: Eloquent provides hooks for model events such as
creating
,created
,updating
,updated
,deleting
, anddeleted
. You can define event listeners, called observers, to perform additional logic before or after certain model events occur. This allows you to encapsulate business logic within your models and keep your code organized. - Mass Assignment Protection: Eloquent includes built-in protection against mass assignment vulnerabilities by allowing you to specify which model attributes are mass assignable using the
fillable
property. This helps prevent unauthorized users from modifying sensitive model attributes through HTTP requests.
Artisan Console:
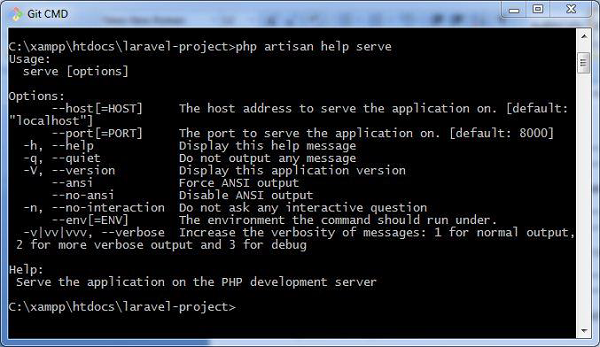
Artisan is Laravel’s command-line interface, offering a wide range of commands for scaffolding, database migrations, testing, and more. With Artisan, developers can automate repetitive tasks and streamline their workflow.
- Code Generation: Artisan allows you to quickly generate boilerplate code for various components of your Laravel application. For example, you can use Artisan to create controllers, models, migrations, seeders, middleware, and more using simple command-line instructions. This saves you time and effort by automating the initial setup of your application’s structure.
- Database Migrations: Laravel’s migration system enables you to define and manage your database schema using PHP code rather than SQL queries. Artisan provides commands to create and run database migrations, allowing you to easily modify your database structure and keep it in sync with your application’s codebase. Migrations also facilitate database version control and collaboration among team members.
- Database Seeding: Artisan includes commands for seeding your database with sample or test data. This is particularly useful during development and testing phases when you need to populate your database with realistic data for evaluation and debugging purposes. Seeders help ensure consistent and reproducible database states across different environments.
- Task Scheduling: Laravel’s task scheduling feature allows you to define scheduled tasks that run at specified intervals or times. Artisan provides commands for managing scheduled tasks, including listing scheduled tasks, running scheduled tasks manually, and checking task execution logs. This enables you to automate recurring tasks such as sending emails, generating reports, and performing data cleanup.
- Application Configuration: Artisan provides commands for managing your application’s configuration settings. You can use these commands to view, modify, and cache configuration values stored in the
config
directory of your Laravel project. This allows you to customize various aspects of your application, such as database connections, caching drivers, session settings, and more. - Code Maintenance: Artisan offers commands for performing routine code maintenance tasks, such as clearing application caches, optimizing class loading, generating optimized autoload files, and refreshing application routes. These commands help keep your application running smoothly and efficiently by clearing stale data and reloading cached resources.
- Custom Commands: In addition to built-in commands, you can create your own custom Artisan commands to extend the functionality of your Laravel application. Custom commands allow you to encapsulate complex or repetitive tasks in reusable scripts that can be executed from the command line. This provides flexibility and scalability in managing your application’s development and deployment processes.
