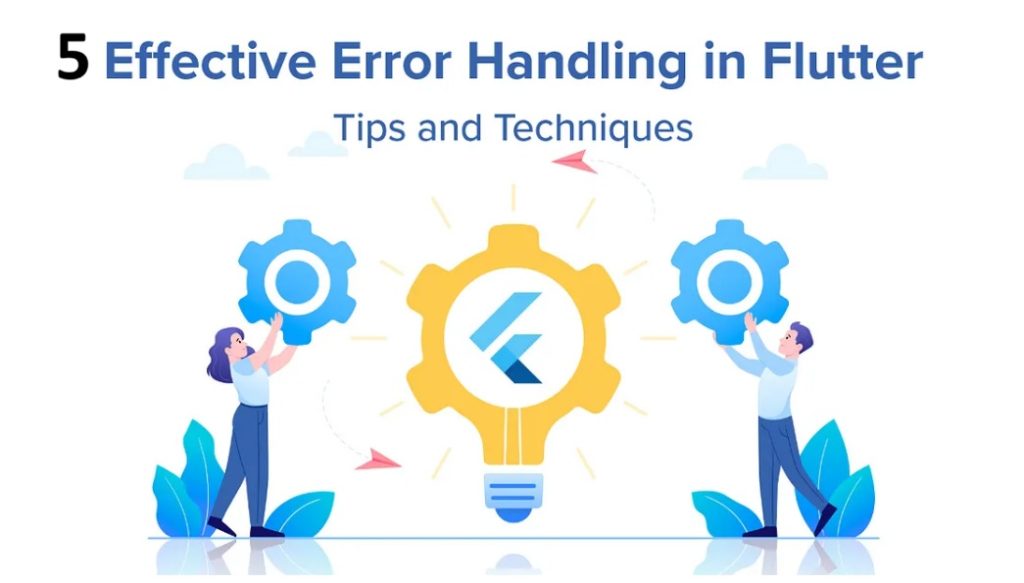
As a Flutter developer, you’re likely familiar with the exhilaration of building sleek, cross-platform mobile apps. However, alongside the excitement comes the challenge of handling errors effectively. Flutter errors can derail your app’s performance and user experience if not managed properly. In this article, we’ll explore five common Flutter errors, delve into their causes and symptoms, and equip you with practical strategies to tackle them like a pro.
Introduction
Imagine this: you’ve just updated your ride-sharing app’s UI to impress your users with a modern look. Excitedly, you launch the app, only to be greeted by a dreaded error message. This scenario is all too familiar for Flutter developers. Errors like these can range from NullPointerExceptions to FormatExceptions, each posing unique challenges that demand swift resolution.
Key Takeaways
Before we dive into the technical details, it’s essential to grasp a few key takeaways:
Error Variety: Flutter errors span a spectrum, including NullPointerExceptions, TypeErrors, and more.
Causes and Symptoms: Understanding what triggers these errors and their telltale signs is crucial for effective resolution.
Best Practices: Implementing robust error handling practices like logging, graceful error messaging, and strategic debugging can prevent and mitigate these issues.
Flutter NullPointerException
Cause: Occurs when attempting to access methods or properties of a null object.
Symptoms: App crashes or unexpected behavior due to uninitialized variables or improper null checks.
Resolution Strategies:
Null Checks: Always validate variables before accessing them to prevent NullPointerExceptions.
Conditional Statements: Use if statements or conditional expressions to handle potential null references.
Try-Catch Blocks: Wrap risky operations in try-catch blocks to catch exceptions gracefully.
Example Code:
String? name; // Nullable string
if (name != null) {
print(name.length); // Safe to access length if name is not null
} else {
print('Name is null'); // Handle null case
}
Flutter IndexError
Cause: Occurs when trying to access an index outside the valid range of a list or array.
Symptoms: App crashes or unexpected behavior when manipulating lists or arrays.
Resolution Strategies:
Index Range Checks: Always validate index ranges before accessing list elements.
Debugging Tools: Utilize Flutter’s debugging tools to pinpoint the source of index errors.
Edge Case Handling: Implement checks for empty lists or specific index bounds.
Example Code:
List<int> numbers = [1, 2, 3];
if (index >= 0 && index < numbers.length) {
print(numbers[index]); // Accessing element safely within bounds
} else {
print('Index out of bounds'); // Handle out-of-bound scenario
}
Flutter TypeError
Cause: Occurs when there is a mismatch between expected and actual types of variables or expressions.
Symptoms: Unexpected behavior or crashes due to type inconsistencies.
Resolution Strategies:
Type Validation: Validate variable types before performing operations to ensure compatibility.
Data Conversion: Use appropriate methods for type conversions to avoid type-related errors.
Testing: Conduct thorough unit tests to catch type-related issues early in development.
Example Code:
int number = '123'; // Incorrect assignment leading to TypeError
if (number is int) {
print('Number is an integer');
} else {
print('Number is not an integer'); // Handle type mismatch
}
Flutter FormatException
Cause: Arises from issues with data formatting or parsing, such as incorrect input formats or invalid conversions.
Symptoms: Errors triggered during data processing due to format inconsistencies.
Resolution Strategies:
Data Validation: Implement checks to ensure input data adheres to expected formats using regex or custom validators.
Exception Handling: Wrap parsing operations in try-catch blocks to handle format errors gracefully.
Data Conversion: Use reliable methods for converting data between formats to prevent format-related issues.
Example Code:
String text = '123abc';
try {
int number = int.parse(text); // Attempting to parse non-numeric string
print('Parsed number: $number');
} catch (e) {
print('Invalid format: $e'); // Handle format exception
}
Flutter ArgumentError
Cause: Occurs when incorrect arguments are passed to a function or method.
Symptoms: Unexpected behaviors or crashes due to improper argument handling.
Resolution Strategies:
Argument Validation: Validate arguments before passing them to functions to ensure they meet expected criteria.
Error Handling: Use try-catch blocks to catch and handle ArgumentError instances gracefully.
Refactoring: Improve code structure to clarify argument expectations and reduce potential errors.
Example Code:
void greet(String name, {int age}) {
if (age != null && age >= 18) {
print('Hello $name');
} else {
throw ArgumentError('Invalid age');
}
}
try {
greet('Alice', age: 20); // Valid call
} catch (e) {
print('Error: $e'); // Handle argument error
}