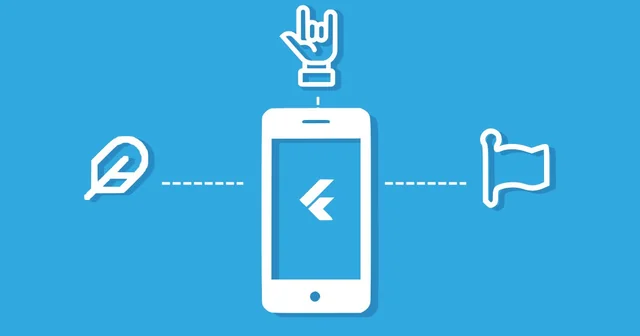
Create a folder for your custom icons in your Flutter project directory. For example, you could create a folder called assets/icons
.
Add your custom icons to the folder. Your icons can be in PNG, SVG, or TTF format.
Add the following code to your pubspec.yaml
file:
flutter:
uses-material-design: true
assets:
- assets/icons/
Import the custom icons into your Dart code. For example, if you have a custom icon called my_icon.png
, you can import it as follows:
import 'package:flutter/material.dart';
class MyClass extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Image.asset('assets/icons/my_icon.png');
}
}
Use the custom icons in your Flutter app like any other widget. For example, you can use the Image.asset()
widget to display a custom icon, or you can use the Icon()
widget to display a custom icon as a vector.
Example
The following example shows how to use a custom icon in a Flutter app:
import 'package:flutter/material.dart';
class MyClass extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('My App'),
leading: Icon(CustomIcons.my_icon),
),
body: Center(
child: Image.asset('assets/icons/my_icon.png'),
),
);
}
}
class CustomIcons {
static const IconData my_icon = IconData(0xe900);
}
This example will create a Flutter app with a custom icon in the app bar and in the body of the app.
Generating custom icons
If you don’t have any custom icons, you can use a variety of online tools to generate them. One popular tool is FlutterIcon.com. To use FlutterIcon.com, simply select the icons you want to use and click the “Download” button. FlutterIcon.com will generate a TTF file and a Dart file for your custom icons.