Routing System:
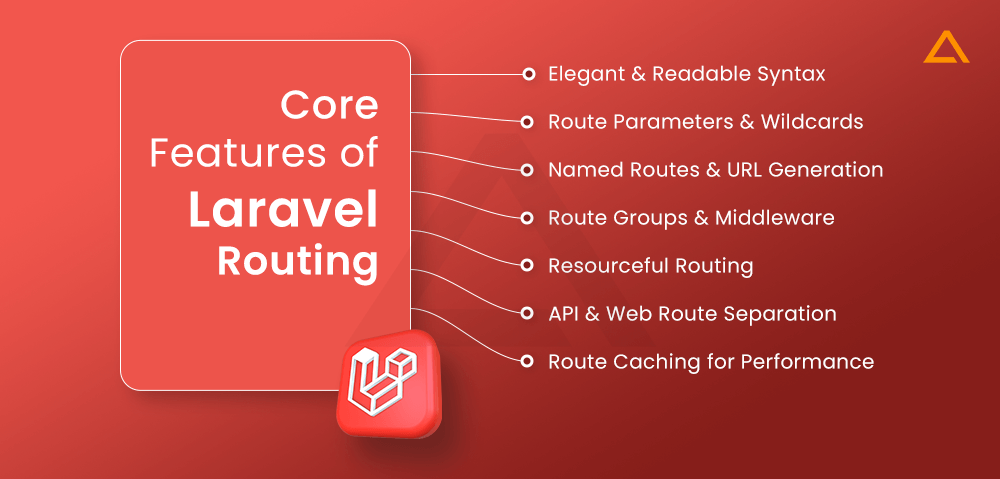
Laravel’s routing system allows developers to define clean and expressive routes for their application. Routes can handle various HTTP request methods and parameters, making it easy to build RESTful APIs and web applications.
- Definition of Routes: In Laravel, routes are defined in the
routes
directory of your application, primarily within theweb.php
andapi.php
files. These route files contain route definitions that map specific URLs (or URIs) to corresponding controller actions or closures. - HTTP Verbs and URIs: Routes are typically defined using HTTP verbs (such as GET, POST, PUT, DELETE) and URIs (Uniform Resource Identifiers) to match incoming requests. For example, a route may be defined to respond to GET requests to the
/products
URI. - Route Parameters: Laravel allows you to define dynamic route parameters within your route definitions, denoted by curly braces
{}
. These parameters capture specific segments of the requested URI and pass them as arguments to the corresponding controller action. For example, a route may be defined with a parameter for the product ID:/products/{id}
. - Named Routes: Routes can also be assigned names, which provide a convenient way to generate URLs or redirects within your application using Laravel’s helper functions. Named routes help avoid hardcoding URLs in your application and make it easier to maintain and update route definitions.
- Route Groups and Middleware: Laravel allows you to group related routes together using route groups. Route groups enable you to apply middleware, prefixes, namespaces, and other common attributes to multiple routes simultaneously, reducing redundancy and improving organization.
- Route Caching: In production environments, Laravel offers route caching as a performance optimization technique. Route caching generates a cached copy of your application’s route definitions, reducing the overhead of parsing and compiling routes on each request.
- API Routes: Laravel provides separate route files (
api.php
) specifically for defining routes intended for API endpoints. API routes often follow RESTful conventions and are typically used for serving JSON responses to client-side applications or mobile devices. - Route Model Binding: Laravel’s route model binding feature automatically injects model instances into route parameters based on their IDs or other unique identifiers. This simplifies controller logic by abstracting away the manual retrieval of model instances from the database.
Middleware:
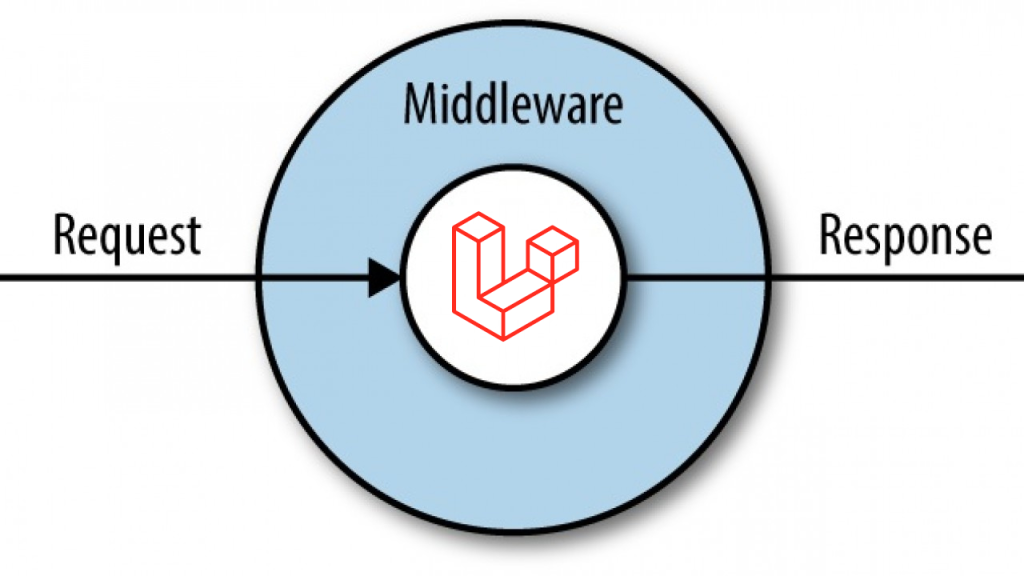
Laravel’s middleware provides a flexible way to filter HTTP requests entering the application. Middleware can perform tasks such as authentication, authorization, logging, and more, helping developers write clean and maintainable code.
