Becoming a Laravel expert requires a structured learning path and consistent practice. Below is a roadmap that outlines the essential skills and topics you should learn to become proficient in Laravel:
1. Basics of PHP:
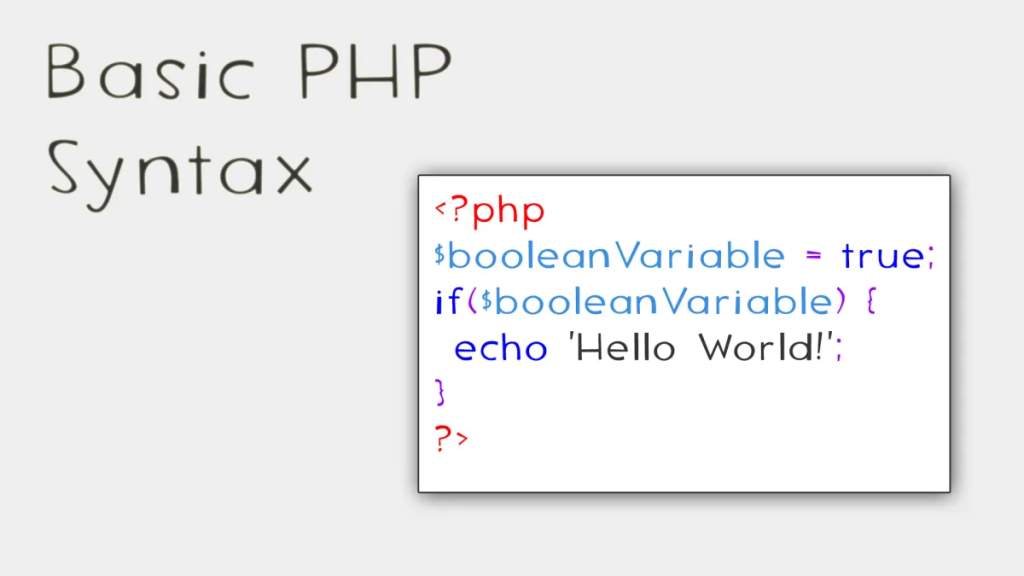
- Syntax and Variables: Understand PHP syntax, data types, and variables.
- Control Structures: Learn about loops, conditional statements, and switch cases.
- Functions: Understand how to define and use functions in PHP.
2. Object-Oriented Programming (OOP):
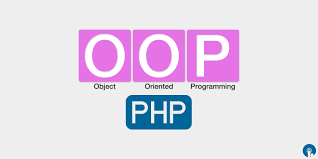
- Classes and Objects: Learn about classes, objects, properties, and methods.
- Inheritance and Polymorphism: Understand inheritance, method overriding, and polymorphism.
- Encapsulation and Abstraction: Learn about encapsulation and abstraction principles in OOP.
3. Composer:
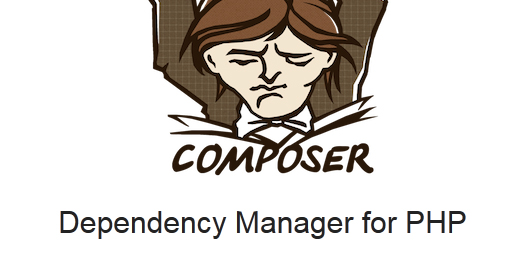
- Dependency Management: Learn how to use Composer for managing dependencies and autoloading classes.
- Installing Laravel: Understand how to install Laravel using Composer.
