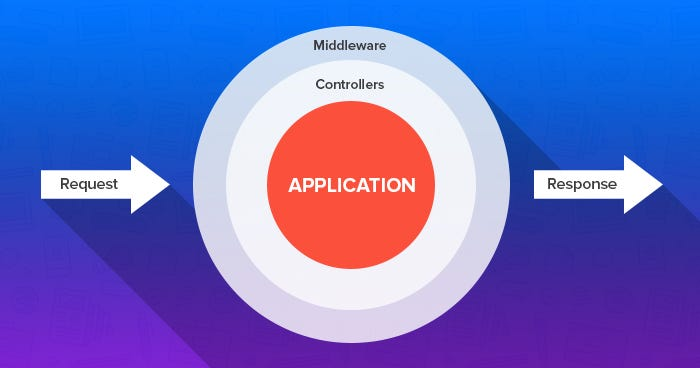
What is Middleware?
Middleware in Laravel is a layer that intercepts and processes HTTP requests entering your application. It acts as a bridge between the client and the application’s core logic, allowing developers to filter, modify, or enhance requests before they reach the intended route handler. Essentially, middleware enables you to inject custom functionality at different points in the HTTP request lifecycle. Laravel Middleware empowers developers to exert fine-grained control over the flow of HTTP requests, enhancing security, modularity, and customization in web applications. Whether leveraging built-in middleware or crafting custom solutions, understanding the role of middleware is fundamental to mastering Laravel development.
Why is Middleware Important for Laravel?
- Request Processing: Middleware plays a crucial role in processing HTTP requests before they reach the application’s core logic. It allows developers to customize, validate, or manipulate incoming data.
- Modularity and Reusability: By breaking down functionality into middleware, developers can create modular and reusable components. This promotes cleaner code architecture and easier maintenance.
- Security: Middleware enhances the security of Laravel applications by providing a convenient way to implement authentication, authorization, and other security-related features.
- Global and Route-Specific Actions: Laravel middleware can be applied globally to all routes or selectively to specific routes, providing fine-grained control over the behavior of the application.
The Use of Middleware:
How is Middleware Used in Laravel?
- Registration:
- Middleware is registered in the
app/Http/Kernel.php
file. Theweb
middleware group, for example, includes middleware responsible for handling cookies, sessions, and CSRF protection.
- Middleware is registered in the
protected $middlewareGroups = [
'web' => [
\App\Http\Middleware\EncryptCookies::class,
\Illuminate\Cookie\Middleware\AddQueuedCookiesToResponse::class,
// ... other middleware
],
];
Global Middleware:
- Global middleware is applied to every HTTP request handled by your application. Examples include logging, CORS handling, or language localization.
protected $middleware = [
// ... other middleware
\App\Http\Middleware\ExampleMiddleware::class,
];
Route Middleware:
- Middleware can be assigned to specific routes, allowing for route-specific processing. This is useful for actions such as user authentication.
Route::get('/dashboard', function () {
// ... route logic
})->middleware('auth');
Types of Middleware in Laravel:
1. Authentication Middleware:
- Purpose: Ensures that a user is authenticated before allowing access to certain routes.
- Usage: Automatically applied to routes using the
auth
middleware.
2. CORS Middleware:
- Purpose: Handles Cross-Origin Resource Sharing to control access to resources from different domains.
- Usage: Applied globally or to specific routes to manage cross-origin requests.
3. Logging Middleware:
- Purpose: Logs information about incoming requests, providing insights into application activity.
- Usage: Added to the global middleware stack for comprehensive request logging.
4. Throttle Requests Middleware:
- Purpose: Prevents abuse or potential attacks by limiting the number of requests a user can make in a specified time period.
- Usage: Applied globally or to specific routes to control request rates.
Why Create Custom Middleware?
- Specific Business Logic:
- When you have specific business logic that needs to be applied to multiple routes or controllers, creating custom middleware provides a clean and reusable solution.
- Intercepting Requests:
- Custom middleware allows you to intercept and modify requests before they reach the route handler, enabling customization based on project requirements.
Steps to Create Custom Middleware:
- Generate Middleware:
- Use the Artisan command to generate a new middleware class.
php artisan make:middleware CustomMiddleware
Define Middleware Logic:
- Open the generated middleware file (
CustomMiddleware.php
) and implement the desired logic in thehandle
method.
public function handle($request, Closure $next)
{
// Custom logic before the request reaches the route handler
$response = $next($request);
// Custom logic after the route handler has processed the request
return $response;
}
Register Middleware:
- Register your custom middleware in the
app/Http/Kernel.php
file.
protected $middleware = [
// ... other middleware
\App\Http\Middleware\CustomMiddleware::class,
];
Apply Middleware to Routes:
- Apply your custom middleware to specific routes in the
web.php
orapi.php
route file.
Route::get('/example', 'ExampleController@index')->middleware('custom');