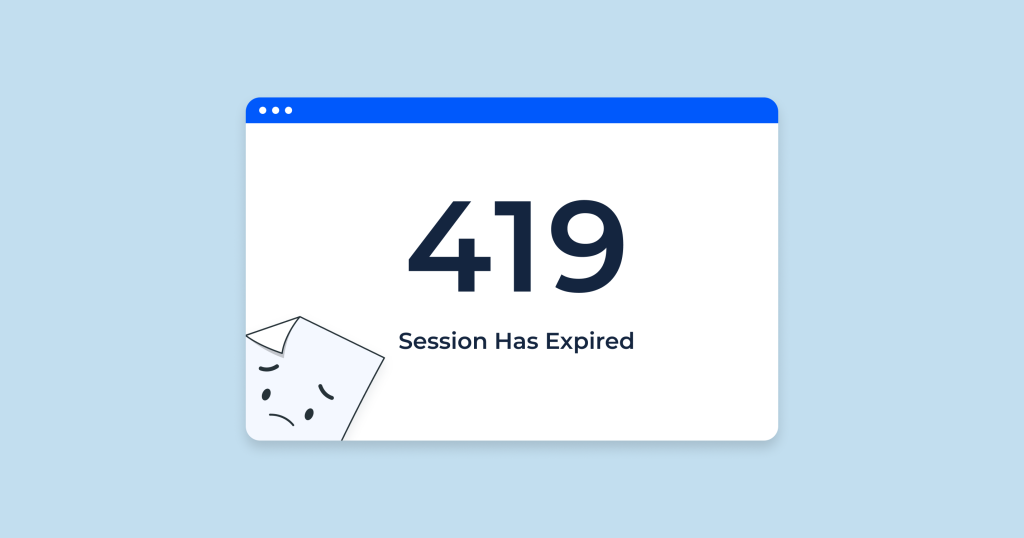
Understanding the 419 Error/Page Expired
The 419 Error/Page Expired is a web error that occurs when a user interacts with a web form, such as a login or a contact form, and the server detects a missing CSRF token. CSRF tokens are a security measure used to prevent Cross-Site Request Forgery attacks. When a form is submitted without a valid CSRF token, the server considers the request expired and returns the 419 Error. In the ever-evolving landscape of web development, security plays a pivotal role in safeguarding sensitive data and ensuring the integrity of web applications. One of the security features that you might encounter while working with web applications is the 419 Error or Page Expired. This error often stems from a crucial security measure known as Cross-Site Request Forgery (CSRF) protection.
What is CSRF and Why is it Important?
Cross-Site Request Forgery (CSRF) is a type of security vulnerability that allows malicious actors to trick users into unknowingly performing actions on a web application without their consent. This can lead to unauthorized actions being taken on behalf of the user, potentially compromising their data and account.
To mitigate this threat, web applications implement CSRF protection by requiring a CSRF token with each form submission. The token acts as a unique and secret identifier, ensuring that the request is coming from a legitimate source. If the token is missing or incorrect, the server will respond with the 419 Error to prevent the potentially malicious request.
Excluding Routes from CSRF Protection
In some cases, you may encounter scenarios where you want to allow certain routes to function without the need for a CSRF token. Laravel, a popular PHP framework, offers a simple way to accomplish this. By modifying the VerifyCsrfToken
middleware, you can specify which routes or URIs should be excluded from CSRF verification.
Here’s an example of how you can exclude routes in Laravel:
<?php
namespace App\Http\Middleware;
use Illuminate\Foundation\Http\Middleware\VerifyCsrfToken as Middleware;
class VerifyCsrfToken extends Middleware
{
/**
* The URIs that should be excluded from CSRF verification.
*
* @var array
*/
protected $except = [
'stripe/*',
'http://example.com/foo/bar',
'http://example.com/foo/*',
];
}
In example, the $except
array lists the URIs that should be exempt from CSRF verification. This can be useful for APIs or specific endpoints that don’t require CSRF protection.