4. Laravel Fundamentals:
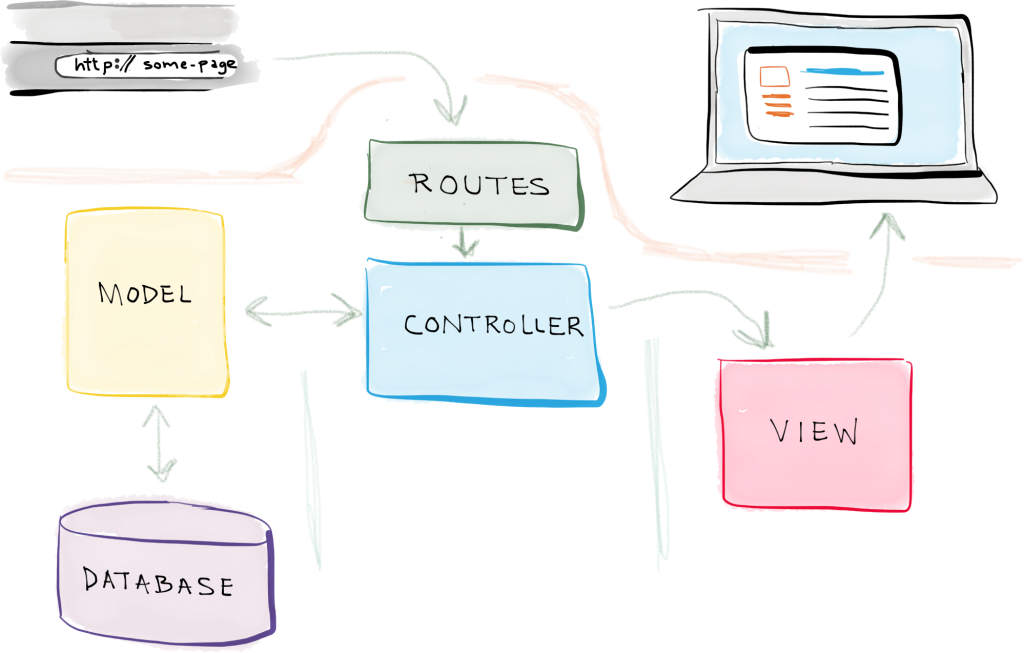
- Routing: Learn about routing in Laravel and how to define routes for your application.
- Controllers: Understand how controllers work and how to create and use them in Laravel.
- Views: Learn about Blade templating engine and how to create views in Laravel.
- Models: Understand Eloquent ORM and how to define and use models in Laravel.
5. Database Management:
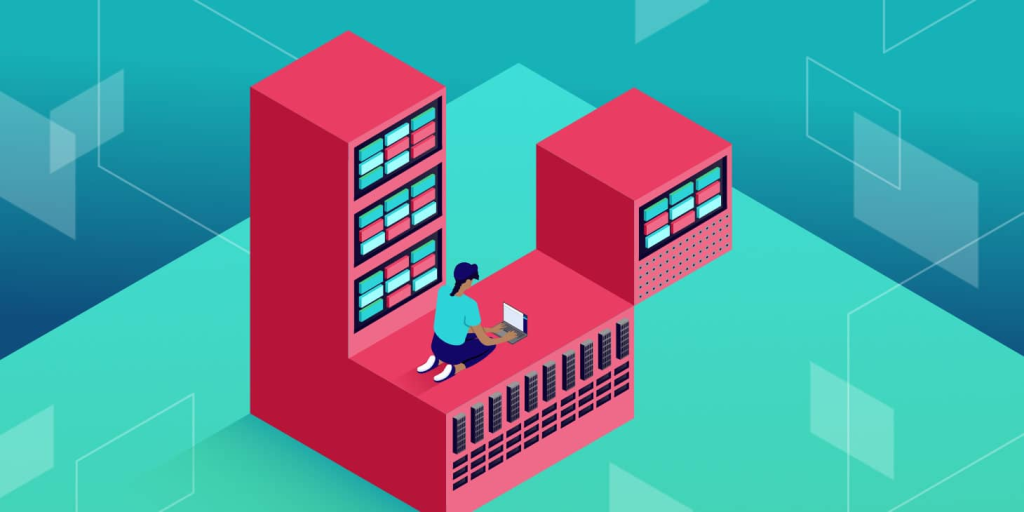
- Database Configuration: Learn how to configure database connections in Laravel.
- Migrations: Understand how to use migrations to manage database schema.
- Seeding: Learn about database seeding for populating the database with sample data.
- Query Builder and Eloquent: Master Laravel’s query builder and Eloquent ORM for database interactions.
