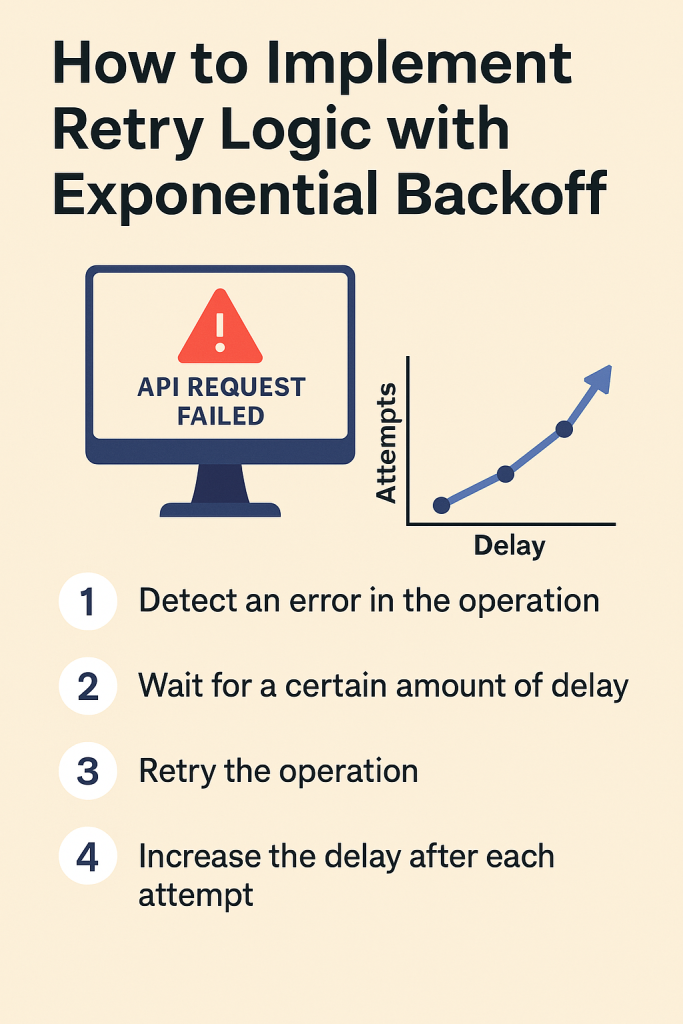
In modern web applications, API calls and network requests play a crucial role in retrieving data or interacting with external services. However, one common challenge developers face when making these requests is handling failures gracefully. Transient errors like network timeouts, rate-limiting, or temporary server unavailability can disrupt the normal operation of an application.
One effective strategy for mitigating these issues is Retry Logic with Exponential Backoff. This technique is particularly useful for handling rate-limited APIs and temporary network failures. In this blog, we will explore how to implement Retry Logic with Exponential Backoff, why it’s necessary, and how to implement it in PHP using Guzzle.
What is Retry Logic with Exponential Backoff?
Retry Logic:
Retry Logic is a method where a system or service attempts to re-execute a failed request after an initial failure. Instead of giving up immediately, the system retries the operation a few times before returning an error.
Exponential Backoff:
Exponential Backoff is a strategy in which the system doesn’t retry immediately. Instead, it waits for an increasing amount of time after each failure. The time between retries increases exponentially (e.g., 2 seconds, 4 seconds, 8 seconds, etc.). This helps reduce the load on a service and avoids hammering the server with repeated requests.
Why Use Exponential Backoff?
- Prevents Server Overload: By gradually increasing the wait time between retries, exponential backoff prevents the system from overwhelming the server with rapid-fire requests.
- Improves Reliability: It increases the chances of a successful request if the failure is temporary, like a server overload or a network glitch.
- Rate-Limit Handling: Many APIs impose rate limits on requests. When these limits are exceeded (e.g., 429 Too Many Requests), exponential backoff can prevent immediate retries and give the server time to reset its rate limit.
How to Implement Retry Logic with Exponential Backoff in PHP?
Example Scenario: API Requests in PHP with Guzzle
Let’s walk through a practical example. Imagine you are making a request to an API using the Guzzle HTTP Client in PHP. The API might sometimes fail due to transient issues, such as:
- Rate-limiting (HTTP status 429)
- Network issues
- Server errors (HTTP status 500)
We can implement retry logic to handle these failures gracefully, using exponential backoff to space out the retries.
Step-by-Step Guide
1. Setting Up Guzzle HTTP Client
First, make sure you have Guzzle installed. If you don’t have it installed, you can do so using Composer:
composer require guzzlehttp/guzzle
2. Define the Retry Logic with Exponential Backoff
Here’s how you can write a PHP function that implements retry logic with exponential backoff.
use GuzzleHttp\Client;
use GuzzleHttp\Exception\RequestException;
public static function getApiData($url) {
$retries = 5; // Number of retries
$delay = 2; // Initial delay in seconds
while ($retries > 0) {
try {
// Create a Guzzle client and make the request
$client = new Client();
$response = $client->get($url);
// If the request is successful, return the response
return json_decode($response->getBody()->getContents(), true);
} catch (RequestException $e) {
// If rate-limiting (429), retry with backoff
if ($e->getCode() == 429) {
// Log retry attempt
echo "Rate limit exceeded. Retrying in $delay seconds...\n";
// Decrement retries and increase delay (exponential backoff)
$retries--;
sleep($delay);
$delay *= 2; // Double the delay for next retry
} else {
// If other errors occur, log and return an error message
echo "Error: " . $e->getMessage() . "\n";
return ['error' => 'Request failed. Please try again later.'];
}
}
}
// If all retries fail, return an error message
return ['error' => 'Too many failed attempts. Please try again later.'];
}
Breakdown of the Code:
- Initial Setup: We start with a maximum of 5 retries (
$retries = 5
) and an initial delay of 2 seconds ($delay = 2
). - API Request: Inside the
try
block, we use the Guzzle client to make an API request. If the request is successful, the response is returned. - Exception Handling: If an exception occurs (e.g., network failure or rate-limiting error):
- Rate-Limiting (
429 Too Many Requests
): If the error code is429
, we log the retry attempt and wait for a specified delay. After each retry, the delay is doubled (exponential backoff). - Other Errors: For any other exceptions, we log the error and return a failure response.
- Rate-Limiting (
- Final Fallback: If all retries fail, we return a message indicating that too many failed attempts have been made.
3. Testing the Function
Now, you can test the getApiData
function by calling it with a URL:
$url = "https://api.example.com/data";
$response = getApiData($url);
if (isset($response['error'])) {
echo $response['error'];
} else {
echo "Data fetched successfully:\n";
print_r($response);
}
Additional Considerations:
- Handling Other Transient Errors: You can enhance this by adding more error codes like
502 Bad Gateway
or503 Service Unavailable
, which also indicate transient issues. - Logging Rate Limits: If the API provides rate limit headers (like
X-RateLimit-Remaining
), you can log these to help decide when to retry. For example: - Handling Timeouts: Network timeouts (e.g.,
30 seconds timeout
) can also be handled by retrying. You can configure Guzzle with a timeout:
$remaining = $response->getHeaderLine('X-RateLimit-Remaining');
$resetTime = $response->getHeaderLine('X-RateLimit-Reset');
echo "Rate limit remaining: $remaining, reset time: $resetTime\n";
$response = $client->get($url, ['timeout' => 30]);