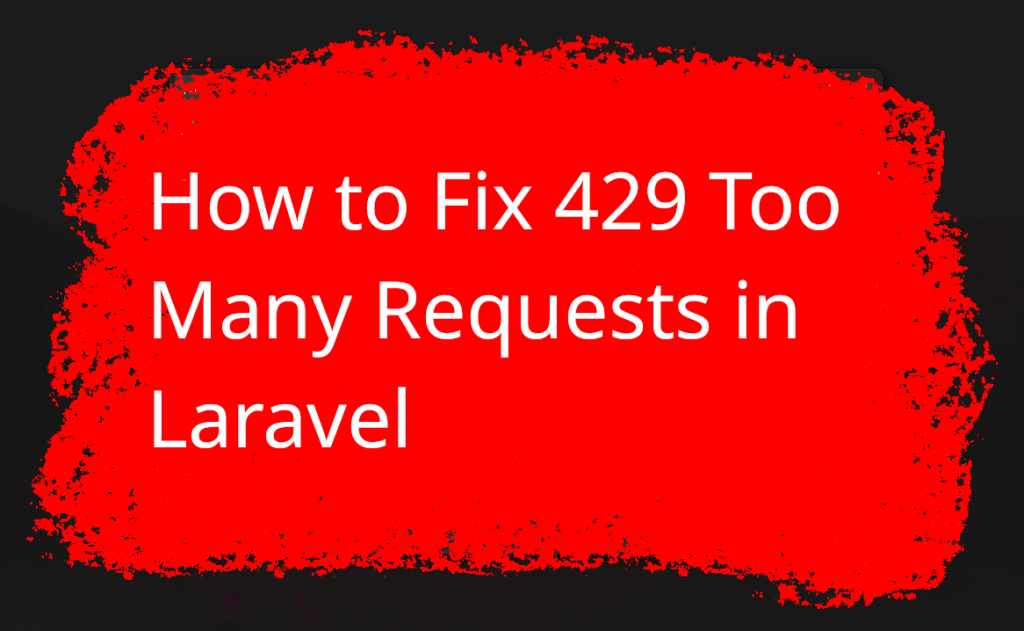
Encountering the “429 Too Many Requests” error in Laravel can be a challenging experience, but it’s not insurmountable. By understanding the underlying causes of the issue and taking proactive steps to adjust throttling parameters or remove unnecessary middleware, you can ensure that your application operates smoothly and efficiently. Remember to adhere to best practices for request throttling and handle errors gracefully to provide a seamless user experience.
When your Laravel application interacts with external APIs or handles a large number of incoming requests, it’s essential to manage the flow of traffic efficiently. The “429 Too Many Requests” error occurs when your application sends more requests to an API endpoint than the allowed limit within a specified period. This limit is typically enforced by the API provider to prevent abuse and ensure fair usage for all consumers.
Identifying the Middleware
In Laravel, request throttling is often implemented using middleware. If you navigate to your app/Http/Kernel.php
file, you’ll likely find a $middlewareGroups
variable that defines middleware for various HTTP request groups. Within the ‘api’ group, you’ll typically see the ‘throttle’ middleware:
protected $middlewareGroups = [
'api' => [
'throttle:60,1',
'bindings',
]
];
Resolving the Issue
To fix the “429 Too Many Requests” error, you have a few options:
Adjust Throttling Parameters: You can modify the throttle middleware parameters to adjust the request limits. The first parameter represents the maximum number of requests allowed per minute, while the second parameter specifies the number of minutes to wait before processing additional requests once the limit is exceeded.For example, to allow 100 requests per minute with a 5-minute wait time, you can modify the throttle middleware as follows:
'throttle:100,5'
Remove Throttling Middleware: If you find that throttling is unnecessary for your application or if you prefer to handle rate limiting differently, you can remove the throttle middleware from the ‘api’ group altogether:
'api' => [
// Remove 'throttle' middleware
'bindings',
]
Best Practices for Throttling
While adjusting throttle parameters can provide a quick fix, it’s essential to consider best practices for request throttling:
Understand API Limits: Familiarize yourself with the rate limits imposed by the API provider. Ensure that your application adheres to these limits to avoid disruptions in service.
Monitor and Adjust: Regularly monitor your application’s traffic patterns and adjust throttle parameters accordingly. Be proactive in responding to changes in usage patterns.
Implement Caching: Consider implementing caching mechanisms to reduce the number of requests hitting external APIs. Caching responses can help alleviate the burden on both your application and the API provider.
Manage Errors Smoothly: Set up error handling mechanisms to address 429 errors gracefully, offering informative responses to users. Consider incorporating retry logic or displaying user-friendly error messages when appropriate.
More topics on Bug fixing: