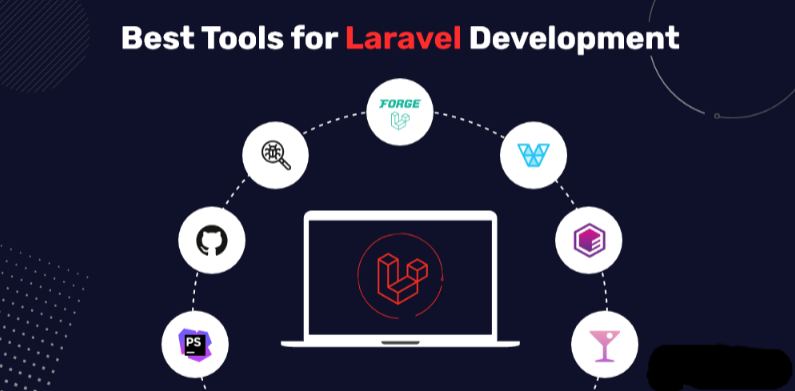
Improving your Laravel website involves utilizing various tools, technologies, and packages to enhance performance, security, user experience, and development workflow. Here’s a list of some tools, technologies, and packages you can consider integrating into your Laravel project:
Laravel Mix:
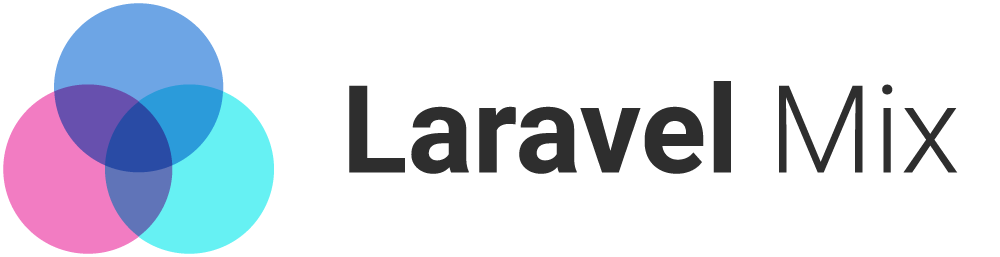
Laravel Mix provides a fluent API for defining webpack build steps for your Laravel application, making asset compilation and optimization simple.
Laravel Mix is a powerful tool provided by the Laravel framework for simplifying and streamlining the process of asset compilation and management in web applications. It’s built on top of webpack, a popular module bundler for JavaScript applications, but abstracts away much of the complexity of webpack configuration, making it more accessible to developers.
Simplified Asset Compilation: Laravel Mix provides a clean and fluent API for defining asset compilation tasks such as compiling CSS, JavaScript, and other assets like Sass, Less, and TypeScript. You can define these tasks using a simple and intuitive syntax, without needing to write complex webpack configuration files manually.
Webpack Integration: Under the hood, Laravel Mix leverages webpack to handle asset compilation. However, you don’t need to interact directly with webpack’s configuration files. Laravel Mix abstracts away webpack’s complexities, allowing you to focus on defining compilation tasks using a more straightforward API.
Pre-configured Webpack: Laravel Mix comes pre-configured with sensible defaults for common web development tasks. This includes settings for handling CSS preprocessing, JavaScript transpilation, minification, and code splitting. These defaults are optimized for typical Laravel applications but can be easily customized to suit specific project requirements.
Mix Chainable Methods: Laravel Mix provides chainable methods that allow you to define various asset compilation tasks in a fluent and expressive manner. For example, you can use methods like js(), sass(), less(), postCss(), typescript(), and more to define how different types of assets should be processed and compiled.
Development and Production Environments: Laravel Mix supports different compilation settings for development and production environments. During development, you might want source maps, hot module replacement, and unminified assets for easier debugging. In production, you can enable optimizations like minification, file hashing, and cache busting for improved performance and reduced file sizes.
