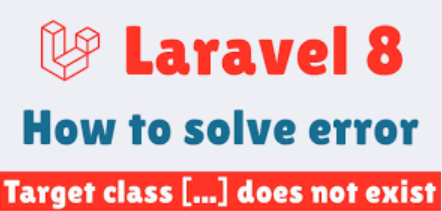
The error message “Target class [UserController] does not exist” indicates that Laravel cannot locate the specified controller class when attempting to route a request.
In my case:
// UserController.php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
class UserController extends Controller
{
public function index(){
return "hello world";
}
}
In the UserController, we have defined an index method that returns a simple string response.
// web.php
use App\Http\Controllers\UserController;
Route::get('/users', 'UserController@index');
In the routes/web.php file, we have defined a route to handle requests to “/users” by invoking the index method of the UserController.
Solution: To resolve the “Target class does not exist” error, we need to ensure that Laravel can locate the UserController class.
Solution 1: Using PHP Callable Syntax
// web.php
use App\Http\Controllers\UserController;
Route::get('/users', [UserController::class, 'index']);
This approach specifies the UserController class using PHP callable syntax, ensuring that Laravel can locate the class without any issues.
Solution 2: Using String Syntax
// web.php
Route::get('/users', 'App\Http\Controllers\UserController@index');