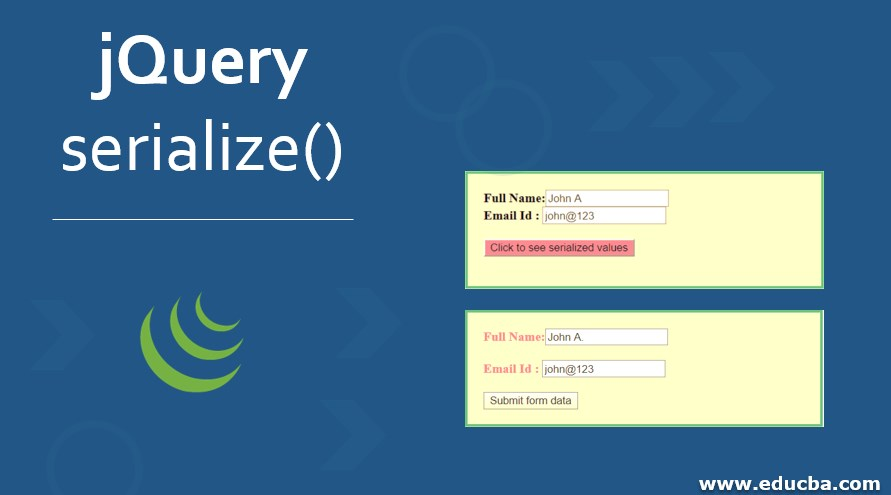
The formObj.serialize()
method is a jQuery method used to serialize form data into a query string format. It allows you to easily extract data from HTML form elements and convert it into a format suitable for transmission via HTTP requests, such as GET or POST.
Properties and Usage:
- Simplicity: One of the key properties of
formObj.serialize()
is its simplicity. With just a single method call, you can serialize an entire form and obtain a string representation of its data. - Form Element Selection: The
formObj
parameter refers to the jQuery object representing the form element you want to serialize. This could be obtained using jQuery selectors or by directly referencing the form element. - Data Serialization: When called on a form element,
formObj.serialize()
serializes all the form controls within the form, including input fields, text areas, and select elements. It collects the name-value pairs of the form controls and concatenates them into a query string format. - Query String Format: The serialized form data is encoded in the standard URL-encoded query string format, where name-value pairs are separated by ‘&’ characters, and names and values are URL-encoded.
Example:
Consider the following HTML form.
<form id="myForm">
<input type="text" name="username" value="John">
<input type="email" name="email" value="john@example.com">
<input type="checkbox" name="subscribe" value="1" checked>
</form>
To serialize this form using formObj.serialize()
, you can use the following JavaScript code.
var formData = $('#myForm').serialize();
console.log(formData);
The output would be
username=John&email=john%40example.com&subscribe=1
Ease of Use: formObj.serialize()
simplifies the process of extracting form data, eliminating the need for manual traversal of form elements.
Compact Data Representation: The serialized form data is concise and suitable for inclusion in URL query strings or as the body of POST requests.
Compatibility: The method is widely supported across browsers and can be used in various web development scenarios.