6. Authentication and Authorization:
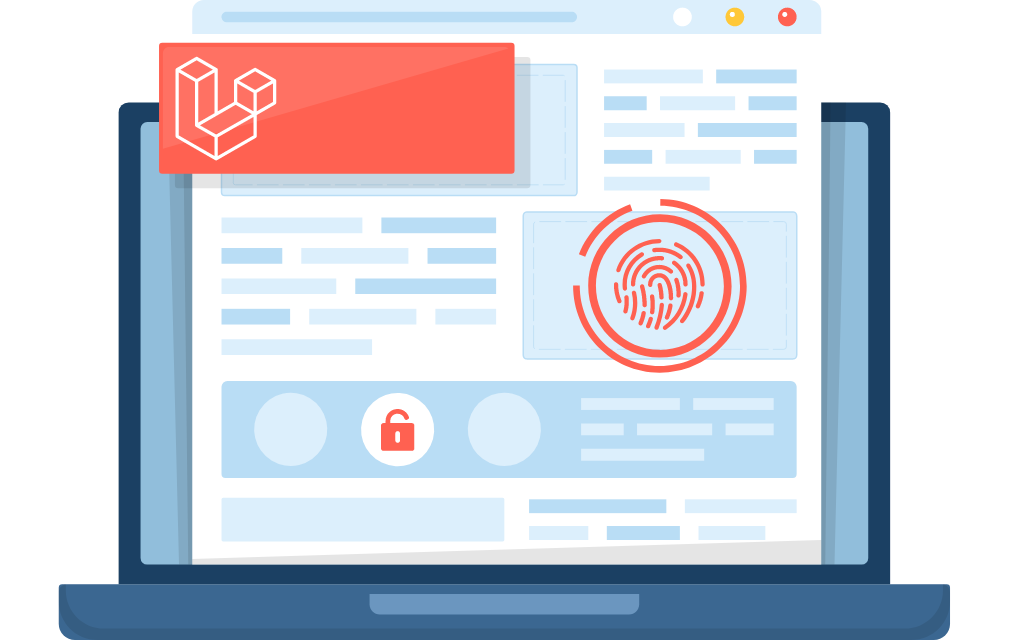
- Authentication: Learn how to implement user authentication using Laravel’s built-in authentication system.
- Authorization: Understand how to implement role-based access control (RBAC) and permissions.
7. Middleware and Requests:
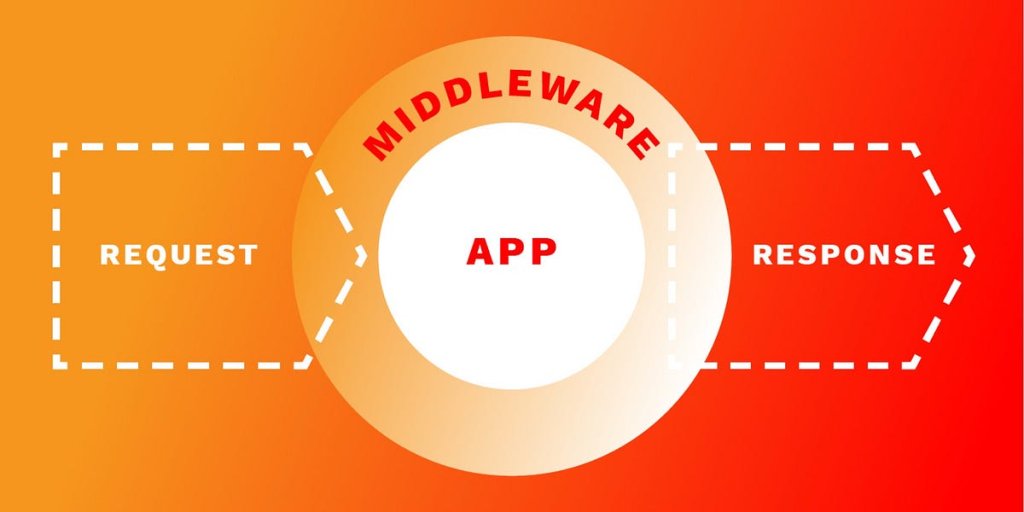
- Middleware: Learn about middleware and how to use it to filter HTTP requests.
- Request Handling: Understand how to handle form submissions, file uploads, and validation.
