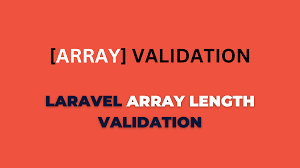
Laravel, one of the most popular PHP frameworks, empowers developers with a wide array of tools and features for efficient web application development. In this guide, we will explore Laravel’s array length validation, a crucial aspect when dealing with arrays in your application. We’ll delve into the Laravel validation rules that allow you to specify the minimum, maximum, or a range of lengths for an array.
Arrays play a significant role in web development, and Laravel provides built-in validation rules to ensure the integrity of array data. You can use these rules to enforce specific constraints on array length, ensuring that your application’s data meets your requirements.
Let’s explore three essential array length validation rules in Laravel:
1. Laravel Validation Array Min:
When you need to validate that an array contains a minimum number of elements, you can use the min
rule. For instance, if you want to ensure that an array named ‘users’ contains at least three elements, you can implement the validation as follows.
'users' => 'array|min:3'
2. Laravel Validation Array Max:
Conversely, when you want to validate that an array does not exceed a maximum number of elements, you can employ the max
rule. For instance, to ensure that the ‘users’ array contains no more than three elements, use the validation rule like this.
'users' => 'array|max:3'
3. Laravel Validation Array Between:
Sometimes, you may require an array to have a length within a specific range. Laravel’s between
rule is the perfect tool for this job. To validate that the ‘users’ array contains at least three but no more than ten elements, you can utilize the between
rule like so.
'users' => 'array|between:3,10'
Putting it into Practice: Controller Code Example
Now that we’ve covered the array length validation rules let’s take a look at a practical example using a Laravel controller. In this example, we have a ‘FormController’ that handles user input validation.
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
use App\Models\User;
use Illuminate\View\View;
use Illuminate\Http\RedirectResponse;
class FormController extends Controller
{
public function create(): View
{
return view('createUser');
}
public function store(Request $request): RedirectResponse
{
$request->validate([
'users' => 'array|between:3,10'
]);
// Additional processing and storage logic can be added here.
return back()->with('success', 'User created successfully.');
}
}
[…] laravel-array-length-validation […]