Introduction
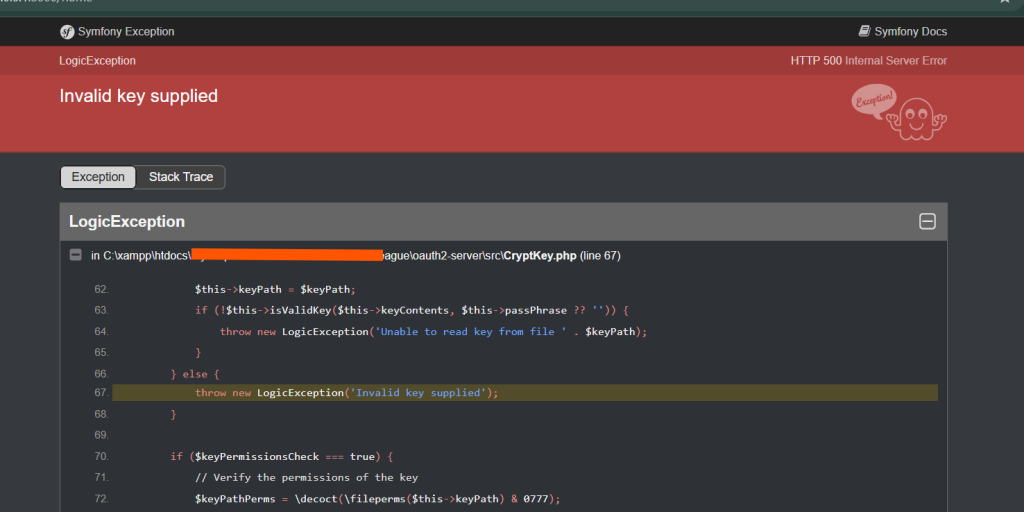
While working with Laravel Passport for authentication, you may encounter the following error:
local.ERROR: Invalid key supplied {"userId":1137,"exception":"[object] (LogicException(code: 0): Invalid key supplied at C:\\xampp\\htdocs\\
This error indicates that the OAuth key used for authentication is either missing or invalid. Below, we will discuss why this error occurs and how to resolve it.
Understanding the Error
In Laravel Passport, authentication relies on private and public keys stored in the storage/oauth-private.key
and storage/oauth-public.key
files. If these keys are missing, corrupted, or incorrectly set up, the application will throw the “Invalid key supplied” exception.
The error occurs in the CryptKey.php
file in the league/oauth2-server
package, which Laravel Passport depends on. Specifically, the exception is triggered when the system fails to validate the provided key:
throw new LogicException('Invalid key supplied');
Solution
1. Generate New Keys
To resolve this issue, regenerate the Passport keys by running the following command in your terminal:
php artisan passport:keys
This command will generate new private and public keys inside the storage/
directory, ensuring your authentication system works correctly.
2. Force Regeneration of Keys
If the above command doesn’t resolve the issue, you can force the regeneration of keys using:
php artisan passport:keys --force
This ensures that the existing keys are replaced with new ones.
3. Verify File Permissions
Ensure that the storage/oauth-private.key
and storage/oauth-public.key
files have the correct permissions. On Linux/macOS, you can set appropriate permissions using:
chmod 600 storage/oauth-private.key storage/oauth-public.key
4. Clear Configuration Cache
After regenerating the keys, clear Laravel’s configuration cache to ensure it loads the latest keys:
php artisan config:clear
php artisan cache:clear
5. Restart the Server
If you are using Laravel’s built-in server, restart it:
php artisan serve