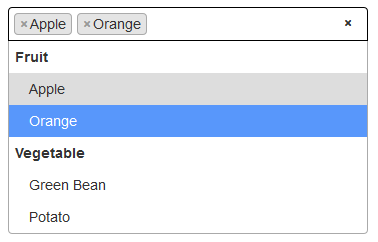
Select2 is a powerful jQuery plugin that enhances the functionality of input fields by providing an intuitive dropdown selection experience. In this blog post, we will explore how to integrate Select2 with jQuery to create dynamic and feature-rich input fields with ease.
To begin, include the Select2 CSS and JavaScript files in your HTML document. You can either host these files locally or use a CDN (Content Delivery Network) for convenience. Here’s an example using the CDN approach.
Create the Input Field:
In your HTML document, create an input field that you want to enhance with Select2. Assign it a unique id
or a specific class
for targeting it in your jQuery code. For instance.
Initialize Select2 in jQuery:
In your jQuery code, select the input field using its id
or class
and call the select2()
method on it. You can customize the behavior and appearance of Select2 by passing an object with various options to the select2()
method.
Ensure that the $(document).ready()
function wraps your jQuery code to ensure it runs when the document is ready.
Customize Select2 Options: Select2 provides a wide range of options to tailor its behavior and appearance according to your needs. You can pass an object with these options to the select2()
method. Let’s customize the placeholder text as an example:
Feel free to explore the Select2 documentation for more options and advanced customization possibilities.
Step 5: Handle Select2 Events: Select2 also offers event handlers to manage user interactions, such as selecting an option or opening/closing the dropdown. You can attach event handlers to the Select2 instance to perform actions when these events occur.
Here’s an example:
$(document).ready(function() { $(‘#myInputField’).select2({ // Options for Select2 }).on(‘select2:select’, function(event) { // Handle the select event var selectedOption = event.params.data; console.log(‘Selected:’, selectedOption); }); });In this example, the select2:select
event handler is attached to the Select2 instance to handle the select
event. You can replace select2:select
with other available event names based on your requirements.