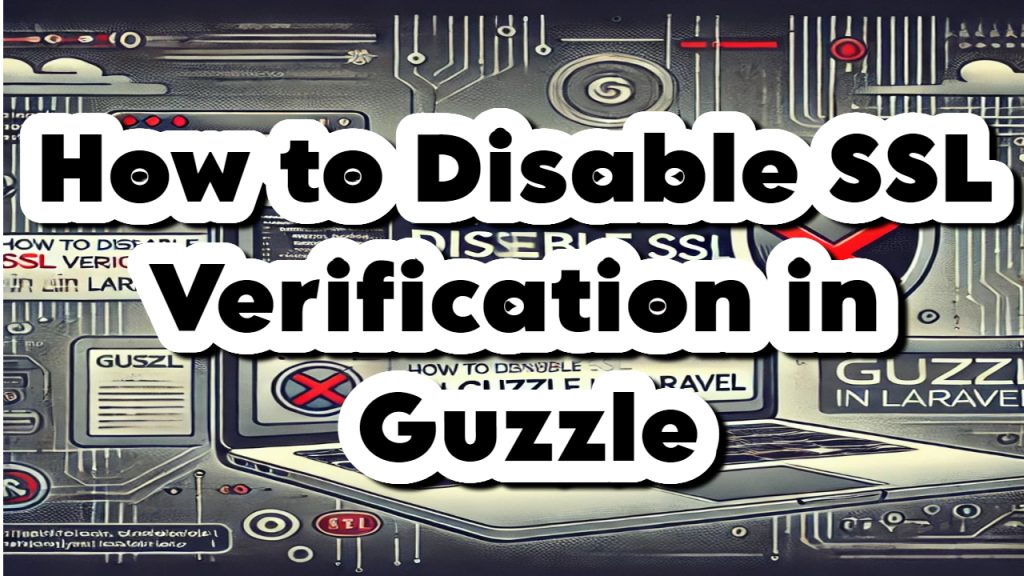
When working with Guzzle, a popular PHP HTTP client, you may encounter SSL certificate issues, especially in local development environments or when connecting to servers with self-signed certificates. A common error developers face is the cURL error 60: SSL certificate problem, which usually indicates that Guzzle couldn’t verify the SSL certificate of the server.
While it’s always recommended to resolve SSL issues properly by configuring certificates, sometimes you may need to disable SSL verification temporarily to continue development. In this blog, we’ll show you how to disable SSL verification in Guzzle when working with Laravel.
Why SSL Verification is Important
Before we dive into how to disable SSL verification, it’s essential to understand why SSL is important. SSL (Secure Sockets Layer) ensures that the data being transferred between your application and the server is encrypted, preventing it from being intercepted or tampered with.
Disabling SSL verification should only be done in specific cases, like during local development or testing with non-production servers, and never in a production environment.
Step-by-Step Guide to Disabling SSL Verification in Guzzle
1. Locate Your HTTP Request
If you’re using Guzzle in a Laravel project, it’s typically implemented in a service or controller. Locate the section of code where you’re making HTTP requests with Guzzle.
Here’s a simple example of using Guzzle to send a request:
use GuzzleHttp\Client;
$client = new Client();
$response = $client->request('GET', 'https://example.com/api/data');
2. Disable SSL Verification in Guzzle Request
To disable SSL verification, you need to pass an option called verify
in the request. Setting verify
to false
tells Guzzle to skip verifying the SSL certificate of the server.
Modify your Guzzle request like this:
use GuzzleHttp\Client;
$client = new Client();
$response = $client->request('GET', 'https://example.com/api/data', [
'verify' => false,
]);
3. Global Configuration in Laravel
If you’re making multiple Guzzle requests throughout your application and want to apply this configuration globally, you can do so in your service provider or by setting up a custom HTTP client.
You can modify the HttpClient
configuration in the app/Providers/AppServiceProvider.php
file.
Here’s an example of configuring a global Guzzle client:
use GuzzleHttp\Client;
use Illuminate\Support\ServiceProvider;
class AppServiceProvider extends ServiceProvider
{
public function register()
{
$this->app->singleton(Client::class, function () {
return new Client([
'verify' => false,
]);
});
}
public function boot()
{
//
}
}
By doing this, any time Guzzle is used in your application, SSL verification will be disabled unless you override it in specific requests.
4. Handling Guzzle in API Services
If you are using Guzzle in a dedicated API service class, you can disable SSL verification when initializing the client:
use GuzzleHttp\Client;
class ApiService
{
protected $client;
public function __construct()
{
$this->client = new Client([
'verify' => false, // Disable SSL verification
]);
}
public function fetchData()
{
$response = $this->client->request('GET', 'https://example.com/api/data');
return json_decode($response->getBody(), true);
}
}
Important Notes
- Use in Development Only: Disabling SSL verification is dangerous in a production environment, as it leaves your application vulnerable to man-in-the-middle attacks. Always ensure that SSL verification is enabled when working with live servers.
- Fix SSL Issues Properly: The right approach is to resolve SSL certificate issues rather than bypassing verification. This can be done by installing the correct certificates or updating the
cacert.pem
file used by Guzzle.