8. RESTful API Development:
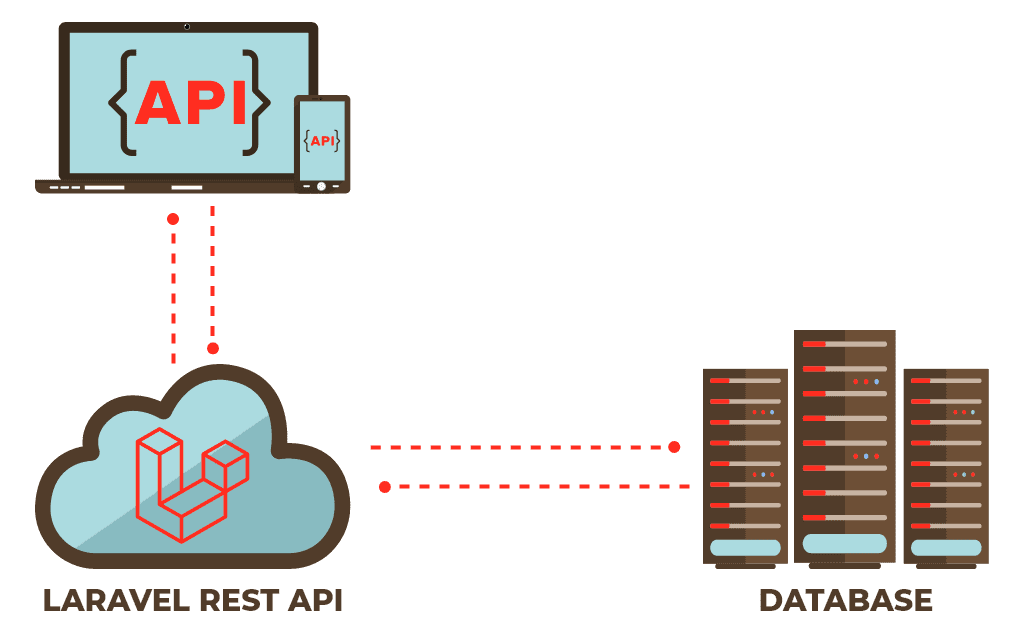
- API Routes: Learn how to define API routes in Laravel.
- Resource Controllers: Understand how to create resource controllers for API endpoints.
- API Authentication: Implement authentication mechanisms for APIs using tokens or OAuth.
9. Testing:
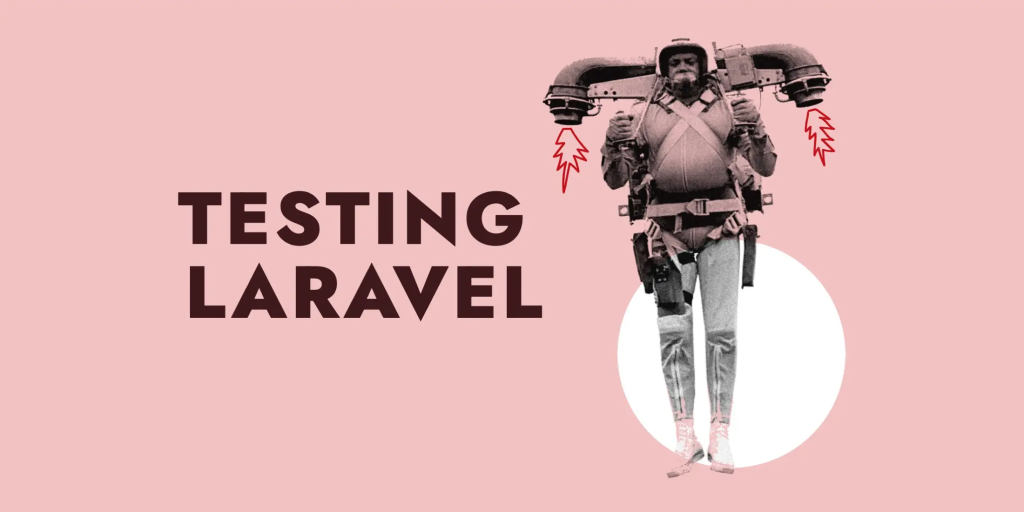
- Unit Testing: Learn about PHPUnit and how to write unit tests for your Laravel applications.
