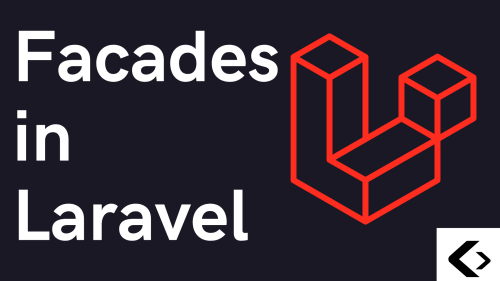
What are Facades in Laravel?
In Laravel, a facade is a design pattern used to provide a clean, static, and easy-to-use interface to the complex classes and services that power the framework. Facades serve as “static proxies” for underlying classes and components, making it a breeze to work with Laravel’s functionality.
Facades are an essential part of the service container, allowing you to access various services and components of the framework without the need to manually create instances of these classes. Laravel facades are a powerful feature that simplifies working with complex components and services in the framework. They provide a clean, consistent, and developer-friendly way to access various features like the database, cache, and authentication. By reducing boilerplate code and improving testability, facades contribute to more readable, maintainable, and efficient code. When developing applications with Laravel, leveraging facades can significantly enhance your development experience and productivity.
Uses of Facades in Laravel
Facades in Laravel serve several important purposes:
1. Simplified Access to Services
Facades provide a simple and expressive way to access Laravel services such as the database, cache, session management, authentication, and more. Instead of dealing with the complexity of these services directly, you can use facades to interact with them in a more intuitive and consistent manner.
2. Improved Testability
Using facades makes it easier to write unit tests for your code. Since facades allow you to interact with services in an isolated manner, you can easily replace real services with mock objects or test doubles during testing. This enhances your application’s testability and helps you identify and fix issues more quickly.
3. Reduced Boilerplate Code
Facades eliminate the need for manually instantiating and configuring objects. They provide a concise way to access complex functionality, reducing the amount of boilerplate code in your application. This results in cleaner, more maintainable code.
4. Consistency
Facades offer a unified and consistent API for interacting with various Laravel services. Developers don’t need to learn a new set of methods or configuration for each service. This consistency makes the framework more user-friendly and reduces the learning curve for developers.
Advantages of Facades
The advantages of using facades in Laravel are numerous and include:
1. Readability
Facades improve the readability of your code by providing a clean and expressive syntax for interacting with complex services. Your code becomes more self-explanatory, making it easier to understand for you and your fellow developers.
2. Maintainability
By reducing boilerplate code and providing a consistent interface to various services, facades contribute to better code maintainability. This means you can spend less time on maintenance and more time on developing new features.
3. Testability
Facades enhance your application’s testability, enabling you to write comprehensive unit tests for your code. You can easily isolate and test specific components without worrying about the intricacies of the underlying services.
4. Developer-Friendly
Laravel’s facades are designed with the developer’s convenience in mind. They simplify the process of working with the framework, allowing developers to be more productive and focused on building features rather than dealing with framework intricacies.
How to Use Facades in Laravel
Now that we’ve discussed what facades are and their advantages, let’s look at how to use them in Laravel. We’ll cover a common use case involving database access and demonstrate the use of both the DB
facade and Eloquent, Laravel’s ORM.
Using the DB Facade
Suppose you want to retrieve data from a database table using the DB
facade. Here’s how you can do it:
use Illuminate\Support\Facades\DB;
// Retrieve data from the "users" table using the DB facade
$users = DB::table('users')->get();
In the code above, we first import the DB
facade. Then, we use the table
method to specify the “users” table and the get
method to fetch the data. This provides a clean and straightforward way to interact with the database without needing to handle database connections and queries manually.
Using Eloquent with Facades
Laravel’s Eloquent ORM can also be used with facades. Here’s an example of how to retrieve a user from the “users” table using the User
facade:
use Illuminate\Support\Facades\User;
$user = User::find(1);
In this code, we import the User
facade and use the find
method to fetch a user by their ID. This demonstrates how you can work with Eloquent models, which are a powerful and expressive way to interact with your database.