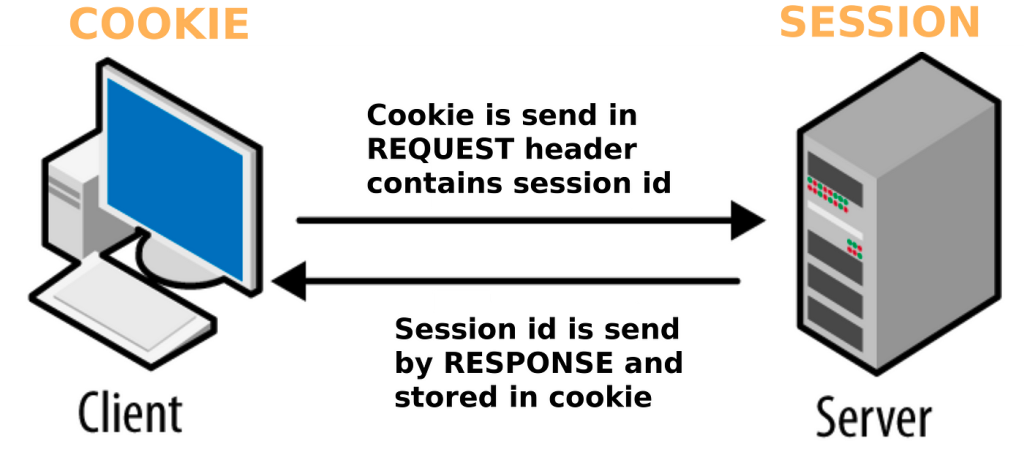
Session cookies are an essential component of any web application, and Laravel provides developers with a simple and effective way to manage them. Laravel’s session cookies are used to store data across multiple requests, allowing developers to maintain user-specific information as users navigate through their web applications.
One of the significant advantages of using session cookies in Laravel is that they provide a way to manage user authentication. Laravel’s session cookies are used to store user credentials, allowing users to stay logged in even when they navigate away from the website or close their browsers. Additionally, session cookies are used to store temporary data, such as shopping cart information, that needs to be preserved across multiple requests.
To set up session cookies in Laravel, developers can use the built-in session
middleware. This middleware handles the creation, retrieval, and storage of session data, making it easy to manage sessions throughout the application. Additionally, Laravel provides developers with the ability to configure session storage, allowing them to store session data in a database, file system, or another external storage provider.
To configure session storage in Laravel, developers can update the config/session.php
file. This file contains configuration options for various session drivers, including file, cookie, database, and Redis. Developers can choose the driver that best fits their needs and configure it according to their application’s requirements.
In conclusion, session cookies are an essential part of any web application, and Laravel provides developers with a robust and straightforward way to manage them. By leveraging Laravel’s built-in session middleware, developers can easily store and retrieve session data, allowing them to create feature-rich and user-friendly web applications.
laravel cookies get, set, delete, using the request()
and response()
helper functions.
Laravel is a popular PHP web application framework that provides a simple and elegant syntax for developers to build robust web applications. One of the important features of Laravel is its ability to handle cookies. Cookies are small pieces of data that are stored on the client’s computer and are used to identify a particular user. In this blog, we will discuss how to get, set, and delete cookies in Laravel with examples.
Getting a Cookie in Laravel To get a cookie in Laravel, you can use the request()
helper function. The request()
function returns an instance of the Illuminate\Http\Request
class, which provides several methods to work with cookies.
For example, let’s say we have a cookie named username
, and we want to retrieve its value. Here’s how we can do it:
In the above code, we are using the cookie()
method to retrieve the value of the username
cookie.
Setting a Cookie in Laravel To set a cookie in Laravel, you can use the cookie()
method provided by the Illuminate\Http\Response
class. This method takes three arguments: the name of the cookie, the value of the cookie, and the number of minutes until the cookie expires.
For example, let’s say we want to set a cookie named username
with a value of John Doe
, and we want the cookie to expire in 60 minutes. Here’s how we can do it:
In the above code, we are creating a new instance of the Illuminate\Http\Response
class and using its withCookie()
method to set the username
cookie.
Deleting a Cookie in Laravel To delete a cookie in Laravel, you can use the forget()
method provided by the Illuminate\Http\Response
class. This method takes the name of the cookie as its argument.
For example, let’s say we want to delete the username
cookie. Here’s how we can do it:
In the above code, we are using the forget()
method to delete the username
cookie.