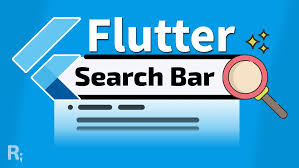
Filtering and searching are essential functionalities in many mobile apps. we’ll explore how to implement a search or filter feature in a Flutter app.
Let’s create a new Flutter project to implement the search or filter feature. Open your terminal and run the following commands:
flutter create search_filter_app
cd search_filter_app
This will create a new Flutter project named “search_filter_app” and change your working directory to the project folder.
Creating the User Interface
To keep things simple, we will create a list of items with a search bar at the top. Follow these steps to create the user interface:
- Open the
lib/main.dart
file. - Replace the contents with the following code:
import 'package:flutter/material.dart';
void main() {
runApp(SearchFilterApp());
}
class SearchFilterApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Search and Filter',
home: SearchFilterScreen(),
);
}
}
class SearchFilterScreen extends StatefulWidget {
@override
_SearchFilterScreenState createState() => _SearchFilterScreenState();
}
class _SearchFilterScreenState extends State<SearchFilterScreen> {
final List<String> items = List.generate(50, (index) => 'Item $index');
List<String> filteredItems = [];
void filterItems(String query) {
filteredItems = items
.where((item) => item.toLowerCase().contains(query.toLowerCase()))
.toList();
setState(() {});
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('Search and Filter'),
),
body: Column(
children: <Widget>[
Padding(
padding: const EdgeInsets.all(16.0),
child: TextField(
onChanged: filterItems,
decoration: InputDecoration(
labelText: 'Search',
border: OutlineInputBorder(
borderRadius: BorderRadius.circular(12),
),
),
),
),
Expanded(
child: ListView.builder(
itemCount: filteredItems.isNotEmpty ? filteredItems.length : items.length,
itemBuilder: (context, index) {
return ListTile(
title: Text(filteredItems.isNotEmpty ? filteredItems[index] : items[index]),
);
},
),
),
],
),
);
}
}
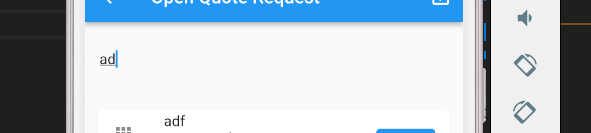
In this code, we create a simple Flutter app with a search bar and a list of items. The filterItems
method is used to filter the items based on the user’s input. The filtered items are displayed in a ListView
.
Filtering or Searching Data
In the code above, the filterItems
method is responsible for filtering the items based on the search query. It uses the where
method to filter the items and converts them into a list. The setState
method is called to trigger a rebuild of the UI with the updated filtered items.
Displaying Filtered Results
The filtered results are displayed in a ListView.builder
. The itemCount
property is set based on whether there are filtered items. If there are filtered items, the count is set to the number of filtered items; otherwise, it’s set to the total number of items.
The filtered items or the original items are displayed as ListTile
widgets in the list.
Styling the Search Bar
In the code, we’ve styled the search bar by providing it with rounded borders. We set the borderRadius
property to achieve the rounded appearance. Adjust the borderRadius
value to control the roundness of the corners.
Testing and Running the App
To test the app, open your terminal and navigate to your project directory. Then, run the app with the following command:
flutter run