Laravel Debugbar:
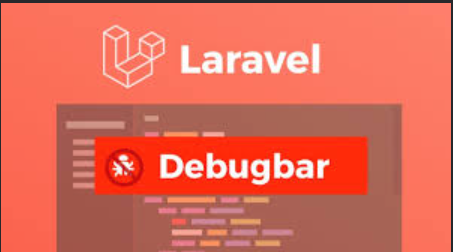
A package that integrates PHP Debug Bar with Laravel, providing detailed information about your application’s performance, queries, routes, and more during development.
Laravel Telescope:
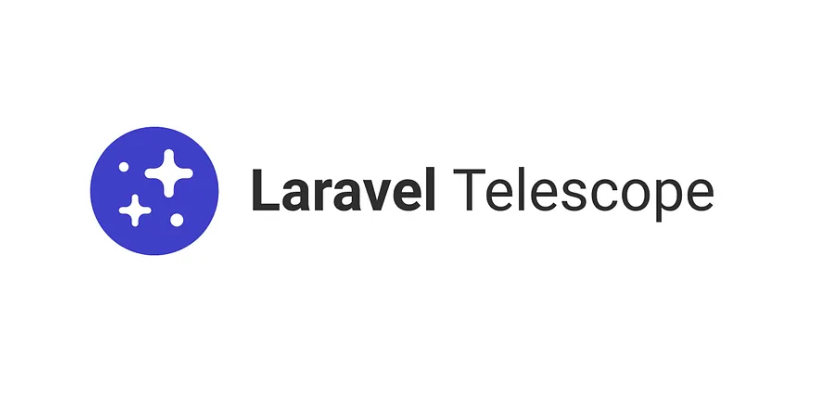
Telescope provides a beautiful and intuitive interface for debugging and monitoring your Laravel application in real-time, allowing you to inspect requests, exceptions, and log entries. Laravel Telescope is a powerful debug assistant and monitoring tool designed specifically for Laravel applications. Developed by the Laravel team, Telescope provides developers with valuable insights into the requests, exceptions, database queries, performance metrics, and more within their Laravel applications.
Request Inspection: Telescope captures detailed information about incoming HTTP requests, including headers, request parameters, route information, response status codes, and more. This allows developers to trace the flow of requests through their application and debug issues related to request handling.
Exception Monitoring: Telescope tracks exceptions and errors that occur during application execution, providing detailed stack traces, error messages, and context information. This helps developers quickly identify and diagnose errors, allowing for more efficient debugging and troubleshooting.
Database Query Monitoring: Telescope records database queries executed by the application, along with their execution time, bindings, and other relevant details. This allows developers to analyze database performance, identify slow queries, and optimize database interactions for better application performance.
Task Scheduling: Telescope provides insights into the execution of scheduled tasks within the application, including task names, schedules, and execution times. This allows developers to monitor and troubleshoot scheduled tasks, ensuring they are running as expected.
Mail and Notification Logging: Telescope logs outgoing emails and notifications generated by the application, providing visibility into email content, recipients, and sending status. This allows developers to verify email functionality and troubleshoot email delivery issues.
Laravel Horizon:
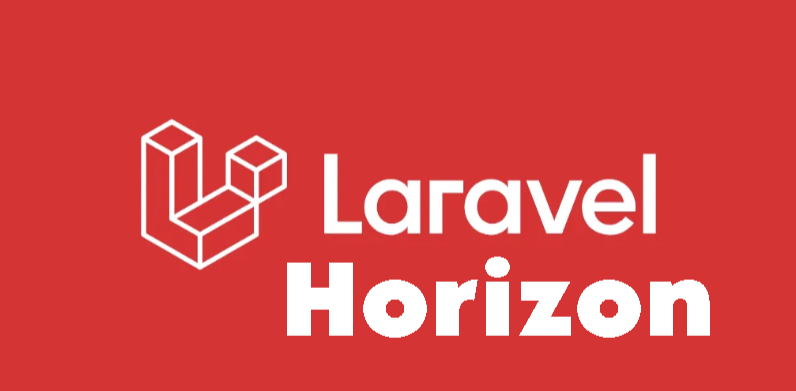
Laravel Horizon is a dashboard and configuration system for managing Laravel Horizon queues, providing insights into job processing, queue workers, and failed jobs. Laravel Horizon is a robust dashboard and configuration system designed to provide a beautiful, real-time view of job queues in Laravel applications. Developed by the Laravel team, Horizon simplifies the monitoring, management, and scaling of queue-based tasks.
