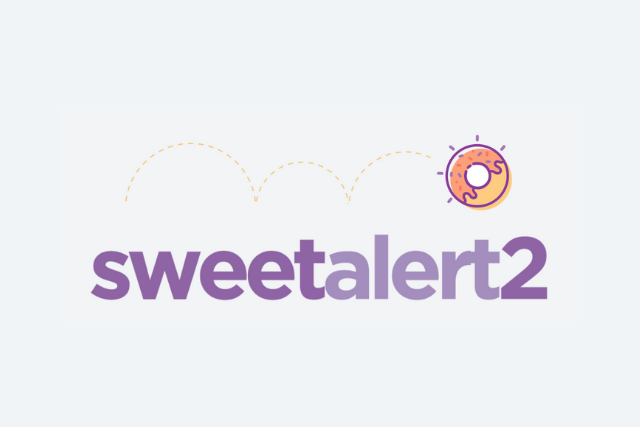
SweetAlert2 is a beautiful, responsive, and customizable replacement for JavaScript’s native alert, confirm, and prompt dialogs. It’s built to be flexible and easy to use, allowing developers to create visually appealing alert messages that seamlessly integrate into their web applications.
Why Choose SweetAlert2?
- Customization: SweetAlert2 provides extensive customization options, allowing developers to tailor alert messages to match their application’s design language perfectly.
- Responsive Design: The alerts generated by SweetAlert2 are responsive out of the box, ensuring a consistent user experience across devices and screen sizes.
- Ease of Use: Integrating SweetAlert2 into your project is straightforward, with clear and concise documentation available to guide you through the process.
- Animations: With built-in animations, SweetAlert2 adds flair to your alert messages, making them more engaging and visually appealing.
Integrating SweetAlert2 into your web application is a breeze. Here’s a quick guide to get you started:
- Installation: You can include SweetAlert2 in your project by adding the script tags directly to your HTML file or by installing it via package managers like npm or yarn.
<!-- Add this to your HTML file -->
<script src="https://cdn.jsdelivr.net/npm/sweetalert2@11"></script>
Basic Usage: Once included, you can use SweetAlert2 to display alerts, confirms, and prompts with just a few lines of code.
// Simple alert
Swal.fire('Hello, world!')
// Confirm dialog
Swal.fire({
title: 'Are you sure?',
text: "You won't be able to revert this!",
icon: 'warning',
showCancelButton: true,
confirmButtonColor: '#3085d6',
cancelButtonColor: '#d33',
confirmButtonText: 'Yes, delete it!'
}).then((result) => {
if (result.isConfirmed) {
Swal.fire(
'Deleted!',
'Your file has been deleted.',
'success'
)
}
})
Customization: SweetAlert2 allows you to customize every aspect of your alert messages, including titles, texts, icons, buttons, and animations. Refer to the documentation for a comprehensive list of customization options.
Example of how you can integrate SweetAlert2 into an HTML file to showcase its usage:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>SweetAlert2 Example</title>
<!-- Include SweetAlert2 CSS -->
<link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/sweetalert2@11/dist/sweetalert2.min.css">
</head>
<body>
<h1>SweetAlert2 Example</h1>
<button onclick="showAlert()">Show Alert</button>
<button onclick="showConfirm()">Show Confirm</button>
<!-- Include SweetAlert2 JS -->
<script src="https://cdn.jsdelivr.net/npm/sweetalert2@11"></script>
<script>
// Function to show a simple alert
function showAlert() {
Swal.fire('Hello, world!');
}
// Function to show a confirm dialog
function showConfirm() {
Swal.fire({
title: 'Are you sure?',
text: "You won't be able to revert this!",
icon: 'warning',
showCancelButton: true,
confirmButtonColor: '#3085d6',
cancelButtonColor: '#d33',
confirmButtonText: 'Yes, delete it!'
}).then((result) => {
if (result.isConfirmed) {
Swal.fire(
'Deleted!',
'Your file has been deleted.',
'success'
)
}
})
}
</script>
</body>
</html>
- We include the SweetAlert2 CSS file via a
<link>
tag in the<head>
section. - We include the SweetAlert2 JavaScript file via a
<script>
tag just before the closing</body>
tag. - Two buttons are provided to demonstrate the usage of SweetAlert2: one for showing a simple alert and another for showing a confirmation dialog.
- JavaScript functions
showAlert()
andshowConfirm()
are defined to trigger the respective SweetAlert2 messages.