- Request Pipeline: When an HTTP request enters your Laravel application, it passes through a series of middleware before reaching the intended route handler. Middleware intercepts the request at various stages of the request-response cycle, allowing you to inspect, modify, or terminate the request as needed.
- Stacked Middleware: Laravel middleware can be assigned globally to apply to all routes or assigned to specific routes or route groups. Middleware can be stacked in the order they should be executed, allowing you to define complex processing pipelines tailored to your application’s requirements.
- Middleware Classes: Middleware in Laravel is typically implemented as classes that implement the
handle
method. This method receives the incoming request and optionally performs actions before passing the request to the next middleware in the pipeline. Middleware classes can modify the request, perform authentication or authorization checks, and terminate the request if necessary. - Request Modification: Middleware can modify incoming requests by adding headers, modifying request parameters, or redirecting the request to a different URL. For example, you can use middleware to enforce HTTPS redirection, set CORS headers, or log requests for auditing purposes.
- Authentication and Authorization: Middleware plays a vital role in implementing authentication and authorization in Laravel applications. The built-in
auth
middleware, for example, verifies that the current user is authenticated before allowing access to protected routes. Similarly, you can define custom middleware to enforce access control policies based on user roles or permissions. - Response Manipulation: In addition to intercepting incoming requests, middleware can also intercept outgoing responses. This allows you to modify the response content, add headers, or perform post-processing tasks before sending the response back to the client.
- Error Handling: Middleware can also handle exceptions and errors that occur during the request processing pipeline. By defining error-handling middleware, you can gracefully handle exceptions, log errors, and return custom error responses to the client.
Session Management:
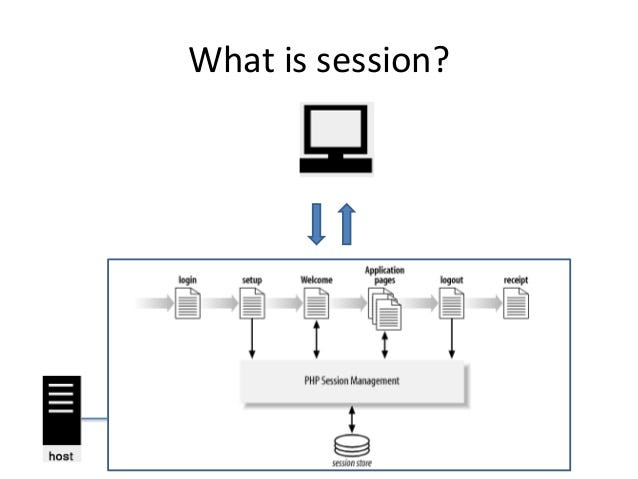
Laravel comes with built-in support for managing session data, allowing developers to store and retrieve user-specific information across requests. Laravel’s session management system offers drivers for file, cookie, database, and more.
- Session Drivers: Laravel supports multiple session drivers out of the box, including file, cookie, database, Memcached, Redis, and array drivers. You can configure the session driver in the
config/session.php
configuration file. Each driver has its own advantages and use cases, depending on factors such as performance, scalability, and persistence. - Session Configuration: You can customize various aspects of session management in Laravel through the configuration file mentioned above. This includes specifying the session lifetime, cookie parameters, encryption settings, and more. Laravel’s configuration options make it easy to tailor session management to the specific requirements of your application.
- Session Middleware: Laravel provides middleware for managing sessions, including the
StartSession
middleware, which initializes the session for each incoming request. This middleware ensures that session data is available throughout the request lifecycle, allowing you to store and retrieve session variables within your application’s routes and controllers. - Session Variables: In Laravel, you can store and retrieve session data using the
session()
helper function or theSession
facade. This allows you to persist user-specific information, such as authentication status, shopping cart contents, user preferences, and more, across multiple requests. Session variables are stored securely and can be accessed across different parts of your application. - Flash Data: Laravel’s session management includes support for flash data, which is data that is only available for the next request and is automatically deleted thereafter. Flash data is commonly used for displaying status messages, such as success or error messages, to users after a form submission or other action.
- Session Drivers: Laravel’s session drivers provide mechanisms for storing session data on the server or client side. For example, the file driver stores session data on the server’s file system, while the cookie driver stores session data in encrypted cookies sent to the client’s browser. Each driver has its own advantages and trade-offs, allowing you to choose the most appropriate option based on your application’s requirements.
Authentication and Authorization:
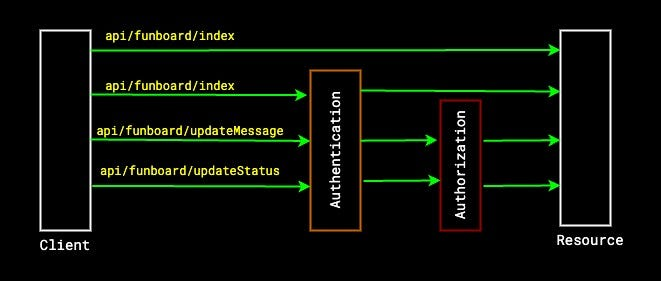
Laravel provides comprehensive support for user authentication and authorization out of the box. Developers can easily set up authentication functionality, including registration, login, password reset, and role-based access control.
- Authentication: Authentication is the process of verifying the identity of a user. It ensures that the user is who they claim to be before granting access to the system or certain resources within the system. In web applications, authentication commonly involves users providing credentials, such as a username and password, which are then compared against stored credentials in the system’s database.
- Types of Authentication: There are various authentication methods, including:
- Password-based Authentication: Users provide a username and password.
- Token-based Authentication: Users provide a token (e.g., JSON Web Token) generated after successful login.
- OAuth Authentication: Users log in using their credentials from a third-party service (e.g., Google, Facebook).
- Biometric Authentication: Users authenticate using unique biological traits (e.g., fingerprint, face recognition).
- Authentication Process: The authentication process typically involves the following steps:
- User submits credentials.
- System validates credentials.
- If credentials are valid, the user is considered authenticated and granted access.
- Types of Authentication: There are various authentication methods, including:
- Authorization: Authorization is the process of determining what actions a user is allowed to perform within the system after authentication. It involves defining and enforcing access control policies based on the user’s identity, roles, and permissions. Authorization ensures that users can only access resources or perform actions that they have been explicitly granted permission to access.
- Access Control: Access control mechanisms enforce authorization policies by:
- Role-Based Access Control (RBAC): Assigning users to roles, and defining permissions for each role.
- Attribute-Based Access Control (ABAC): Making access decisions based on attributes associated with users, resources, and environmental conditions.
- Policy-Based Access Control (PBAC): Using predefined policies to determine access rights.
- Authorization Process: The authorization process typically involves the following steps:
- User requests access to a resource or performs an action.
- System evaluates the user’s permissions based on their identity and associated roles.
- If the user has the necessary permissions, access is granted; otherwise, access is denied.
- Granularity: Authorization can be fine-grained, allowing administrators to specify permissions at the individual resource or action level, or coarse-grained, where permissions are applied at a broader level.