Real-Time Monitoring: Horizon provides a real-time dashboard that displays detailed information about your application’s job queues, workers, throughput, and performance metrics. This allows developers and administrators to monitor queue activity and performance in real-time, ensuring optimal operation of background job processing.
Job Metrics and Statistics: Horizon tracks various job metrics and statistics, including job throughput, execution times, failure rates, and queue sizes. These metrics are presented in a visually appealing dashboard, making it easy to identify trends, anomalies, and performance issues.
Worker Management: Horizon allows you to manage your application’s queue workers directly from the dashboard. You can view active workers, pause or resume worker processes, and scale worker instances up or down based on workload and resource availability.
Laravel Nova:
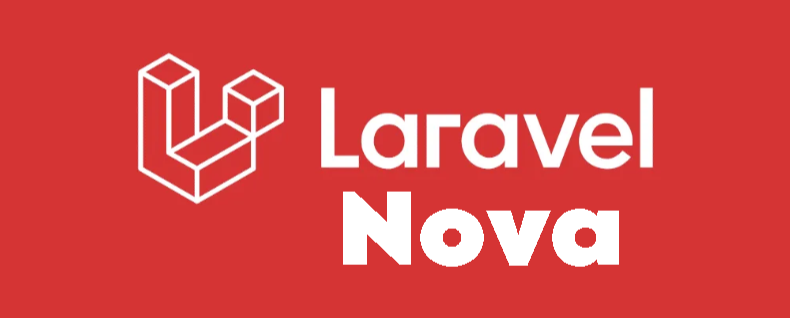
Laravel Nova is a beautifully designed administration dashboard for Laravel applications, allowing you to quickly build custom admin panels with minimal code. Laravel Nova is a beautifully designed administration panel for Laravel applications. Developed by the Laravel team, Nova provides a powerful and customizable dashboard for managing and interacting with your application’s data models, resources, and administrative tasks.
Resource Management: Nova allows you to define resources for your application’s data models, such as users, products, orders, and more. Each resource corresponds to a database table and defines how data should be displayed, edited, and managed within the administration panel.
CRUD Operations: With Nova, you can perform CRUD (Create, Read, Update, Delete) operations on your application’s data directly from the administration panel. Nova provides intuitive interfaces for creating, editing, and deleting records, making it easy to manage your application’s data without writing custom administration interfaces.
Customizable Dashboards: Nova allows you to customize the layout, appearance, and functionality of your administration dashboard to suit your application’s requirements and branding. You can add custom widgets, metrics, charts, and navigation menus to create a personalized and tailored user experience.
Powerful Filters and Search: Nova provides powerful filtering and search capabilities that allow you to quickly find and narrow down records based on specific criteria. You can create custom filters, search fields, and sorting options to streamline data exploration and analysis.
