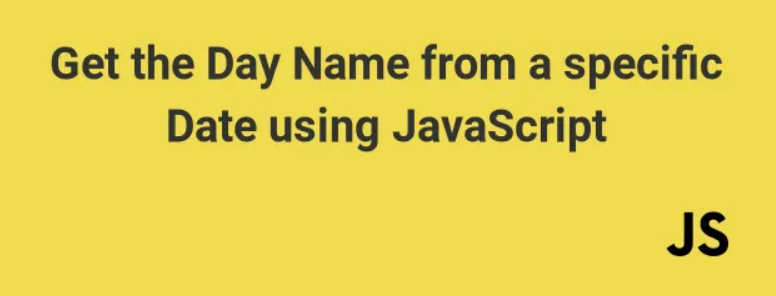
JavaScript, one of the core technologies of web development, is a versatile language that can perform a wide range of tasks, including handling date and time operations. One common requirement in web applications is to determine the day of the week (e.g., Monday, Tuesday, etc.) for a given date. Here we use JavaScript to find the day name from a specific date, whether it’s for displaying events, scheduling tasks, or creating dynamic calendars.
JavaScript’s Date Object
JavaScript provides a built-in Date
object that makes working with dates and times relatively straightforward. To find the day name from a specific date, follow these steps:
1. Create a Date Object
To start, create a Date
object and provide the specific date you want to work with. You can provide the date in various formats, including ISO 8601, timestamp, or as separate year, month, and day values.
Here’s an example using ISO 8601 format (YYYY-MM-DD)
const date = new Date('2023-10-01');
2. Get the Day of the Week
JavaScript’s Date
object has a method called getDay()
that returns the day of the week as an integer, where Sunday is 0, Monday is 1, and so on, up to Saturday as 6.
To get the day name, you can create an array of day names and use the getDay()
result as an index to retrieve the corresponding day name.
const daysOfWeek = ['Sunday', 'Monday', 'Tuesday', 'Wednesday', 'Thursday', 'Friday', 'Saturday'];
const dayName = daysOfWeek[date.getDay()];
Putting It All Together
Here’s the complete JavaScript code to find the day name from a specific date.
function getDayNameFromDate(inputDate) {
const date = new Date(inputDate);
const daysOfWeek = ['Sunday', 'Monday', 'Tuesday', 'Wednesday', 'Thursday', 'Friday', 'Saturday'];
const dayName = daysOfWeek[date.getDay()];
return dayName;
}
// Example usage:
const dateToCheck = '2023-10-01';
const dayName = getDayNameFromDate(dateToCheck);
console.log(`The day on ${dateToCheck} is ${dayName}.`);
The getDayNameFromDate
function takes an input date, creates a Date
object, and returns the corresponding day name.