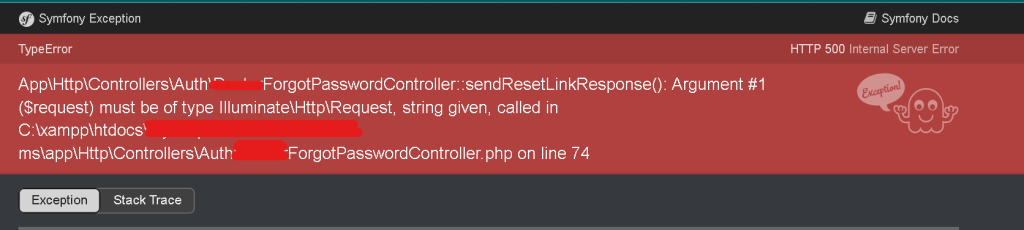
The error you’re encountering seems to stem from a method call within the sendResetLinkEmail()
function. Specifically, there appears to be an issue with the sendResetLinkResponse()
method call.
Identifying the Cause
- Method Invocation: The error likely occurs when calling the
sendResetLinkResponse()
method. - Incorrect Parameters: The parameters passed to the
sendResetLinkResponse()
method may not match its expected signature.
Error:
Error: public function sendResetLinkEmail(Request $request)
{
$input = $request->all();
$validator = Validator::make($request->only('email'), [
'email' => 'required|string|email|max:255|exists:users,email'
]);
if ($validator->fails()) {
return view('auth.passwords.email')->with(
[
'success' => false,
'data' => 'Validation Error.',
'message' => 'The selected email is invalid OR No admin approval to this account. Please check with admin to activate your account!'
]
);
} else {
$this->validateEmail($request);
Log::info('response 1');
// We will send the password reset link to this user. Once we have attempted
// to send the link, we will examine the response then see the message we
// need to show to the user. Finally, we'll send out a proper response.
$response = $this->broker()->sendResetLink(
$request->only('email')
);
Log::info('response');
return $response == Password::RESET_LINK_SENT
? $this->sendResetLinkResponse($response)
: $this->sendResetLinkFailedResponse($request, $response);
}
}
My error is in this line
? $this->sendResetLinkResponse($response)
: $this->sendResetLinkFailedResponse($request, $response);
Solution
To resolve this error, let’s ensure that the correct parameters are passed to the sendResetLinkResponse()
method. Here’s the modified code:
return $response == Password::RESET_LINK_SENT
? $this->sendResetLinkResponse($request, $response)
: $this->sendResetLinkFailedResponse($request, $response);
}
By ensuring that the correct parameters are passed to the sendResetLinkResponse()
method, you can resolve the error and ensure that password reset emails are sent successfully in your Laravel application.