- Feature Testing: Understand how to write feature tests to test application functionality.
10. Performance Optimization and Security:
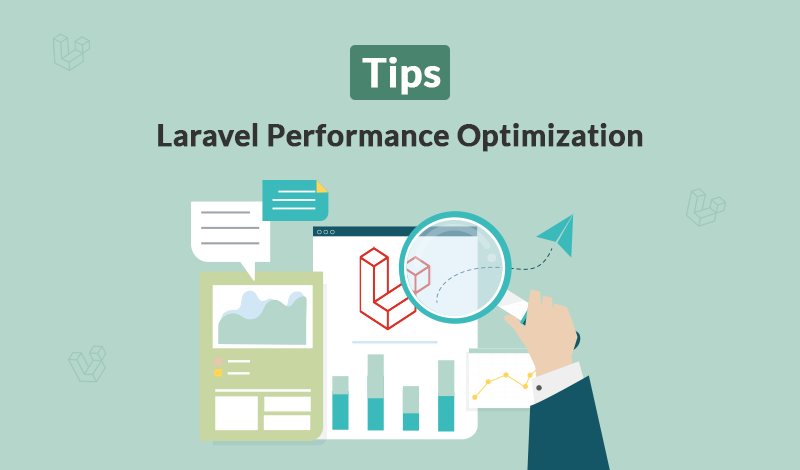
- Caching: Learn how to use caching mechanisms to improve performance.
- Security Best Practices: Understand Laravel security features and best practices for securing your applications.
11. Advanced Topics:
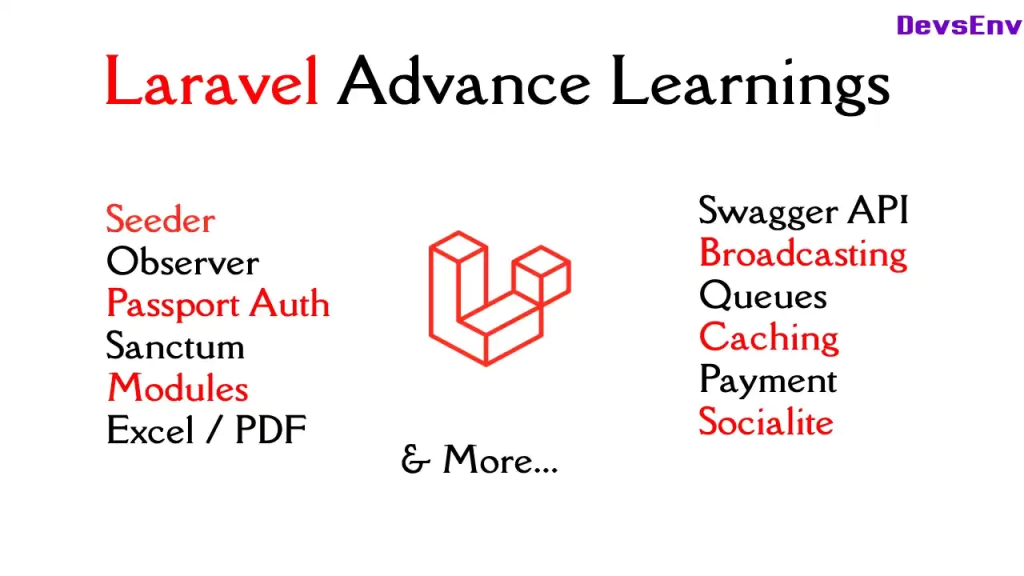
- Queueing and Job Processing: Learn about Laravel’s queue system and how to process background jobs.
- Event Broadcasting: Understand how to implement real-time communication using Laravel’s event broadcasting feature.
- Task Scheduling: Learn how to schedule tasks and commands using Laravel’s scheduler.
12. Continuous Learning and Community Involvement:
- Stay Updated: Keep up with Laravel’s latest releases, features, and best practices by reading documentation and following Laravel-related blogs and forums.
- Contribute to Open Source: Contribute to Laravel’s ecosystem by submitting bug fixes, documentation improvements, or new features to Laravel’s GitHub repositories.