Create a New Flutter Project
To create a new Flutter project, run the following command in the terminal.
flutter create hello_world_app
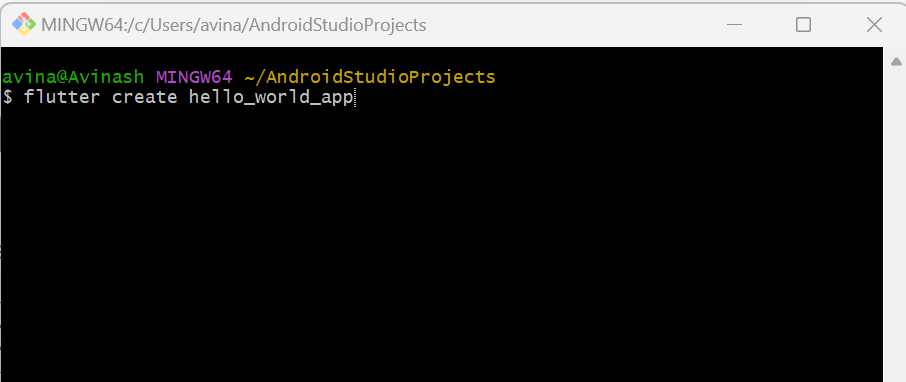
This command creates a new directory named “hello_world_app” with the necessary files and folder structure for a Flutter project.
Depending on your chosen IDE (Android Studio or Visual Studio Code), open the “hello_world_app” directory as a Flutter project. Ensure that you have an emulator set up, Open your emulator.
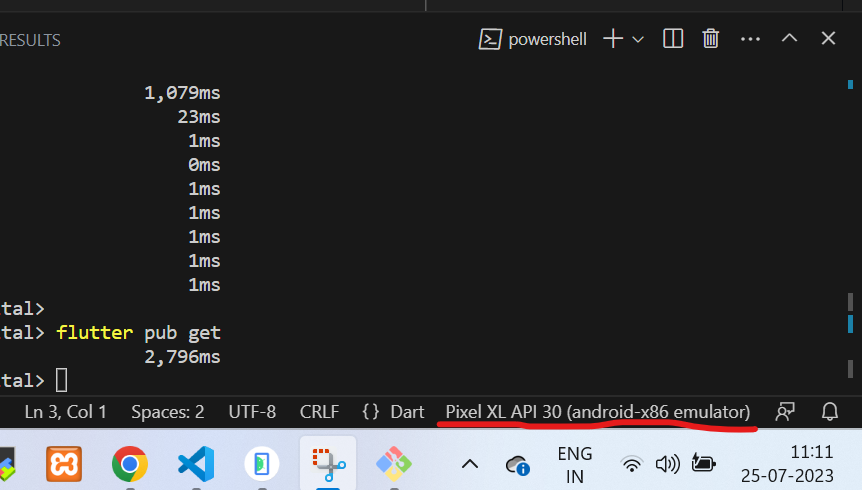
In your IDE, click on the “Run” button (usually a green triangle) to run the app.
flutter run
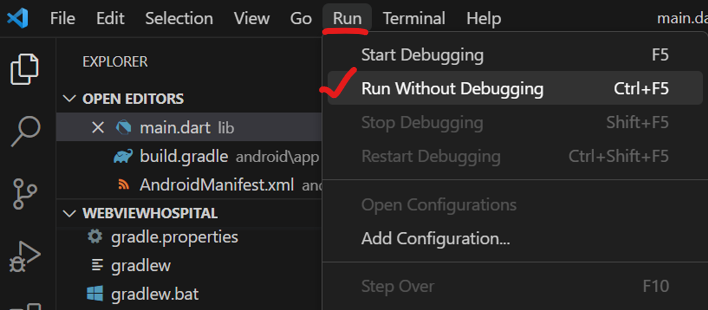
This will launch the app on the emulator or connected device, and you’ll see the “Hello World” message on the screen.
Open the main.dart
file located inside the lib
folder of your project. You will see the following code:
import 'package:flutter/material.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(
title: Text('Hello World'),
),
body: Center(
child: Text('Hello World!'),
),
),
);
}
}
The main
function is the entry point of the Flutter app, and it calls runApp()
to start the application. The MyApp
class is a StatelessWidget, which means its UI cannot change after it’s built.
The MaterialApp
widget sets up the basic material design for the app, and the Scaffold
widget provides a basic structure for the app, including an AppBar
and a body
.
The Text
widget inside the Center
widget displays the “Hello World!” message on the screen.
Now that you have successfully run your first Flutter app