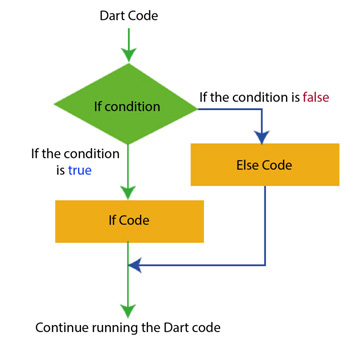
The if-else statement is a fundamental construct in programming that allows you to execute different blocks of code based on specific conditions. In Flutter, if-else statements are used extensively to control the flow of your application’s logic and user interface.
Syntax of If-Else Statements in Dart (Flutter’s Programming Language):
The syntax of an if-else statement in Dart is as follows.
if (condition) {
// Code to execute if the condition is true
} else {
// Code to execute if the condition is false
}
In this syntax:
condition
is a boolean expression that evaluates to either true or false.- The code block inside the
if
statement is executed if the condition is true. - The code block inside the
else
statement is executed if the condition is false.
Example: Using If-Else Statements in Flutter Widgets:
Let’s consider a simple example where we want to display a message based on whether a user is logged in or not. We can achieve this using if-else statements in Flutter widgets.
import 'package:flutter/material.dart';
class HomePage extends StatelessWidget {
final bool isLoggedIn = true;
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('Conditional Statements in Flutter'),
),
body: Center(
child: isLoggedIn
? Text('Welcome, User!')
: Text('Please log in to continue.'),
),
);
}
}
void main() {
runApp(MaterialApp(
home: HomePage(),
));
}
We define a boolean variable isLoggedIn
that determines whether the user is logged in.
Inside the build
method of the HomePage
widget, we use an if-else statement to conditionally display different messages based on the value of isLoggedIn
.
If isLoggedIn
is true, we display a welcome message. Otherwise, we prompt the user to log in.