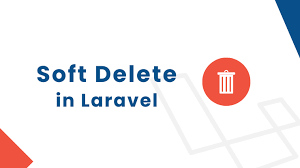
Soft deleting is a Laravel feature that allows you to hide records from your application without permanently deleting them from the database. This can be useful for a variety of reasons,
such as:
- Keeping a record of deleted records for audit purposes
- Giving users the ability to restore deleted records
- Avoiding database fragmentation
To use soft deleting in Laravel, you need to:
- Add a
deleted_at
column to your database table. This column should be of typedatetime
and nullable. - Add the
SoftDeletes
trait to your model. - Call the
delete()
method on the model to soft delete it.
Soft deleting is a crucial feature in Laravel, a popular PHP framework, that allows you to “delete” records from a database while preserving their existence in the database. This feature is a powerful tool for protecting your data integrity and complying with legal or regulatory requirements. It is removed from the database permanently. Soft deleting, however, doesn’t actually erase the data but instead marks it as “deleted.” This way, the information remains in the database but is no longer visible in standard queries. Soft deleting is especially useful when you need to maintain a historical record of data changes, comply with data retention policies, or recover accidentally deleted data.
Step 1: Database Migration
In your database migration file, add a deleted_at
column of type timestamp
to the table you want to enable soft deleting for. This column will store the deletion timestamp for each record.
Schema::create('your_table', function (Blueprint $table) {
// ... other columns
$table->softDeletes(); // Adds the 'deleted_at' column
});
Step 2: Model Configuration
In your model, use the SoftDeletes
trait provided by Laravel. This trait adds the necessary methods and properties to the model.
use Illuminate\Database\Eloquent\Model;
use Illuminate\Database\Eloquent\SoftDeletes;
class YourModel extends Model
{
use SoftDeletes;
// ...
}
That’s it! Your Laravel model is now set up for soft deleting.
Performing Soft Deletes
To soft delete a record, use the delete
method on your model instance. Laravel will automatically set the deleted_at
column to the current timestamp without physically removing the record from the database.
$record = YourModel::find($id);
$record->delete();
Restoring Soft Deleted Records
Soft deleted records can be restored easily. Use the restore
method on a model instance to bring the record back to life.
$record = YourModel::withTrashed()->find($id); // Find the soft deleted record
$record->restore(); // Restore the record
Permanently Deleting Records
In some cases, you may want to remove soft-deleted records permanently from the database. To do this, use the forceDelete
method.
$record = YourModel::withTrashed()->find($id); // Find the soft deleted record
$record->forceDelete(); // Permanently delete the record
Querying Soft Deleted Records
To retrieve soft deleted records alongside active ones, use the withTrashed
method in your query:
$records = YourModel::withTrashed()->get();
To retrieve only soft deleted records, you can use the onlyTrashed
method:
$records = YourModel::onlyTrashed()->get();