Laravel Sanctum:
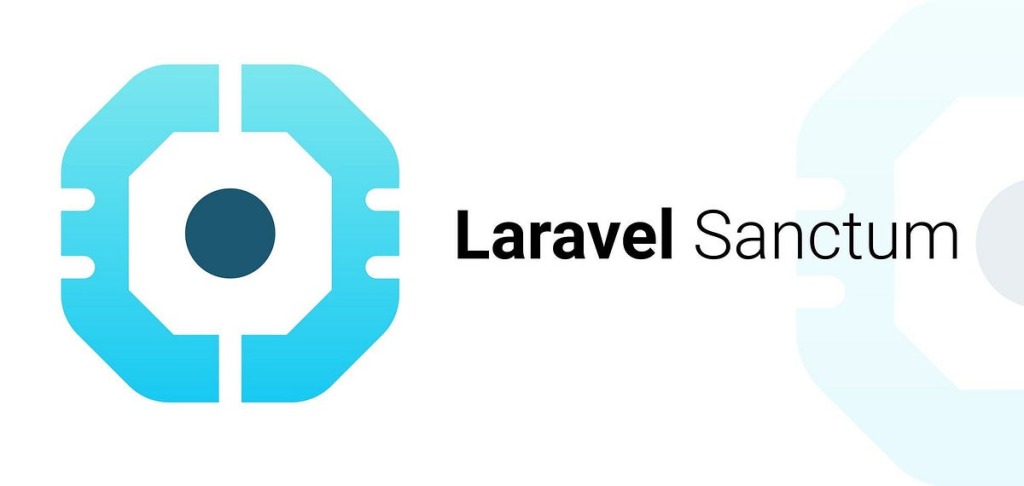
Formerly Laravel Airlock, Sanctum provides a lightweight authentication system for SPAs (Single Page Applications), allowing you to authenticate users via API tokens or Laravel sessions. Laravel Sanctum is a lightweight package for API authentication in Laravel applications. It provides a simple and easy-to-use solution for authenticating users and managing API tokens, enabling secure communication between client-side applications (such as SPAs or mobile apps) and the Laravel backend.
Token Authentication: Sanctum allows you to authenticate users using API tokens, which are cryptographic strings generated by the server and passed by the client with each API request. These tokens serve as credentials to verify the identity of the user and grant access to protected resources.
Stateful and Stateless Tokens: Sanctum supports both stateful and stateless token authentication. Stateful tokens are stored server-side in session cookies, providing a secure and convenient way to authenticate users in traditional web applications. Stateless tokens, on the other hand, are passed via HTTP headers or request parameters, making them ideal for stateless API authentication.
API Token Management: Sanctum provides a set of helper methods and middleware for generating, validating, and managing API tokens in Laravel applications. You can easily generate new tokens for authenticated users, validate tokens on incoming requests, and revoke or delete tokens as needed.
Cross-Origin Resource Sharing (CORS): Sanctum includes middleware for handling Cross-Origin Resource Sharing (CORS) headers, allowing you to configure CORS policies and control access to your API endpoints from different domains or origins.
SPA Authentication: Sanctum is particularly well-suited for authenticating users in Single Page Applications (SPAs) built with frontend frameworks like Vue.js, React, or Angular. It provides a seamless authentication experience for SPA applications, allowing users to log in, register, and access protected resources via API requests.
Laravel Passport:
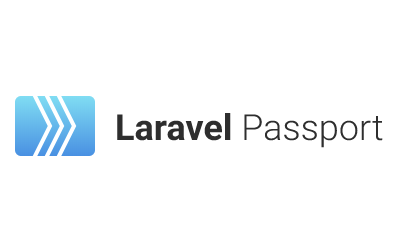
Passport provides a full OAuth2 server implementation for authenticating clients and issuing access tokens, enabling secure API authentication in your Laravel application. Laravel Passport is an official package provided by the Laravel framework for implementing OAuth2 authentication in Laravel applications. OAuth2 is an industry-standard protocol for authorization, commonly used for securing APIs and enabling third-party authentication in web and mobile applications.
OAuth2 Server Implementation: Passport provides a complete OAuth2 server implementation for Laravel applications, allowing you to issue access tokens, manage token scopes, and authenticate clients using various grant types.
API Authentication: Passport enables secure authentication for APIs by issuing access tokens to clients (such as web or mobile applications) after successful authentication. These access tokens are then used to authorize subsequent API requests and grant access to protected resources.
Token Management: Passport includes built-in functionality for generating, validating, and revoking access tokens. You can easily generate new tokens for authenticated users, validate tokens on incoming requests, and revoke or delete tokens as needed.
Token Scopes: Passport supports token scopes, which allow you to define fine-grained permissions and access levels for different types of clients or users. You can create custom scopes to restrict access to specific API endpoints or resources based on user roles or privileges.
