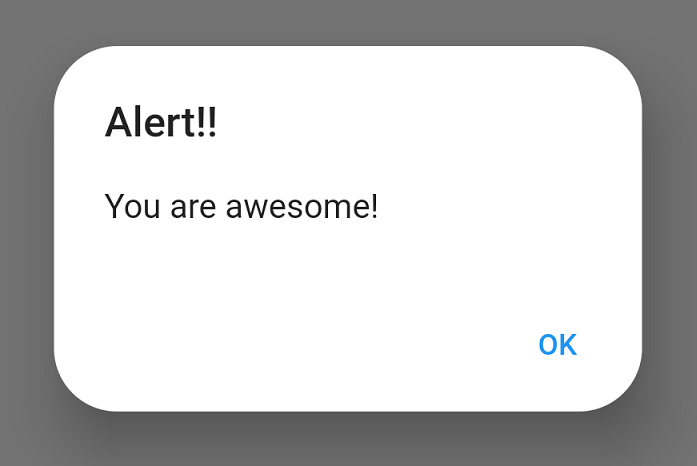
Alert dialog boxes are a common way to display important information to users in mobile apps. Flutter provides a simple and easy way to create alert dialog boxes.
Creating an alert dialog box
To create an alert dialog box in Flutter, you can use the AlertDialog
widget. The AlertDialog
widget takes a number of parameters, including:
title
: The title of the alert dialog box.content
: The content of the alert dialog box.actions
: A list of buttons that can be displayed at the bottom of the alert dialog box.
Example
The following code shows how to create a simple alert dialog box:
import 'package:flutter/material.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
body: Center(
child: TextButton(
onPressed: () {
showDialog(
context: context,
builder: (context) => AlertDialog(
title: Text('Alert Dialog Title'),
content: Text('Alert Dialog Content'),
actions: [
TextButton(
onPressed: () {
Navigator.of(context).pop();
},
child: Text('Close'),
),
],
),
);
},
child: Text('Show Alert Dialog'),
),
),
),
);
}
}
When the user taps the “Show Alert Dialog” button, an alert dialog box will be displayed with the title “Alert Dialog Title” and the content “Alert Dialog Content”. The alert dialog box will also have a single close button.
Customizing an alert dialog box
You can customize the appearance and behavior of an alert dialog box by passing additional parameters to the AlertDialog
widget. For example, you can:
- Set the background color of the alert dialog box.
- Set the elevation of the alert dialog box.
- Set the shape of the alert dialog box.
- Add additional buttons to the alert dialog box.
Example
The following code shows how to customize an alert dialog box:
import 'package:flutter/material.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
body: Center(
child: TextButton(
onPressed: () {
showDialog(
context: context,
builder: (context) => AlertDialog(
title: Text('Alert Dialog Title'),
content: Text('Alert Dialog Content'),
backgroundColor: Colors.blue,
elevation: 20,
shape: RoundedRectangleBorder(
borderRadius: BorderRadius.circular(10),
),
actions: [
TextButton(
onPressed: () {
Navigator.of(context).pop();
},
child: Text('Close'),
),
TextButton(
onPressed: () {
// Do something else
},
child: Text('Cancel'),
),
],
),
);
},
child: Text('Show Alert Dialog'),
),
),
),
);
}
}
This code will create an alert dialog box with a blue background, a 20 pixel elevation, and rounded corners. The alert dialog box will also have two buttons: a close button and a cancel button.