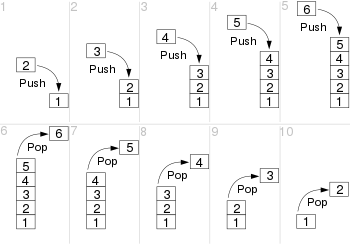
In programming, “push” and “pop” are operations commonly associated with the manipulation of data in a data structure called an array. Arrays are used to store collections of elements of the same type.
Push: The “push” operation adds an element to the end of an array, increasing its size by one. The new element is inserted at the last position, shifting any existing elements to accommodate the new one.
Example:
let numbers = [1, 2, 3]; console.log(numbers); // Output: [1, 2, 3] numbers.push(4); console.log(numbers); // Output: [1, 2, 3, 4]In this example, the push
method is used to add the number 4 to the end of the numbers
array. After the push operation, the array contains [1, 2, 3, 4].
- Pop: The “pop” operation removes and returns the last element from an array, reducing its size by one. The element that is popped is permanently removed from the array.
In this example, the pop
method is used to remove the last element (4) from the numbers
array. The popped element is stored in the variable poppedElement
. After the pop operation, the array contains [1, 2, 3], and the popped element is 4.