Authentication Grant Types: Passport supports multiple OAuth2 grant types, including Authorization Code, Implicit, Password, Client Credentials, and Refresh Token grants. These grant types provide different authentication workflows and security mechanisms for authenticating clients and obtaining access tokens.
Laravel Jetstream:
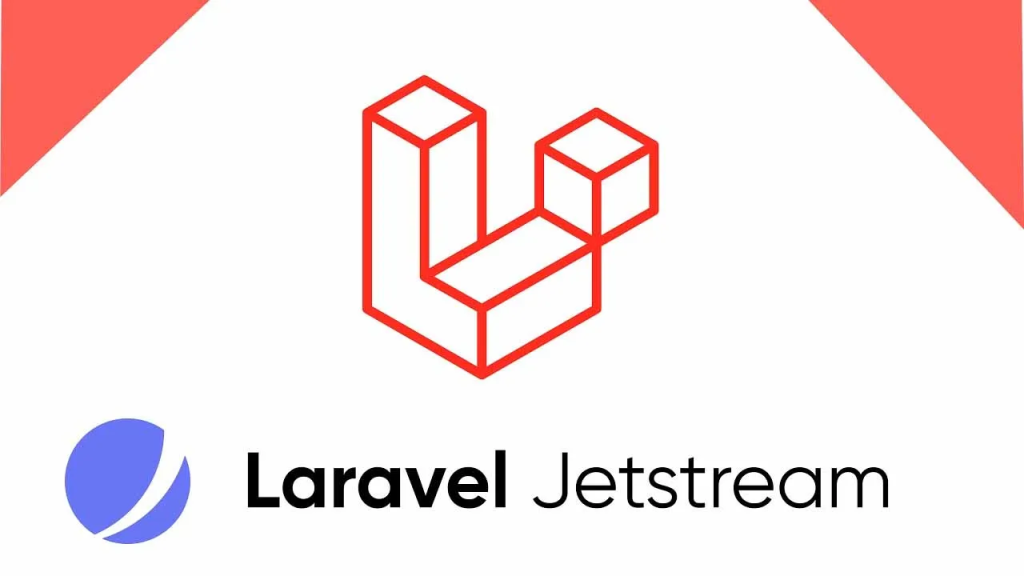
Jetstream is a scaffolding tool for Laravel that provides authentication, teams, and API support out of the box, streamlining the process of building robust web applications. Laravel Jetstream is a robust scaffolding tool for Laravel applications that provides a solid foundation for building modern, feature-rich web applications with minimal effort. Developed by the Laravel team, Jetstream offers pre-built authentication, team management, and API support out of the box, allowing developers to quickly set up and customize common application features without starting from scratch.
Authentication: Jetstream includes a fully featured authentication system with support for user registration, login, email verification, password reset, and two-factor authentication (2FA). It provides customizable views, controllers, and routes for handling authentication workflows, making it easy to integrate user authentication into your Laravel application.
Teams and Collaboration: Jetstream introduces the concept of teams, allowing users to belong to multiple teams and collaborate on projects with team members. It provides features for team management, including team creation, invitation, removal, and role-based permissions, enabling flexible collaboration within your application.
API Support: Jetstream includes built-in support for API authentication and token management, allowing you to authenticate users and issue API tokens for accessing protected resources. It provides a seamless integration with Laravel Sanctum, making it easy to secure API endpoints and interact with your application programmatically.
Laravel Livewire:
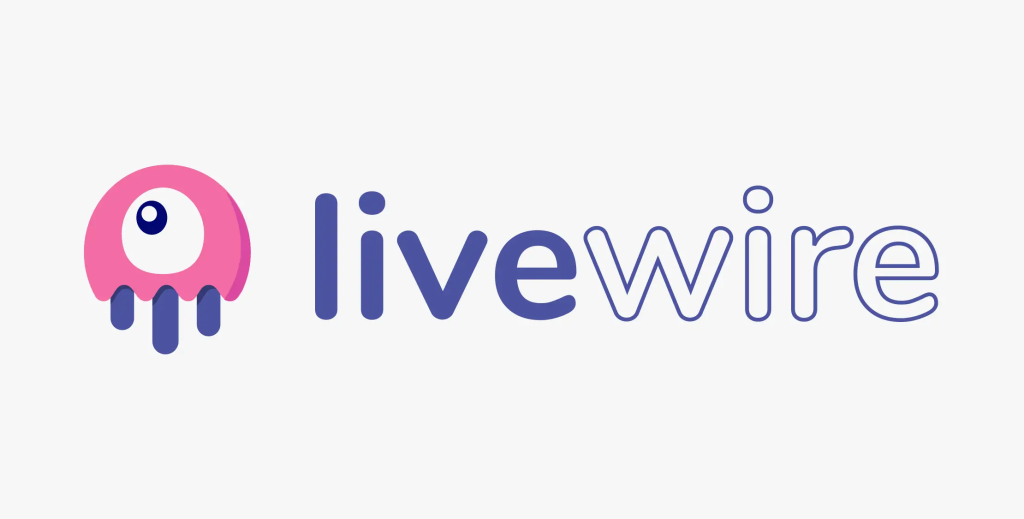
Livewire is a full-stack framework for Laravel that enables dynamic, reactive interfaces using server-side rendering, simplifying the development of interactive UI components. Laravel Livewire is a full-stack framework for Laravel applications that enables you to build interactive and dynamic user interfaces using server-side rendering (SSR). Developed by Caleb Porzio, Livewire allows you to write frontend components in PHP, which are then rendered on the server and updated dynamically without needing to write any JavaScript.
Component-Based Architecture: Livewire organizes UI elements into reusable components, each encapsulating its own logic, markup, and behavior. Components are self-contained units that can be easily composed and reused across different parts of your application, promoting code reusability and maintainability.
Server-Side Rendering: Unlike traditional frontend frameworks, which rely on client-side rendering (CSR) and JavaScript to update the DOM, Livewire performs rendering and data handling on the server. This approach reduces the complexity of managing client-side state and eliminates the need for writing and debugging complex JavaScript code.
Two-Way Data Binding: Livewire provides two-way data binding between frontend components and backend data, allowing you to synchronize UI elements with application state seamlessly. Changes made to form inputs, checkboxes, selects, and other UI elements are automatically propagated to the backend, and vice versa, without requiring manual event handling or AJAX requests.
Lifecycle Hooks: Livewire components support lifecycle hooks, which allow you to define custom logic that runs at specific stages of a component’s lifecycle. You can use lifecycle hooks to perform initialization, validation, data fetching, and cleanup tasks, providing fine-grained control over component behavior and rendering.
Laravel Nova Resource Tool: A tool for creating custom Nova resources, allowing you to define custom fields, actions, and filters for your Laravel Nova admin panel. Laravel Nova Resource Tool is a feature of Laravel Nova, a beautifully designed administration panel for Laravel applications. Nova Resource Tools allow developers to customize and extend the functionality of Nova by creating custom tools for managing and interacting with resources within the administration panel.
Custom Tools: Laravel Nova provides a set of built-in tools for managing resources such as users, posts, products, etc. However, Nova Resource Tools allow developers to create their own custom tools tailored to the specific needs of their application.
Resource Management: Nova Resource Tools typically focus on managing specific types of resources within the administration panel. For example, you might create a custom tool for managing blog posts, customer orders, inventory items, or any other type of data stored in your application’s database.
CRUD Operations: Resource Tools in Nova typically include features for performing CRUD (Create, Read, Update, Delete) operations on the associated resource. This allows administrators and content managers to add, view, edit, and delete resource records directly from the Nova dashboard.
Custom Views and Actions: Nova Resource Tools allow developers to define custom views, actions, and workflows for interacting with resource data. You can create custom views to display data in different formats (e.g., tables, cards, charts), as well as define custom actions (e.g., bulk actions, export options) for managing resource records.