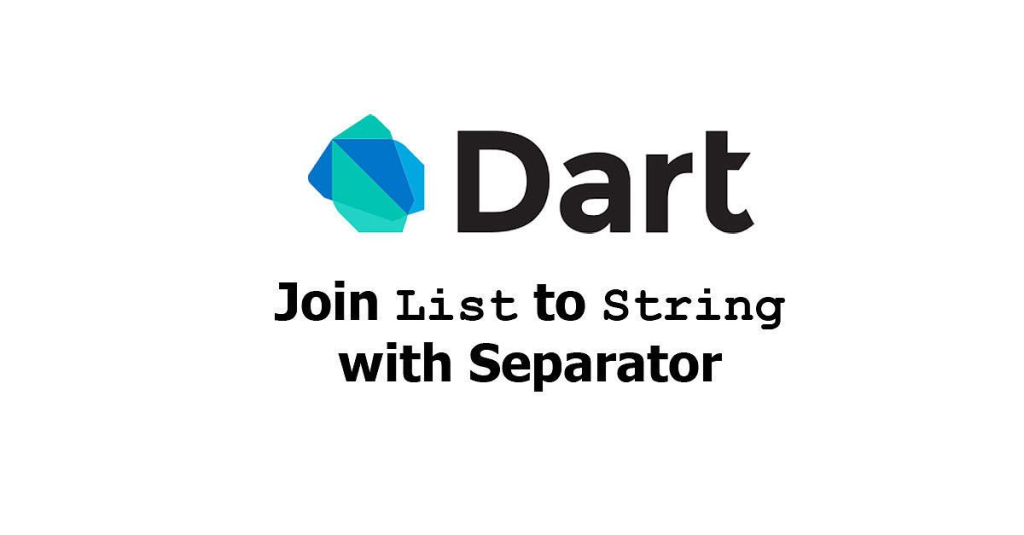
Consider you have a list of languages selected by the user, and you want to display them in a formatted string with commas and spaces between each language. For example, if the user selects “English,” “Spanish,” and “French,” you want to display it as “English, Spanish, French” rather than “EnglishSpanishFrench.”
The Solution
Flutter provides a simple and elegant solution to achieve this using the join
method available on lists. The join
method concatenates all the elements of a list into a single string, with an optional separator between each element. By using a comma and a space as the separator, we can achieve the desired formatting.
Implementation
Here’s how you can implement this in Flutter
List<String> selectedLanguages = ['English', 'Spanish', 'French'];
String formattedLanguages = selectedLanguages.join(', ');
print(formattedLanguages); // Output: English, Spanish, French
- We define a list of strings
selectedLanguages
containing the languages selected by the user. - We use the
join
method to concatenate the elements of the list into a single string, with a comma and a space,
as the separator. - The resulting string
formattedLanguages
contains the formatted list of languages.
Usage in Flutter Widgets
You can easily use the formattedLanguages
string in your Flutter widgets to display the list of selected languages to the user. Here’s an example of how you can integrate it into a Text
widget.
Text(
'Selected Languages: $formattedLanguages',
style: TextStyle(fontSize: 16),
),
Formatting lists of data with commas and spaces in Flutter is a straightforward task thanks to the join
method available on lists. By utilizing this method, you can easily create visually appealing and human-readable representations of lists for your Flutter applications.