- Access Control: Access control mechanisms enforce authorization policies by:
Database Migrations and Seeding:
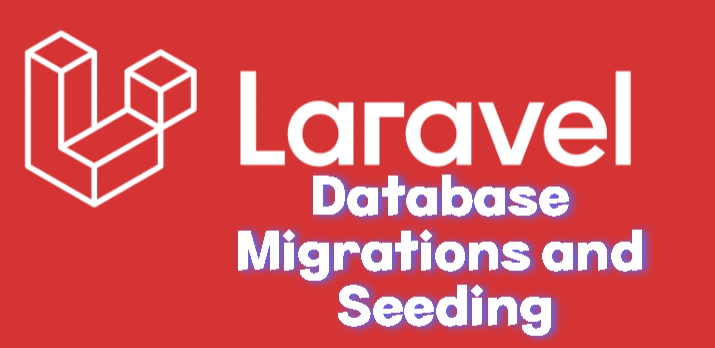
Laravel’s migration system enables developers to define and manage database schemas using PHP code. Additionally, Laravel offers database seeding functionality, allowing developers to populate databases with test data.
- Schema Definition: In Laravel, database migrations are defined using a fluent, expressive syntax that closely resembles SQL. You can create new tables, modify existing tables, add columns, define indexes, and perform other schema-related tasks using migration files.
- Migration Files: Migration files are PHP classes stored in the
database/migrations
directory of your Laravel application. Each migration file contains two methods:up()
anddown()
. Theup()
method defines the changes to be applied to the database, while thedown()
method specifies how to revert those changes if necessary. - Running Migrations: You can run migrations using the Artisan command-line interface provided by Laravel. By running
php artisan migrate
, Laravel will execute all pending migrations that haven’t been run yet. This applies the changes defined in theup()
method of each migration file to the database. - Rolling Back Migrations: If you need to revert a migration, you can use the
php artisan migrate:rollback
command. This will execute thedown()
method of the most recently migrated batch of migrations, undoing the changes made to the database. - Migration Status: You can check the status of migrations using the
php artisan migrate:status
command. This command displays which migrations have been run and which are pending.
Testing Support:
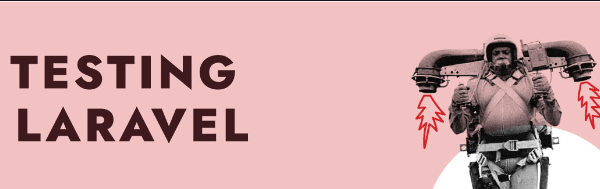
Laravel includes support for writing and running automated tests using PHPUnit. Developers can write unit tests, feature tests, and browser tests to ensure the quality and reliability of their applications.
- PHPUnit Integration: Laravel utilizes PHPUnit, a popular testing framework for PHP, to support various types of tests such as unit tests, feature tests, and browser tests. PHPUnit is integrated into Laravel’s testing infrastructure, allowing developers to write test cases using PHPUnit’s syntax and assertions.
- Test Directory Structure: Laravel organizes test files in the
tests
directory of your application. Within this directory, you’ll find subdirectories for different types of tests, includingUnit
,Feature
, andBrowser
tests. This structure helps maintain a clean and organized approach to testing. - Preconfigured Environment: Laravel provides a preconfigured testing environment that closely mirrors your application’s production environment. This environment includes a separate database for testing purposes, allowing you to perform tests without affecting your production data.
- Testing Database Transactions: Laravel wraps each test case in a database transaction by default. This means that any changes made to the database during a test are rolled back after the test completes, ensuring that your database remains in a consistent state between tests.
- Assertions and Helpers: Laravel provides a variety of helper methods and assertions to simplify the process of writing tests. These helpers cover common testing scenarios such as asserting database records, making HTTP requests, interacting with forms, and more.
- Mocking and Dependency Injection: Laravel’s testing support includes features for mocking objects and injecting dependencies, which are essential for isolating and testing individual components of your application in isolation.